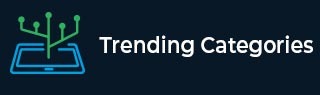
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming
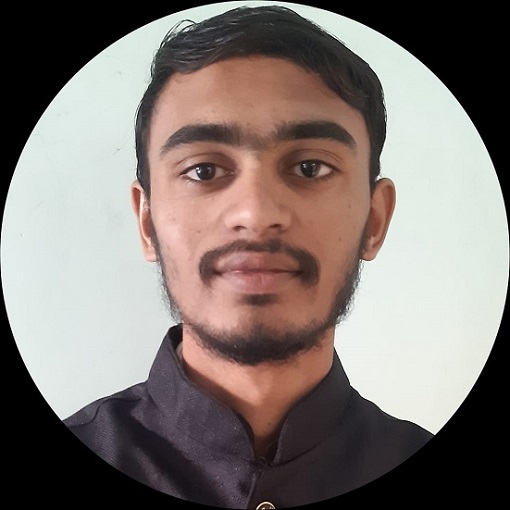
88 Views
In this problem, we will find the largest multiple of 3 using the array elements. The naïve approach is that generate all possible combinations of array elements and check whether it is divisible by 3. In this way, keep track of the maximum possible multiple of 3. The efficient approach is using three queues. We can use queues to store elements based on the remainder after dividing it into 3. After that, we can remove some elements from the queue to make a multiple of 3 from the remaining array elements. Problem statement − We have given an array ... Read More

151 Views
An octal number is a number expressed in the base-8 numeral system. It uses digits from 0 to 7. Octal numbers are commonly used in computer science and digital systems, especially when dealing with groups of three bits. In octal notation, each digit represents an increasing power of 8. The rightmost digit represents 8^0 (1), the next digit represents 8^1 (8), the next digit represents 8^2 (64), and so on. By combining these digits, octal numbers can represent positive integers. For example, the octal number 52 represents the decimal number as follows. (5 * 8^1) + (2 * 8^0) = ... Read More
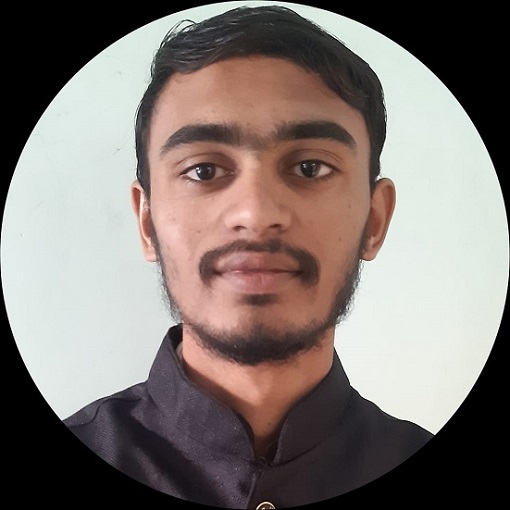
134 Views
In this problem, we will find the K nearest point from the origin in the 2D plane from the given N points. We can use the standard Euclidean distance formula to calculate the distance between the origin and each given point. After that, we can store the points with distance in the array, sort the array according to the distance, and take the first K points. However, we can also use the priority queue to store the 2D points according to their distance from the origin. After that, we can deque the queue for K times. Problem statement − ... Read More
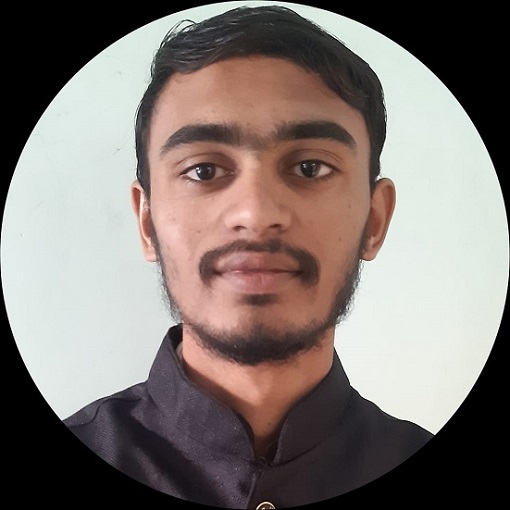
41 Views
In this problem, we will convert the number N to M by adding the one prime factor of N to itself and updating it in each operation. We will use the breadth−first search algorithm to solve the problem. We will find the prime factor of each updated N and insert it into the queue after adding it to the prime factor of N. Also, we will define functions to find the smallest prime factor of a particular number. Problem statement − We have given N and M integer values. We need to count the minimum number of operations required to ... Read More

