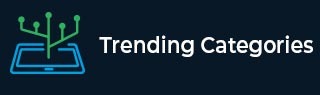
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 4219 Articles for MySQLi

9K+ Views
You can use DATE() from MySQL to select records with a particular date. The syntax is as follows.SELECT *from yourTableName WHERE DATE(yourDateColumnName)=’anyDate’;To understand the above syntax, let us first create a table. The query to create a table is as follows.mysql> create table AllRecordsFromadate -> ( -> Id int, -> Name varchar(100), -> Age int, -> AdmissionDate datetime -> ); Query OK, 0 rows affected (0.53 sec)Insert some records in the table using insert command. The query to insert records is as follows.mysql> insert into AllRecordsFromadate values(101, 'John', 23, '2018-10-13'); Query OK, 1 row affected (0.18 sec) mysql> insert ... Read More

874 Views
To sort string number, use the CAST() function from MySQL. The syntax is as follows −SELECT *FROM yourTableName ORDER BY (yourColumnName as Decimal(integerValue, integerValueAfterDecimalPoint)) desc;To understand the above syntax, let us first create a table. The query to create a table is as follows −mysql> create table SortingStringDemo -> ( -> Amount varchar(10) -> ); Query OK, 0 rows affected (0.91 sec)Insert some records in the table using insert command. The query is as follows.mysql> insert into SortingStringDemo values('12.34'); Query OK, 1 row affected (0.21 sec) mysql> insert into SortingStringDemo values('124.50'); Query OK, 1 row affected (0.56 sec) ... Read More

6K+ Views
You can use CAST() function from MySQL to achieve this. The syntax is as follows −SELECT CAST(yourColumnName as Date) as anyVariableName from yourTableName;To understand the above syntax, let us first create a table. The query to create a table is as follows −mysql> create table ConvertDateTimeToDate -> ( -> ArrivalDatetime datetime -> ); Query OK, 0 rows affected (0.37 sec)Insert the datetime in the table using insert command. The query is as follows −mysql> insert into ConvertDateTimeToDate values(date_add(now(), interval -1 year)); Query OK, 1 row affected (0.19 sec) mysql> insert into ConvertDateTimeToDate values('2017-11-21 13:10:20'); Query OK, 1 row affected ... Read More

7K+ Views
To add columns at a specific position in existing table, use after command. The syntax is as follows −ALTER TABLE yourTableName ADD COLUMN yourColumnName data type AFTER yourExistingColumnName;To understand the above syntax, let us first create a table. The query to create a table is as follows.mysql> create table changeColumnPosition -> ( -> Id_Position1 int, -> Name_Position2 varchar(100), -> Address_Position4 varchar(200) -> ); Query OK, 0 rows affected (0.53 sec)Now you can check the description of existing table using desc command. The syntax is as follows −desc yourTableName;The following is the query to check the description.mysql> desc changeColumnPosition;The following is ... Read More

9K+ Views
In MySQL, auto increment counter starts from 0 by default, but if you want the auto increment to start from another number, use the below syntax.ALTER TABLE yourTable auto_increment=yourIntegerNumber;To understand the above syntax, let us first create a table. The query to create a table is as follows.mysql> create table startAutoIncrement -> ( -> Counter int auto_increment , -> primary key(Counter) -> ); Query OK, 0 rows affected (0.90 sec)Implement the above syntax to begin auto increment from 20. The query is as follows.mysql> alter table startAutoIncrement auto_increment=20; Query OK, 0 rows affected (0.30 sec) Records: 0 Duplicates: 0 Warnings: ... Read More

4K+ Views
The symbol in MySQL is same as not equal to operator (!=). Both gives the result in boolean or tinyint(1). If the condition becomes true, then the result will be 1 otherwise 0.Case 1 − Using != operator.The query is as follows −mysql> select 3!=5;The following is the output.+------+ | 3!=5 | +------+ | 1 | +------+ 1 row in set (0.00 sec)Case 2 − Using operator.The query is as follows −mysql> select 3 5;The following is the output.+--------+ | 3 5 | +--------+ | 1 | +--------+ 1 row in set (0.00 ... Read More

1K+ Views
You can use INFORMATION_SCHEMA.COLUMNS to describe all tables in database through a single statement. The syntax is as follows.SELECT *FROM INFORMATION_SCHEMA.COLUMNS WHERE TABLE_SCHEMA=’yourDatabaseName’\GHere I am using my database sample with two tables.The table names are as follows −mytableyourtableImplement the above syntax for your database. The query is as follows −mysql> select * FROM information_schema.columns WHERE table_schema = 'sample'\GThe following is the output describing the two tables in our database.*************************** 1. row *************************** TABLE_CATALOG: def TABLE_SCHEMA: sample TABLE_NAME: mytable COLUMN_NAME: id ORDINAL_POSITION: 1 COLUMN_DEFAULT: NULL IS_NULLABLE: YES DATA_TYPE: int CHARACTER_MAXIMUM_LENGTH: NULL CHARACTER_OCTET_LENGTH: NULL NUMERIC_PRECISION: 10 NUMERIC_SCALE: 0 DATETIME_PRECISION: NULL CHARACTER_SET_NAME: NULL ... Read More

612 Views
There is no whoami function in MySQL. The whoami can be used to know the current user in UNIX. Use user() or current_user() function from MySQL for the same purpose.The following is the output.+-----------+ | version() | +-----------+ | 8.0.12 | +-----------+ 1 row in set (0.00 sec)Case 1 −Using CURRENT_USER() function.The query to know the current user is as follows.mysql> select current_user();The following is the output.+----------------+ | current_user() | +----------------+ | root@% | +----------------+ 1 row in set (0.00 sec)Case 2 − Using USER() function.The query is as follows −mysql> select user();The following is ... Read More

6K+ Views
To select increment counter in MySQL, first you need to declare and initialize a variable. The syntax is as follows −set @anyVariableName=0; select yourColumnName, @anyVariableName:=@anyVariableName+1 as anyVariableName from yourTableName;To understand the above syntax and set an increment counter, let us first create a table. The query to create a table is as follows.mysql> create table incrementCounterDemo -> ( -> Name varchar(100) -> ); Query OK, 0 rows affected (1.01 sec)Insert some records in the table using insert command. The query is as follows.mysql> insert into incrementCounterDemo values('John'); Query OK, 1 row affected (0.18 sec) mysql> insert into incrementCounterDemo values('Carol'); ... Read More

403 Views
You can select values that meet different conditions on different rows using IN() and GROUP BY. The syntax is as follows −SELECT yourColumnName1 from yourTableName WHERE yourColumnName2 IN(value1, value2, .....N) GROUP BY yourColumnName1 HAVING COUNT(DISTINCT yourColumnName2)=conditionValue;To understand the above syntax, let us first create a table. The query to create a table is as follows −mysql> create table DifferentRows -> ( -> FirstRow int, -> SecondRow int -> ); Query OK, 0 rows affected (0.72 sec)Insert some records in the table using insert command. The query is as follows −mysql> insert into DifferentRows values(10, 10); Query OK, 1 row affected ... Read More