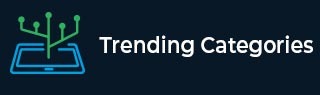
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 4219 Articles for MySQLi

3K+ Views
You can count values from comma-separated field using CHAR_LENGTH() method from MySQL. The syntax is as follows −SELECT *, (CHAR_LENGTH(yourColumnName) - CHAR_LENGTH(REPLACE(yourColumnName, ', ', '')) + 1) as anyVariableName from yourTableName;To understand the above syntax, let us create a table. The query to create a table is as follows −mysql> create table CountValuesCommaSeparated -> ( -> Id int NOT NULL AUTO_INCREMENT, -> CommaSeparatedValue text, -> PRIMARY KEY(Id) -> ); Query OK, 0 rows affected (1.76 sec)Insert some records in the table using insert command. The query is as follows −mysql> insert into CountValuesCommaSeparated(CommaSeparatedValue) values('101, 104, ... Read More

2K+ Views
To update an entire row in MySQL, use UPDATE command. You need to know the primary key column. The syntax is as follows to update an entire row.UPDATE yourTableName SET yourColumnName1 = ’yourValue1’ ,yourColumnName2 = ’yourValue2’ , yourColumnName3 = ’yourValue3’ ,.......................N WHERE yourPrimaryKeyColumnName = yourValue;To understand the above syntax, let us create a table. The query to create a table is as follows −mysql> create table UpdateEntireRowDemo -> ( -> Id int NOT NULL AUTO_INCREMENT, -> Name varchar(20), -> Age int, -> Marks int, -> PRIMARY KEY(Id) -> ); Query OK, ... Read More

888 Views
There are lots of options available to replace NULL values using select statement. You can use CASE statement or IFNULL() or COALESCE()Case 1 − Using IFNULL()The syntax of IFNULL() is as follows −SELECT IFNULL(yourColumnName, ’yourValue’) AS anyVariableName from yourTableName;Case 2 − Using COALESCE()The syntax of COALESCE() is as follows −SELECT COALESCE(yourColumnName, ’yourValue’) AS anyVariableName from yourTableName;Case 3 − Using CASE statementThe syntax of CASE statement.SELECT CASE WHEN yourColumnName IS NULL THEN ‘yourValue’ ELSE yourColumnName END AS anyVariableName FROM yourTableNameTo understand what we discussed above, let us create a table. The query to create a table is as follows −mysql> create ... Read More

339 Views
In order to work with hex, use the CONV() function to convert between bases. The syntax is as follows −SET anyVariableName = CONV(yourHexValue, 16, 10);To understand the above syntax, let us create a stored procedure. The query to create a stored procedure is as follows −mysql> DELIMITER // mysql> CREATE PROCEDURE SP_HEX_TO_DEC( HEXVALUE VARCHAR(10) ) -> BEGIN -> DECLARE Decimalvalue INTEGER; -> SET Decimalvalue = CONV(HEXVALUE, 16, 10); -> select Decimalvalue; -> END; -> // Query OK, 0 rows affected (0.19 sec) mysql> DELIMITER ;The above stored procedure converts the hexadecimal to decimal. As ... Read More

333 Views
You can use CREATE TABLE command to create a table from an existing table. The syntax is as follows:CREATE TABLE yourNewTableName LIKE yourOldTableNameTo understand the above syntax, let us create a table. The query to create a table is as follows:mysql> create table ShowCreateTableCommand -> ( -> Id int NOT NULL AUTO_INCREMENT, -> FirstName varchar(20), -> LastName varchar(20), -> Age int, -> TotalMarks int, -> PRIMARY KEY(Id) -> ); Query OK, 0 rows affected (1.22 sec)Here is the query to describe a table using DESC command. The query is as follows:mysql> desc ... Read More

109 Views
You need to use ALTER command to change collation to utf8_bin. The syntax is as follows:ALTER TABLE yourTableName COLLATE utf8_general_ci;To understand the above syntax, let us create a table. The query to create a table is as follows:mysql> create table CollateDemo -> ( -> Id int NOT NULL AUTO_INCREMENT, -> Name varchar(20), -> Age int, -> PRIMARY KEY(Id) -> ); Query OK, 0 rows affected (0.98 sec)Check the DDL of the table. The syntax is as follows:SHOW CREATE TABLE yourTableName;Let us now check the DDL of our table:mysql> show create table CollateDemo;The following is ... Read More

1K+ Views
You can use binary to search for exact string in MySQL. The syntax is as follows:SELECT * FROM yourTableName WHERE BINARY yourColumnName = yourStringValue;To understand the above syntax, let us create a table. The query to create a table is as follows:mysql> create table ExactSearch -> ( -> Id int NOT NULL AUTO_INCREMENT, -> UserId varchar(10), -> UserName varchar(20), -> PRIMARY KEY(Id) -> ); Query OK, 0 rows affected (0.69 sec)Insert some records in the table using insert command. The query is as follows:mysql> insert into ExactSearch(UserId, UserName) values('USER12', 'John'); Query OK, 1 row ... Read More

18K+ Views
Use STR_TO_DATE() method from MySQL to convert. The syntax is as follows wherein we are using format specifiers. The format specifiers begin with %.SELECT STR_TO_DATE(yourDateColumnName, '%d.%m.%Y') as anyVariableName FROM yourTableName;To understand the above syntax, let us create a table. The query to create a table is as follows.mysql> create table ConvertIntoDateFormat -> ( -> Id int NOT NULL AUTO_INCREMENT, -> LoginDate varchar(30), -> PRIMARY KEY(Id) -> ); Query OK, 0 rows affected (0.47 sec)Insert some records in the table using insert command. The query is as follows −mysql> insert into ConvertIntoDateFormat(LoginDate) values('11.01.2019'); Query OK, 1 ... Read More

44 Views
You can use CASE statement to get the results that match some expressions−SELECT *FROM yourTableName WHERE CASE WHEN yourColumnName1 = yourValue1 THEN 1 ELSE 0 END + CASE WHEN yourColumnName2 = yourValue2 THEN 1 ELSE 0 END + CASE WHEN yourColumnName3 = yourValue3 THEN 1 ELSE 0 END + . . CASE WHEN yourColumnNameN = yourValueN THEN 1 ELSE 0 END > = 3;To understand the above syntax, let us create a table. The query to create a table is as follows −mysql> create table UserInformation -> ( -> Id int NOT NULL AUTO_INCREMENT, ... Read More

2K+ Views
You can select all records that are 10 minutes within current timestamp using the following syntax−SELECT *FROM yourTableName WHERE yourColumnName > = DATE_SUB(NOW(), INTERVAL 10 MINUTE);To understand the above syntax, let us create a table. The query to create a table is as follows−mysql> create table users -> ( -> Id int NOT NULL AUTO_INCREMENT, -> UserName varchar(20), -> UserLastseen datetime, -> PRIMARY KEY(Id) -> ); Query OK, 0 rows affected (0.91 sec)Insert some records in the table using insert command. The query is as follows−mysql> insert into users(UserName, UserLastseen) values('Larry', '2019-01-15 02−45−00'); Query ... Read More