99 Views
Python socket is a set of modules used for socket programming, which enables communication between processes over IP networks. Sockets in Python provide the backbone for most network programming tasks. By importing relevant modules, we can write Python programs to create client and server programs for different types of network applications such as web scraping, file transfer, email clients, and chat applications. The "socket" module is the most important module of socket programming in Python. It provides different types of connection-oriented and connection-less sockets that implement specific protocol levels such as TCP, UDP, etc. TCP (Transmission Control Protocol) sockets TCP ... Read More
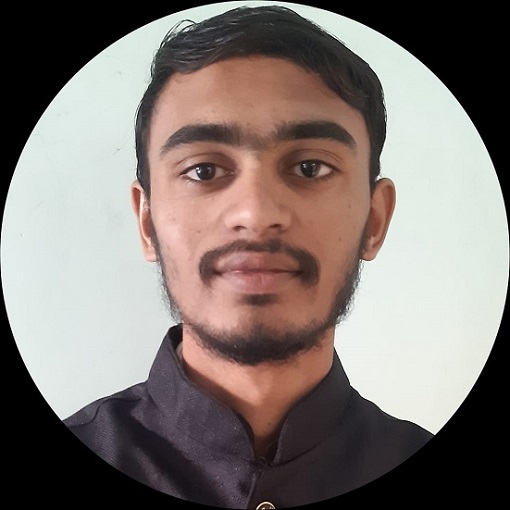
49 Views
In this problem, we will count the number of elements removed from the array before they are inserted in the array. The logical part for solving the problem is that check for all numbers that its position in the remove[] array before its position in the insert[] array. If yes, we can count that particular element of the remove[] array in the answer. However, we will use the map data structure to improve the performance of the code. Problem statement − We have given an insert[] and remove[] arrays containing first N integers. The insert[] array represents the order of ... Read More

55 Views
A Dictionary is an unordered collection of data structure which stores the elements as key and value. It is mutable and in other programming languages Dictionaries are also known as associative arrays, hash maps, or hash tables. The key is the unique one and the values are of a single element or a list of elements with duplicates. A Dictionary can be created by using the curly braces {} or by using the built in function dict(). Let’s see an example of creating the dictionary in python. Example student = { 'name': 'Tutorialspoint', 'age': ... Read More
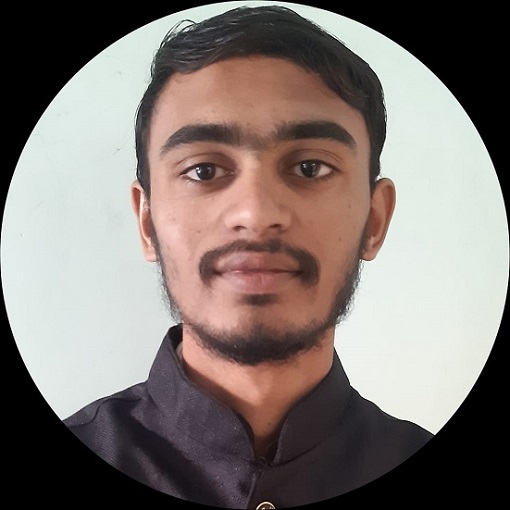
404 Views
In this problem, we will convert the linked list to the complete binary tree. We can assume the linked list as an array. The pth element of the linked list is the parent node of the binary tree's 2*p + 1 and 2*p + 2 elements. So, we can traverse through each element of the linked list and construct the binary tree. Problem statement − We have given a linked list containing N nodes. We need to construct the complete binary tree from the given linked list. Also, print the in−order traversal of the binary tree. Note − In the ... Read More

2K+ Views
Progress bars in Python are visual indicators that provide feedback on the progress of a task or operation. They are useful for long-running processes or iterations where it's helpful to show how much work has been completed and how much is remaining. A progress bar typically consists of a visual representation, such as a horizontal bar or a textual representation, which dynamically updates to reflect the progress of the task. It also includes additional information like the percentage of completion, estimated time remaining, and any relevant messages or labels. The following are the purposes served by the progress bars in ... Read More

69 Views
What is PHP? PHP (Hypertext Preprocessor) is a popular scripting language designed for web development. It is widely used for creating dynamic and interactive web pages. PHP code can be embedded directly into HTML, allowing developers to mix PHP and HTML seamlessly. PHP can connect to databases, process form data, generate dynamic content, handle file uploads, interact with servers, and perform various server-side tasks. It supports a wide range of web development frameworks, such as Laravel, Symfony, and CodeIgniter, which provide additional tools and features for building web applications. PHP is an open-source language with a large community, extensive documentation, ... Read More