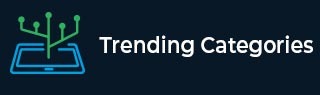
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

280 Views
Image classification is a type of machine learning task that involves identifying the objects or scenes in an image. It is a challenging task, but it has many applications in real−world problems such as facial recognition, object detection, and medical image analysis. In this article, we will discuss how to classify clothing images using Python. We will use the Fashion−MNIST dataset, which is a collection of 60, 000 grayscale images of 10 different clothing items. We will build a simple neural network model to classify these images. Import the Modules The first step is to import the necessary modules. We ... Read More

1K+ Views
Excel uses a special format to store dates and times, called serial date numbers. Serial date numbers are a count of days since January 1, 1899, which is the date that Excel considers to be the beginning of time. Python's datetime module provides powerful tools for working with dates and times. However, when it comes to interoperability with other applications, such as Microsoft Excel, we often encounter the need to convert Python datetime objects into Excel's serial date number format. In this article, we will explore how to perform this conversion and bridge the gap between Python and Excel. Understanding ... Read More

114 Views
Arrays are fundamental data structures in programming, enabling us to store and manipulate collections of values efficiently. Python, as a versatile programming language, provides numerous tools and libraries for working with arrays and matrices. In particular, the ability to convert 1−D arrays into 2−D arrays is an essential skill when dealing with tabular data or performing operations requiring a two−dimensional structure. In this article, we will explore the process of converting 1−D arrays into columns of a 2−D array using Python. We will cover various methods, ranging from manual manipulation to leveraging powerful libraries such as NumPy. Whether you are ... Read More

129 Views
In this Go language article, we will write programs that take in list of weights and values for items and a maximum weight capacity for knapsack. Knapsack problem is an optimization problem that uses dynamic programming. Here, the purpose is to find out the set of items that can be included in the knapsack without exceeding its weight capacity or maximum weight. Dynamic programming involves solving of problems by breaking them down into smaller subproblems and them combining them to get an optimal solution. Syntax func make ([] type, size, capacity) The make function in go language ... Read More

135 Views
In this Go language article, we will write programs that take in a slice of integers and an anonymous function that filters each element in the slice. An anonymous function is the function which uses no function name and is called by the variable which is assigned to it. It is used generally used with event listeners. Here, Filter function will be created with the anonymous functions to filter the values from slice. Syntax func append(slice, element_1, element_2…, element_N) []T The append function is used to add values to an array slice. It takes number of arguments. The first ... Read More

62 Views
In this article, we will write Go language programs that take in a slice of integers and an anonymous function that maps each element in the slice to a new value. An anonymous function is declared without a name and is assigned to a variable which is called to execute the process. Syntax func make ([] type, size, capacity) The make function in go language is used to create an array/map it accepts the type of variable to be created, its size and capacity as arguments. func range(variable) The range function is used to iterate over any data ... Read More

454 Views
In this article, we will write a Go language program to print the numbers from 1 to 100 concurrently with each number printed by a separate go routine. Concurrency means execution of multiple tasks parallelly and independently and making sure all the resources of the system are utilized. It can be achieved via go routines which are light weight threads and the channels. Syntax func make ([] type, size, capacity) The make function in go language is used to create an array/map it accepts the type of variable to be created, its size and capacity as arguments. Algorithm ... Read More

653 Views
In this Go language article, we will write programs to take in a slice of integers and compute their sum using concurrency. Concurrency helps multiple tasks or operations to be performed simultaneously. It helps make efficient use of system resources. It is achieved using Go routines which are light-weight threads and channels which helps in the communication between the go routines. Syntax func make ([] type, size, capacity) The make function in go language is used to create an array/map it accepts the type of variable to be created, its size and capacity as arguments. func range(variable) The ... Read More

156 Views
In this article, we will write Go language programs to sort a slice of integers using merge sort with concurrency. It is a process which makes the parts of a program run independently and parallelly enhancing the efficiency of the program. Go routines and channels are used to execute concurrency. Merge sort is a divide and conquer algorithm used to sort the unsorted array or slice by dividing the input slice into smaller sub-slices, individually sort them recursively and then merge them into a single slice which is sorted. Syntax func make ([] type, size, capacity) The make function ... Read More

240 Views
In this article, we will write Go language programs to compute the factorial of a number using concurrency. It is a task of implementing multiple operations simultaneously and can be implemented using Go routines and channels. Go routines are the lightweight threads and channels help in non-conflicting communications between the routines. Syntax func make ([] type, size, capacity) The make function in go language is used to create an array/map it accepts the type of variable to be created, its size and capacity as arguments. Algorithm This program imports the necessary packages main and fmt. In this ... Read More