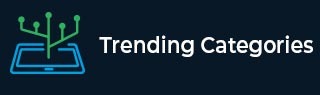
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 4219 Articles for MySQLi

18K+ Views
Once you have created the statement object you can execute it using one of the execute methods of the Statement interface namely, execute(), executeUpdate() and, executeQuery().The execute() method: This method is used to execute SQL DDL statements, it returns a boolean value specifying weather the ResultSet object can be retrieved.Exampleimport java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.sql.Statement; public class Example { public static void main(String args[]) throws SQLException { //Registering the Driver DriverManager.registerDriver(new com.mysql.jdbc.Driver()); //Getting the connection String mysqlUrl = "jdbc:mysql://localhost/sampleDB"; Connection con = ... Read More

6K+ Views
The CallableStatement interface provides methods to execute the stored procedures. Since the JDBC API provides a stored procedure SQL escape syntax, you can call stored procedures of all RDBMS in single standard way.Creating a CallableStatementYou can create an object of the CallableStatement (interface) using the prepareCall() method of the Connection interface. This method accepts a string variable representing a query to call the stored procedure and returns a CallableStatement object.A Callable statement can have input parameters, output parameters or both. To pass input parameters to the procedure call you can use place holder and set values to these using the ... Read More

269 Views
You can use aggregate function count along with if() for this. To understand the concept, let us create a table. The query to create a table is as followsmysql> create table CountOccurrencesDemo -> ( -> Id int NOT NULL AUTO_INCREMENT PRIMARY KEY, -> TechnicalSubject varchar(100) -> ); Query OK, 0 rows affected (0.68 sec)Now you can insert some records in the table using insert command. The query is as followsmysql> insert into CountOccurrencesDemo(TechnicalSubject) values('Java'); Query OK, 1 row affected (0.14 sec) mysql> insert into CountOccurrencesDemo(TechnicalSubject) values('MongoDB'); Query OK, 1 row affected (0.13 sec) mysql> insert into ... Read More

948 Views
You need to use REGEXP for this. The syntax is as followsSELECT *FROM yourTableName WHERE yourColumnName REGEXP '[a-zA-Z]';To understand the concept, let us create a table. The query to create a table is as followsmysql> create table SelectNonNumericValue -> ( -> Id int NOT NULL AUTO_INCREMENT PRIMARY KEY, -> UserId varchar(100) -> ); Query OK, 0 rows affected (0.58 sec)Insert some records in the table using insert command. The query is as followsmysql> insert into SelectNonNumericValue(UserId) values('123John'); Query OK, 1 row affected (0.12 sec) mysql> insert into SelectNonNumericValue(UserId) values('58475Carol98457Taylor24'); Query OK, 1 row affected (0.52 sec) ... Read More

3K+ Views
You need to use CREATE command to create a new user with password in MySQL 8. Let us check the versionmysql> select version(); +-----------+ | version() | +-----------+ | 8.0.12 | +-----------+ 1 row in set (0.14 sec)The syntax is as follows to create a new user with passwordCREATE USER 'yourUserName'@'localhost' IDENTIFIED BY 'yourPassword';The following is the syntax to grant all privileges to the created userGRANT ALL ON *.* TO 'yourUserName'@'localhost';Now flush the privileges using flush commandflush privileges; Let us create a new user with the help of the above syntax. The query is as followsmysql> use MySQL; Database ... Read More

474 Views
To understand the MySQL select statement DISTINCT for multiple columns, let us see an example and create a table. The query to create a table is as followsmysql> create table selectDistinctDemo -> ( -> InstructorId int NOT NULL AUTO_INCREMENT PRIMARY KEY, -> StudentId int, -> TechnicalSubject varchar(100) -> ); Query OK, 0 rows affected (0.50 sec)Insert some records in the table using insert command. The query is as followsmysql> insert into selectDistinctDemo(StudentId, TechnicalSubject) values(121, 'Java'); Query OK, 1 row affected (0.15 sec) mysql> insert into selectDistinctDemo(StudentId, TechnicalSubject) values(121, 'MongoDB'); Query OK, 1 row affected (0.16 ... Read More

502 Views
For this, you need to use an INSERT SELECT statement. The syntax is as followsINSERT INTO yourDatabaseName1.yourTableName1(yourColumnName1, yourColumnName2, ....N) SELECT yourColumnName1, yourColumnName2, ....N FROM yourdatabaseName2.yourTableName2;Here, I am using the following two databasessampletestLet us create the first table in the “test” databasemysql> use test; Database changed mysql> create table send -> ( -> Id int NOT NULL AUTO_INCREMENT PRIMARY KEY, -> Name varchar(20) -> ); Query OK, 0 rows affected (1.19 sec)Insert some records in the first table using insert command. The query is as followsmysql> insert into send(Name) values('John'); Query OK, 1 row affected ... Read More

1K+ Views
Let us first create a table. The query to create a table is as followsmysql> create table FirstAndLastDataDemo -> ( -> EmployeeId int NOT NULL AUTO_INCREMENT PRIMARY KEY, -> EmployeeName varchar(20), -> EmployeeAge int -> ); Query OK, 0 rows affected (0.59 sec)Insert some records in the table using insert command. The query is as followsmysql> insert into FirstAndLastDataDemo(EmployeeName, EmployeeAge) values('John', 23); Query OK, 1 row affected (0.15 sec) mysql> insert into FirstAndLastDataDemo(EmployeeName, EmployeeAge) values('Bob', 13); Query OK, 1 row affected (0.11 sec) mysql> insert into FirstAndLastDataDemo(EmployeeName, EmployeeAge) values('Larry', 24); Query OK, 1 row affected ... Read More

721 Views
You can use INFORMATION_SCHEMA.COLUMNS table to display MySQL table name with columns. The syntax is as follows −SELECT DISTINCT TABLE_NAME, Column_Name FROM INFORMATION_SCHEMA.COLUMNS WHERE TABLE_SCHEMA = 'yourDatabaseName';Here, we have a database with the name ‘sample’ with tables. The query is as follows to display table name along with column name −mysql> SELECT DISTINCT TABLE_NAME, Column_Name -> FROM INFORMATION_SCHEMA.COLUMNS -> WHERE TABLE_SCHEMA = 'sample';Output+--------------------------------+-------------------------------+ | TABLE_NAME | COLUMN_NAME | +--------------------------------+-------------------------------+ | aggregatefunctiondemo | UserId ... Read More

2K+ Views
Achieve this with the help of where clause.The syntax is as followsSELECT yourColumnName1, yourColumnName2, ...N FROM yourTableName WHERE ( SELECT COUNT(*) FROM yourTableName )=2;To understand the concept, let us create a table. The query to create a table is as followsmysql> create table CountWithSubqueryDemo - > ( - > Id int NOT NULL AUTO_INCREMENT PRIMARY KEY, - > EmployeeName varchar(20) - > ); Query OK, 0 rows affected (2.09 sec)Insert some records in the table using insert command.The query is as followsmysql> insert into CountWithSubqueryDemo(EmployeeName) values('John'); Query OK, 1 row affected (0.54 sec) mysql> ... Read More