- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9321 Articles for Object Oriented Programming

2K+ Views
strictfp is used to ensure that floating points operations give the same result on any platform. As floating points precision may vary from one platform to another. strictfp keyword ensures the consistency across the platforms.strictfp can be applied to class, method or on interfaces but cannot be applied to abstract methods, variable or on constructors.ExampleFollowing are the valid examples of strictfp usage.strictfp class Test { } strictfp interface Test { } class A { strictfp void Test() { } }Following are the invalid strictfp usage.class A { strictfp float a; } class A { strictfp abstract void test(); } class A { strictfp A() { } }

50K+ Views
Call by Value means calling a method with a parameter as value. Through this, the argument value is passed to the parameter.While Call by Reference means calling a method with a parameter as a reference. Through this, the argument reference is passed to the parameter.In call by value, the modification done to the parameter passed does not reflect in the caller's scope while in the call by reference, the modification done to the parameter passed are persistent and changes are reflected in the caller's scope.Following is the example of the call by value −The following program shows an example of ... Read More

725 Views
Following is a simple example of a single dimensional array.Example Live Demopublic class Tester { public static void main(String[] args) { double[] myList = {1.9, 2.9, 3.4, 3.5}; // Print all the array elements for (double element: myList) { System.out.print(element + " "); } } }Output1.9 2.9 3.4 3.5

200 Views
Java supports single dimensional and multidimensional arrays. See the example below:Example Live Demopublic class Tester { public static void main(String[] args) { double[] myList = {1.9, 2.9, 3.4, 3.5}; // Print all the array elements for (double element: myList) { System.out.print(element + " "); } System.out.println(); int[][] multidimensionalArray = { {1,2},{2,3}, {3,4} }; for(int i = 0 ; i < 3 ; i++) { //row for(int j = 0 ; j < 2; j++) { System.out.print(multidimensionalArray[i][j] + " "); } System.out.println(); } } }Output1.9 2.9 3.4 3.5 1 2 2 3 3 4

2K+ Views
Following are various methods of Object class −protected Object clone() - Used to create and return a copy of this object. boolean equals(Object obj) - Used to indicate whether some other object is "equal to" this one.protected void finalize() - garbage collector calls this method on an object when it determines that there are no more references to the object.Class getClass() - Used to get the runtime class of this Object.int hashCode() - Used to get a hash code value for the object.void notify() - Used to wake up a single thread that is waiting on this object's monitor.void notifyAll() - ... Read More

4K+ Views
Yes, an overridden method can have a different access modifier but it cannot lower the access scope.The following rules for inherited methods are enforced -Methods declared public in a superclass also must be public in all subclasses.Methods declared protected in a superclass must either be protected or public in subclasses; they cannot be private.Methods declared private are not inherited at all, so there is no rule for them.
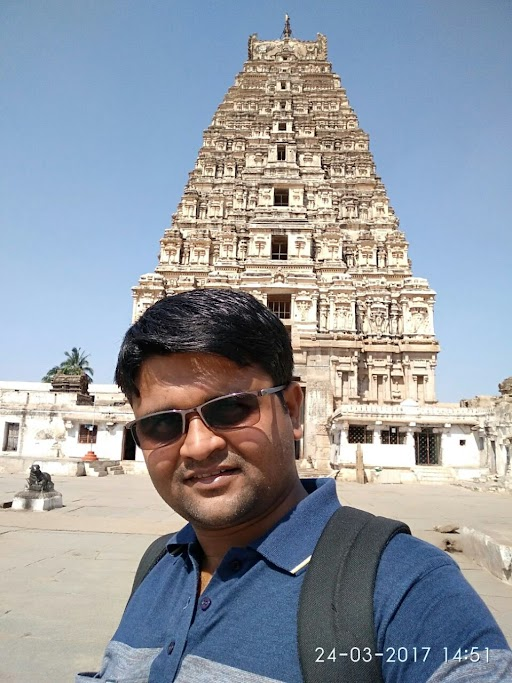
11K+ Views
Classes are the fundamental building blocks of Java. It is a programmable template that can be expanded upon, and it determines the form and characteristics of an item. One may say that a class is the most fundamental component of an object oriented programming language like Java. Each and every idea that will be put into action by means of a Java application must first be encased inside of a class. In object-oriented programming, the fundamental building blocks are called classes objects.Variables and methods are the building blocks of a Java class. Instance variables are the terms used to refer ... Read More

6K+ Views
Yes! Java supports constructor overloading. In constructor loading, we create multiple constructors with the same name but with different parameters types or with different no of parameters.ExampleLive Demopublic class Tester { private String message; public Tester(){ message = "Hello World!"; } public Tester(String message){ this.message = message; } public String getMessage(){ return message ; } public void setMessage(String message){ this.message = message; } public static void main(String[] args) { Tester tester = new Tester(); System.out.println(tester.getMessage()); Tester tester1 = new Tester("Welcome"); System.out.println(tester1.getMessage()); } } OutputHello World! Welcome

12K+ Views
Anonymous object in Java means creating an object without any reference variable. Generally, when creating an object in Java, you need to assign a name to the object. But the anonymous object in Java allows you to create an object without any name assigned to that object. So, if you want to create only one object in a class, then the anonymous object would be a good approach. Reading this article, you will learn what an anonymous object is and how to create and use anonymous objects in Java. Let's get started! Anonymous Object in Java Anonymous means Nameless. An ... Read More