- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 6685 Articles for Javascript

1K+ Views
To pass a parameter to setTimeout() callback, use the following syntax −setTimeout(functionname, milliseconds, arg1, arg2, arg3...)The following are the parameters −function name − The function name for the function to be executed.milliseconds − The number of milliseconds.arg1, arg2, arg3 − These are the arguments passed to the function.ExampleYou can try to run the following code to pass a parameter to a setTimeout() callbackLive Demo Submit function timeFunction() { setTimeout(function(){ alert("After 5 seconds!"); }, 5000); } Click the above button and wait for 5 seconds.

628 Views
The datetime in Javascript and in Python have 2 major differences. First one being the meaning of the month argument.The month in Javascript is expected between 0-11 while in Python it is expected to be between 1-12. So the following tuple actually represents 2 different dates in Python and in Javascript −(2017, 11, 1) Python: 1st November 2017 Javascript: 1sd December 2017The second difference is that they have different default timezones, with Python defaulting to UTC while JavaScript defaults to the user's "local" timezone. You can use Date.UTC(), which returns the timestamp, for the equivalent in JavaScript. For example,var utc = Date.UTC(2013, 7, 10);

121 Views
To execute an anonymous function you need to use the leading bang! or any other symbol −!function(){ // do stuff }();You can also write it like −+function(){ // do stuff }();Even the following is an acceptable syntax −~function(){ // do stuff return 0; }( );

65 Views
To determine if an argument is sent to function, use “default” parameters.ExampleYou can try to run the following to implement itLive Demo function display(arg1 = 'Tutorials', arg2 = 'Learning') { document.write(arg1 + ' ' +arg2+""); } display('Tutorials', 'Learning'); display('Tutorials'); display();

1K+ Views
To call multiple JavaScript functions in on click event, use a semicolon as shown below −onclick="Display1();Display2()"ExampleLive Demo function Display1() { document.write ("Hello there!"); } function Display2() { document.write ("Hello World!"); } Click the following button to call the function

95 Views
The JavaScript eval() is used to execute an argument. The code gets execute slower when the eval() method is used. It also has security implementations since it has a different scope of execution.ExampleHere’s how you can implement eval() function − var a = 30; var b = 12; var res1 = eval("a * b") + ""; var res2 = eval("5 + 10") + ""; document.write(res1); document.write(res2);
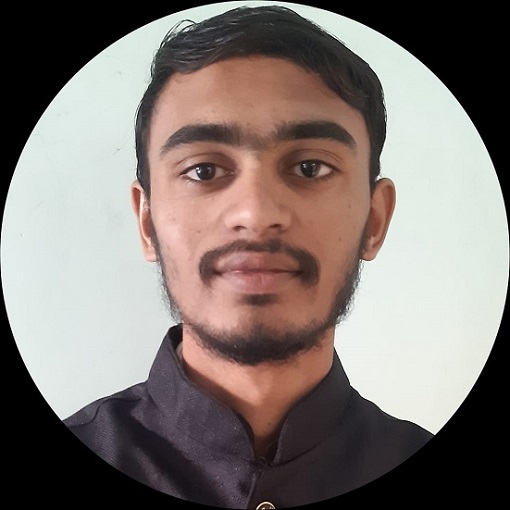
49K+ Views
This tutorial will teach us to call a JavaScript function on page load. In many cases, while programming with HTML and JavaScript, programmers need to call a function, while loading the web page or after the web page load finishes. For example, programmers need to show the welcome message to the user on page load, and the programmer needs to call a JavaScript function after the page load event.There are various ways to call a function on page load or after page load in JavaScript, and we will look at them one by one in this tutorial.Using the onload event ... Read More

7K+ Views
++a returns the value of an after it has been incremented. It is a pre-increment operator since ++ comes before the operand.a++ returns the value of a before incrementing. It is a post-increment operator since ++ comes after the operand.ExampleYou can try to run the following code to learn the difference between i++ and ++i − var a =10; var b =20; //pre-increment operator a = ++a; document.write("++a = "+a); //post-increment operator b = b++; document.write(" b++ = "+b);

134 Views
Using “javascript:void(0)” is definitely better, since its faster. Try to run both the examples in Google Chrome with the developer tools. The “javascript:void(0)” method takes less time than the only #.Here’s the usage of “javascript: void(0)”:If inserting an expression into a web page results in an unwanted effect, then use JavaScript void to remove it. Adding “JavaScript:void(0)”, returns the undefined primitive value.The void operator is used to evaluate the given expression. After that, it returns undefined. It obtains the undefined primitive value, using void(0).The void(0) can be used with hyperlinks to obtain the undefined primitive valueExampleLive Demo ... Read More

123 Views
To split a string on every occurrence of ~, split the array. After splitting, add a line break i.e. for each occurrence of ~.For example, This is demo text 1!~This is demo text 2!~~This is demo text 3!After the split and adding line breaks like the following for ~ occurrence −This is demo text 1! This is demo text 2! This is demo text 3!ExampleLet’s see the complete exampleLive Demo Adding line break var myArray = 'This is demo text 1!~This is demo text 2!~ ... Read More