- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 6685 Articles for Javascript
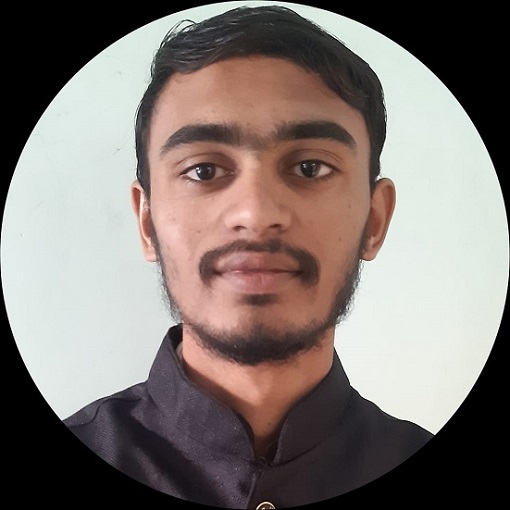
2K+ Views
In this tutorial, we will see if we can declare JavaScript variables as a specific type or not. The JavaScript contains the three reserve keywords to declare the variables: ‘let, ’ ‘var’, and ‘const.’ When the user declares the variable using any keyword, it has a different scope. The variable declared with the const keyword always remains constant, and we can’t change its value after initializing it. The variables declared with the let keyword have block scope and can’t be accessed outside its scope. When we declare the variables with the var keyword, it can have the global scope or ... Read More
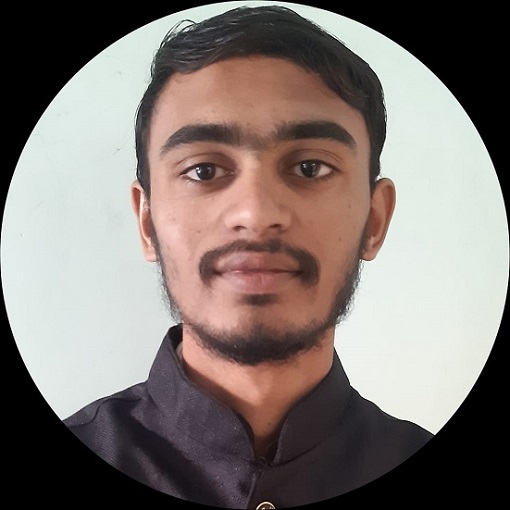
375 Views
This tutorial teaches us to make an array empty in JavaScript. While programming with JavaScript, programmers need to make an array empty in many conditions. For example, coders are doing competitive programming with JavaScript. Suppose, to solve some problem, they need to create a new or an empty array while calling the function every time. Users can visualize this problem from the below example.function child ( ){ // create a new array or use a single array and make it empty every time function invokes } function parent ( ) { for ( int ... Read More
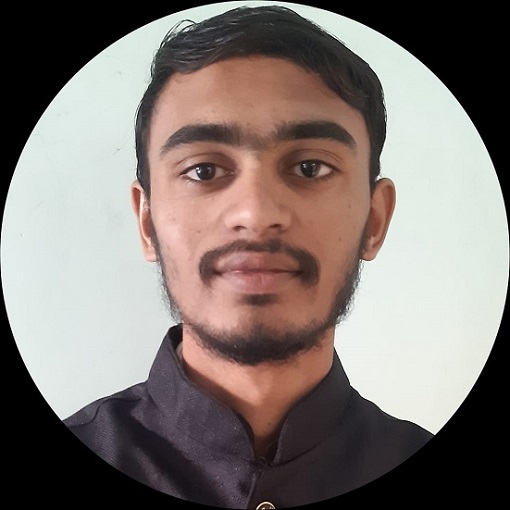
3K+ Views
In this tutorial, we will learn to check the existence of the variable, which means whether the variable is declared and initialized or not in JavaScript. In many scenarios, JavaScript programmers need to check the presence of the variable. Otherwise, it throws a reference error if we use it without declaring or defining it.For example, Programmers have defined the variable and it is uninitialized. Programmers want to change the variables' value through the data they will get from API calls. Suppose that the value of the uninitialized variable is not updated due to some error during the API call. If ... Read More

159 Views
To check a variable is ‘undefined’, you need to check using the following. If the result is “false”, it means the variable is not defined. Here, the variable results in “True” −ExampleLive Demo var points = 100; if(points){ document.write("True"); }else{ document.write("False"); }

280 Views
functionDisplayOne is a function expression, however, functionDisplayTwo is a function declaration. It is defined as soon as its surrounding function is executed.Both the ways are used to declare functions in JavaScript and functionDisplayOne is an anonymous function.Here’s the function expression −functionDisplayOne(); var functionDisplayOne = function() { console.log("Hello!"); };The following is the function declaration −functionDisplayTwo(); function functionDisplayTwo() { console.log("Hello!"); }

154 Views
On getting the result of the following, you can find whether a variable is ‘undefined’. If the result is “false”, it means the variable is undefined. Here, the variable results in “True” Example Live Demo var res = 10; if(res) { document.write("True"); } else { document.write("False"); }

175 Views
To generate a random number, use the JavaScript Math.random() method. Here set the minimum and maximum value and generate a random number between them as in the following code −ExampleLive Demo var max = 6 var min = 2 var random = Math.floor(Math.random() * (max - min + 1)) + min; document.write(random)
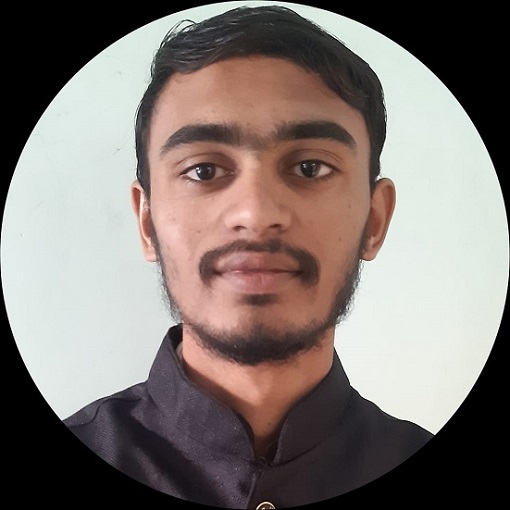
8K+ Views
In this tutorial, we will learn how to pass JavaScript Variables with AJAX calls. AJAX stands for Asynchronous JavaScript and XML. As the name suggests, it performs asynchronous operations on your web page. AJAX communicates with the server by HTTP requests and gets the required data as an HTTP response. We can control these HTTP requests by passing the JavaScript variables with AJAX calls. There are various types of HTTP requests, and in this tutorial, we will discuss the most popular HTTP requests by using AJAX and also pass JavaScript variables with it. Pass JavaScript Variables with “GET” AJAX calls ... Read More

1K+ Views
Like many other programming languages, JavaScript has variables. Variables can be thought of as named containers. You can place data into these containers and then refer to the data simply by naming the container.JavaScript variables get stored in the memory of the browser process. The following ways can work for storing variables:The variables which you declare in your JavaScript code gets saved in the memory of the browser process.Cookies can also store variables, they are often saved on your hard disk;

125 Views
Definitely, the following way of declaring multiple variables is more efficient:var variable1 = 5; var variable2 = 3.6; var variable3 = “Amit”;Suppose you need to add, remove, or update a variable, then you can easily do it.But with the following method, you need to do more changes. For example, on removing a variable, you need to remove the semi-colon. If it’s the first, then you need to add var to the second variable.var variable1 = 5, variable2 = 3.6, variable3 = “Amit”