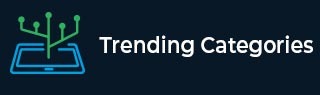
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 4219 Articles for MySQLi

8K+ Views
Equivalent of Microsoft SQL Server IDENTITY column in MySQL is AUTO_INCREMENT. The IDENTITY in SQL Server acts like AUTO_INCREMENT in MySQL.The syntax is as follows −CREATE TABLE yourTableName ( yourColumnName1 dataType NOT NULL AUTO_INCREMENT, yourColumnName2 dataType, . . . N, PRIMARY KEY(yourColumnName1) );In MySQL, you need to use primary key if your column is auto_increment otherwise MySQL will give an error. Look at the error −mysql> create table EquivalentOfIdentityInMySQL -> ( -> ProductId int NOT NULL AUTO_INCREMENT, -> ProductName varchar(30) -> ); ERROR 1075 (42000) − Incorrect table definition; ... Read More

375 Views
You can update two columns using SET command separated with comma(, ). The syntax is as follows −UPDATE yourTableName SET yourColumnName1 = ’yourValue1’, yourColumnName2 = ’yourValue2’ where yourCondition;To understand the above syntax, let us create a table. The query to create a table is as follows −mysql> create table StudentInformations -> ( -> StudentId int not null auto_increment, -> StudentFirstName varchar(20), -> StudentLastName varchar(20), -> Primary Key(StudentId) -> ); Query OK, 0 rows affected (0.57 sec)Insert some records in the table using insert command. The query is as follows −mysql> insert into StudentInformations(StudentFirstName, StudentLastName) ... Read More

3K+ Views
To change the year in MySQL date, you need to use DATE_FORMAT() function with UPDATE command. The syntax is as follows.UPDATE yourTableName SET yourDateColumnName = DATE_FORMAT(yourDateColumnName ,'yourYearValue-%m-%d');To understand the above syntax, let us create a table. The query to create a table is as follows −mysql> create table ChangeYear -> ( -> id int not null auto_increment, -> ArrivalTime date, -> PRIMARY KEY(id) -> ); Query OK, 0 rows affected (0.83 sec)Insert some records in the table using insert command −mysql> insert into ChangeYear(ArrivalTime) values(date_add(now(), interval -2 year)); Query OK, 1 row affected, 1 warning ... Read More

265 Views
Order by the choice of strings you want, using the FIELD() function. The syntax is as follows −SELECT *FROM yourTableName ORDER BY FIELD(yourColumnName, ’yourValue1’, ’yourValue2’, ’yourValue3’, ....N);To understand the above syntax, let us create a table. The query to create a table is as follows −mysql> create table OrderByListOfStrings -> ( -> Id int not null auto_increment, -> CarName varchar(20), -> PRIMARY KEY(Id) -> ); Query OK, 0 rows affected (0.68 sec)Insert some records in the table using insert command. The query is as follows −mysql> insert into OrderByListOfStrings(CarName) values('Ford'); Query OK, 1 row affected ... Read More

96 Views
To get the output MySQL query result in CSV format, use concat_ws(). The syntax is as follows −SELECT CONCAT_WS(‘, ’, yourColumnName1, yourColumnName2, yourColumnName3, ....N) as anyVariableName from yourTableName;To understand the above syntax, let us create a table. The query to create a table is as follows −mysql> create table CSVFormatOutputs -> ( -> StudentId int not null auto_increment, -> StudentName varchar(20), -> StudentAge int, -> PRIMARY KEY(StudentId) -> ); Query OK, 0 rows affected (1.15 sec)Insert some records in the table using insert command. The query is as follows −mysql> insert into CSVFormatOutputs(StudentName, StudentAge) ... Read More

2K+ Views
To order by like in MySQL, use the case statement. The syntax is as follows −SELECT *FROM yourTableName ORDER BY CASE WHEN yourColumnName like '%yourPatternValue1%' then 1 WHEN yourColumnName like '%yourPatternValue2%' then 2 else 3 end;To understand the above syntax, let us create a table. The query to create a table is as follows −mysql> create table OrderByLikeDemo -> ( -> Id int, -> Name varchar(100) -> ); Query OK, 0 rows affected (1.84 sec)Insert some records in the table using insert command. The query is as follows −mysql> insert into OrderByLikeDemo values(100, ... Read More

172 Views
You can get max and second max salary from an Employee table using subquery.Let us first create a table. The query to create a table is as follows −mysql> create table EmployeeMaxAndSecondMaxSalary -> ( -> EmployeeId int, -> Employeename varchar(20), -> EmployeeSalary int -> ); Query OK, 0 rows affected (0.88 sec)Insert some records in the table using insert command −mysql> insert into EmployeeMaxAndSecondMaxSalary values(1, 'John', 34566); Query OK, 1 row affected (0.20 sec) mysql> insert into EmployeeMaxAndSecondMaxSalary values(2, 'Bob', 56789); Query OK, 1 row affected (0.17 sec) mysql> insert into EmployeeMaxAndSecondMaxSalary values(3, ... Read More

512 Views
To get the output MySQL query result in CSV format, use concat(). The syntax is as follows −mysql> select concat(StudentId, ', ', StudentName, ', ', StudentAge) as CSVFormat from CSVFormatOutputs;To understand the above syntax, let us create a table. The query to create a table is as follows−mysql> create table CSVFormatOutputs -> ( -> StudentId int not null auto_increment, -> StudentName varchar(20), -> StudentAge int, -> PRIMARY KEY(StudentId) -> ); Query OK, 0 rows affected (1.15 sec)Insert some records in the table using insert command. The query is as follows −mysql> insert into CSVFormatOutputs(StudentName, ... Read More

351 Views
You can get max and second max salary from an Employee table using LIMIT OFFSET. The syntax is as follows −SELECT yourColumnName1, yourColumnName2, ....N from yourTableName ORDER BY yourColumnName desc limit 2 offset 0;To understand the above syntax, let us create a table. The query to create a table is as follows −mysql> create table EmployeeMaxAndSecondMaxSalary -> ( -> EmployeeId int, -> Employeename varchar(20), -> EmployeeSalary int -> ); Query OK, 0 rows affected (0.88 sec)Insert some records in the table using insert command −mysql> insert into EmployeeMaxAndSecondMaxSalary values(1, 'John', 34566); Query OK, 1 row ... Read More

322 Views
You can add column values without using aggregate function like sum(). For that, the syntax is as follows −SELECT *, (yourColumnName1+yourColumnName2+yourColumnName3, ....N) as anyVariableName from yourTableName;To understand the above syntax, let us create a table. The query to create a table is as follows −mysql> create table AddingColumnDemo -> ( -> StudentId int, -> StudentName varchar(20), -> MathMarks int, -> PhysicsMarks int, -> ChemistryMarks int -> ); Query OK, 0 rows affected (0.82 sec)Insert records in the table using insert command. The query is as follows −mysql> insert into AddingColumnDemo values(1, 'John', 35, ... Read More