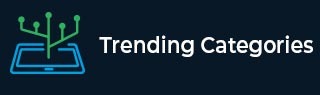
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

209 Views
When working with strings in Python, it is often necessary to validate whether a string contains only numbers and alphabets or if it includes other special characters. String validation is crucial in various scenarios such as input validation, data processing, and filtering. In this article, we will explore a Python program to test if a given string consists of only alphanumeric characters. We will discuss the criteria for a valid string, provide examples of valid and invalid strings, and present an efficient approach to solve this problem using built-in string methods. Understanding the Problem Before we dive into solving the ... Read More

2K+ Views
In Python programming, lists are a versatile and commonly used data structure. They allow us to store and manipulate collections of elements efficiently. At times, we may need to swap the positions of two elements within a list, either to reorganize the list or to perform specific operations. This blog post explores a Python program that swaps two elements in a list. We will discuss the problem, outline an approach to solve it, and provide a step-by-step algorithm. By understanding and implementing this program, you will gain the ability to manipulate lists and change the arrangement of elements according to ... Read More

1K+ Views
CAPTCHA, short for Completely Automated Public Turing test to tell Computers and Humans Apart is an important test used in many websites to check whether the person accessing the website is a human or a bot. This is done mainly for security reasons so that bots cannot intrude on the website and throw false statistics and skew internet traffic. Its main purpose is to generate tests that are easy for humans, but difficult for bots or other computers to come up with a solution. They present tests that make use of a user’s cognitive and thinking abilities. ... Read More

430 Views
Regular expressions, commonly referred to as regex, are powerful tools for pattern matching and manipulation of text in Python. They allow you to define patterns and search for matches within strings, making them extremely useful in various applications such as data validation, text processing, and web scraping. In regex, metacharacters play a crucial role. These special characters have a predefined meaning and are used to build complex patterns. Understanding and utilizing metacharacters effectively can significantly enhance your regex skills. In this article, we will explore the world of Python regex metacharacters. We will learn about different metacharacters and how they ... Read More

49 Views
In many programming scenarios, we come across situations where we need to determine if all elements in a list are maximum of K positions apart. This problem arises in various domains, such as data analysis, sequence processing, and algorithmic challenges. Being able to test and validate such conditions is essential for ensuring the integrity and correctness of our programs. In this article, we will explore a Python program to solve this problem efficiently. We'll discuss the concept, present a step-by-step approach to tackle the problem, and provide a working code implementation. By the end of this article, you will have ... Read More

343 Views
Natural Language Processing (NLP) allows computers to interpret and analyze human language. Finding the most identical word or sentence to a given input sentence is a prevalent NLP problem. In Python, there are various methods available to find identical sentences. Required Resources To get this done you have to install nltk library in your system. Therefore run the following command in your Python command prompt to install nltk. pip install nltk You may also run the following command in your Windows cmd if the above command fails to execute. python --version pip --version pip ... Read More

67 Views
When working with lists in Python, it can be valuable to identify non-neighbors, which are elements that are not adjacent to each other. Whether it's finding elements that are at least a certain distance apart or identifying gaps in a sequence, the ability to test for non-neighbors can provide valuable insights and facilitate specific operations. In this article, we will explore a Python program that tests for non-neighbors in a list. We will discuss the importance of identifying non-neighbors in various scenarios and provide a step-by-step explanation of the approach and algorithm used. Understanding the Problem Before we dive into ... Read More

399 Views
Swapping the values of two variables is a common operation in programming. Typically, the swap is performed using a third variable to temporarily store one of the values. However, in some cases, we may want to swap two numbers without using an additional variable. This can be particularly useful in scenarios where memory optimization is a concern or when working with restricted environments. In this article, we will explore a Python program that allows us to swap two numbers without using a third variable. We will discuss the traditional approach of using a temporary variable for swapping and introduce an ... Read More

421 Views
Pandas Dataframe, a python open-source library, is used for storing, deleting, modifying, and updating data in tabular form. It is designed so that I can easily integrate with Python programs for data analysis. It provides various ways of data manipulation techniques and tools for processing data. The mathematical notion of geometric means is a highly useful concept for the determination of average or central tendencies within a given set of numerical data. This is achieved by multiplying each individual number present within the data set, resulting in an nth root. The value of n, in turn, is dictated by ... Read More

1K+ Views
In this article, we will explore a Python program to swap the first and last character of a string. Swapping characters within a string can be a useful operation in various scenarios, such as data manipulation, text processing, or even string encryption. By swapping the first and last characters, we can transform the string and potentially change its meaning or representation. We will dive into the details of solving this problem efficiently using Python. We will discuss the approach, provide a step-by-step algorithm, and implement the solution using Python code. Additionally, we will include test cases to validate the program's ... Read More