- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 6685 Articles for Javascript

91K+ Views
This tutorial will teach us how to format a number with two decimals using JavaScript. Formatting a number means rounding the number up to a specified decimal place. In JavaScript, we can apply many methods to perform the formatting. Some of them are listed as follows − The toFixed() Method Math.round() Method Math.floor() Method Math.ceil() Method The toFixed() Method The toFixed() method formats a number with a specific number of digits to the right of the decimal. it returns a string representation of the number that does not use exponential notation and has the exact number of digits after ... Read More
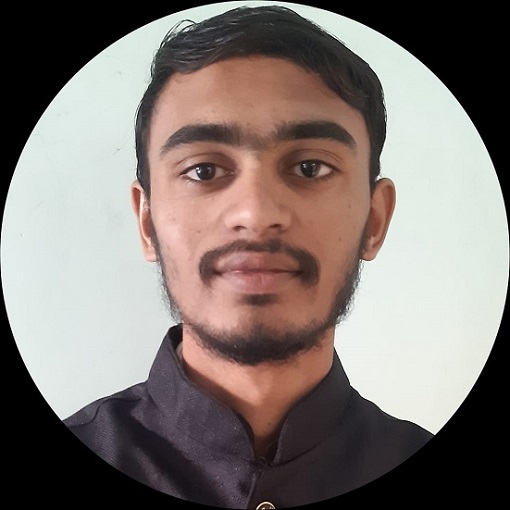
2K+ Views
In this tutorial, we will learn how to perform integer division and get the remainder in JavaScript. The division operator (/) returns the quotient of its operands, where the dividend is on the left, and the divisor is on the right. When another splits one operand, the remainder operator (%) returns the residual. It always takes the dividend sign. In the operation x% y, x is known as the dividend, and y is known as the divisor. If one of the operands is NaN, x is Infinity, or y is 0, the operation returns NaN. Otherwise, the dividend x is ... Read More
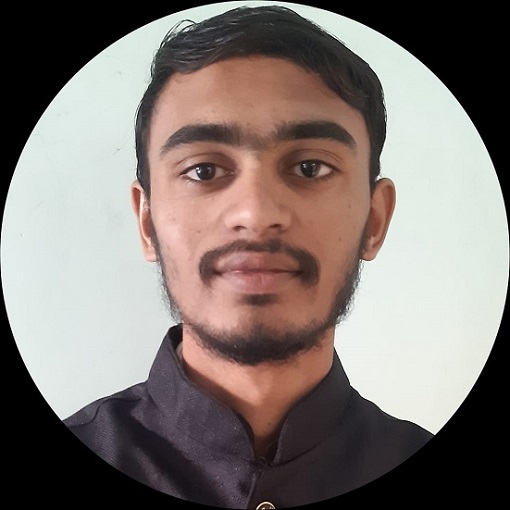
7K+ Views
This tutorial teaches us to find the number of days between the two dates in JavaScript. If you are a developer, sometimes you face the scenario where you need to find the number of days between 2 dates.For example, if you are building a software application to keep track of employees' attendance and salary. If an employee leaves without completing the month, you need to count the number of days the employee attended in the current month to give the salary. Furthermore, there can be many scenarios like the above example.In this tutorial, we will see the different methods to ... Read More

1K+ Views
To get dates in JavaScript, use the getTime() method. Forgetting the difference between two dates, calculate the difference between date and time.ExampleYou can try to run the following code to learn how to calculate a difference between two dates −Live Demo var dateFirst = new Date("11/25/2017"); var dateSecond = new Date("11/28/2017"); // time difference var timeDiff = Math.abs(dateSecond.getTime() - dateFirst.getTime()); // days difference var diffDays = Math.ceil(timeDiff / (1000 * 3600 * 24)); // difference alert(diffDays);

91 Views
Here’s the best way to read cookies by name in JavaScript. The following is the function −function ReadCookie() { var allcookies = document.cookie; document.write ("All Cookies : " + allcookies ); // Get all the cookies pairs in an array cookiearray = allcookies.split(';'); // Now take key value pair out of this array for(var i = 0; i < cookiearray.length; i++) { name = cookiearray[i].split('=')[0]; value = cookiearray[i].split('=')[1]; document.write ("Key is : " + name + " and Value is : " + value); } }

10K+ Views
To generate a random number, we use the Math.random() function. This method returns a floating-point number in the range 0 (inclusive) to 1 (exclusive). To generate random numbers in different range, we should define the minimum and maximum limits of the range. Please follow the second syntax below for this. To generate random integers, you can follow the third and fourth syntax discussed below. As the Math.random() method gives us a random floating point number so to convert the result into an integer, we need to apply some basic math. Please follow the below syntaxes. Syntax Following is the syntax ... Read More

933 Views
The standard Unix epoch is an expression in seconds. The Unix epoch or Unix time is the number of seconds elapsed since January 1, 1970.The getTime() method is used to return the millisecond representation of the Date object.To get time in milliseconds, use the following:var milliseconds = (new Date).getTime();

384 Views
To get an integer in the string “abcde 30”, use the following regex in JavaScript, else it will return NaN. With that, use parseInt() to get the numbers with regex under String match() method −ExampleLive Demo var myStr = "abcdef 30"; var num = parseInt(myStr.match(/\d+/)) alert(num);

350 Views
Use the toString() method to get the string representation of a number. You can try to run the following code to print a string representation of numbers like 20, -40, 15.5 −ExampleLive Demo var num1 = 25; document.write(num1.toString()+""); document.write((30.2).toString()+""); var num2 = 3; document.write(num2.toString(2)+""); document.write((-0xff).toString(2)); Output25 30.2 11 -11111111Above, you can see we added a parameter to the toString() method. This is an optional parameter, which allows you to add an integer between 2 and 36, which is the base for representing numeric values.