- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 6685 Articles for Javascript

3K+ Views
To test if a cookie has expired in JavaScript, add the following condition and check −if( !($.cookie('myCookie') === null) ) { // Do something (cookie exists) } else { // Do something (cookie expired) }You can also check it using a single line code −checkCookie = $.cookie('myCookie') === null ? ( /*Condition TRUE...*/ ) : ( /*Condition FALSE...*/ );

433 Views
To change the styling of JavaScript alert button, use the custom alert box. You can try to run the following code to change the styling of alert buttons. The button looks different and more fancy −ExampleLive Demo function functionAlert(msg, myYes) { var confirmBox = $("#confirm"); confirmBox.find(".message").text(msg); confirmBox.find(".yes").unbind().click(function() { confirmBox.hide(); }); ... Read More
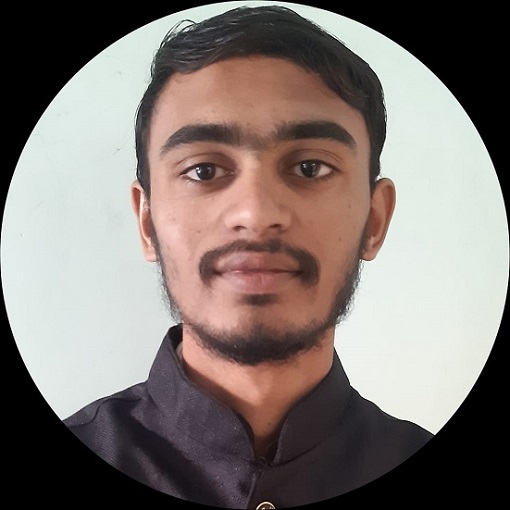
8K+ Views
In this tutorial, we will learn to change the color of the alert box in JavaScript. Also, we will learn to style the whole alert box, including the content of the alert box. In JavaScript, the alert box is the best way to show the success, failure, or informative messages to the user, when the user performs some operation on the application. The programmers can create the default alert box using the alert() method, but they can’t change the default style of the alert() box. To change the style of the alert box, programmers need to create the custom alert ... Read More
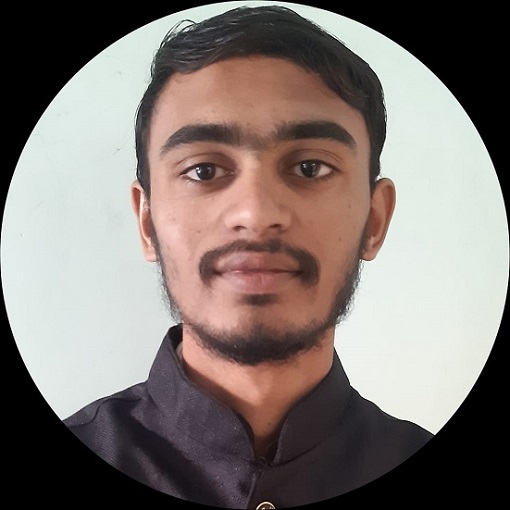
3K+ Views
In this tutorial, we will learn how to preserve leading 0 with JavaScript numbers. Sometimes we need to sort strings that are similar to numbers. For example, 10 comes before 2, but after 02 in the alphabet. Leading zeros are used to align numbers in ascending order with alphabetical order. Following are the methods used to preserve leading zero with JavaScript numbers. Using the Number as a String The number is converted into a string using string manipulation, and the leading zero is preserved. Syntax myFunc2('Neel', 27, '0165') The function myFunc2() is called, and the values are passed ... Read More

4K+ Views
In this tutorial, we will learn how we can split a JavaScript number into individual digits. Sometimes, we need to split the number into individual digits to accomplish the task given to us like checking for the palindrome number. Let us discuss the methods to split numbers into digits. When the number is taken in the form of a number. When the number is taken in the form of a string. Let us discuss both of them individually with program examples. When the input is in form of a number If the input number is in the form ... Read More

734 Views
To handle floating point number precision in JavaScript, use the toPrecision() method. It helps to format a number to a length you can specify.ExampleLive Demo var num = 28.6754; document.write(num.toPrecision(3)); document.write(""+num.toPrecision(2)); document.write(""+num.toPrecision(5)); Output28.7 29 28.675
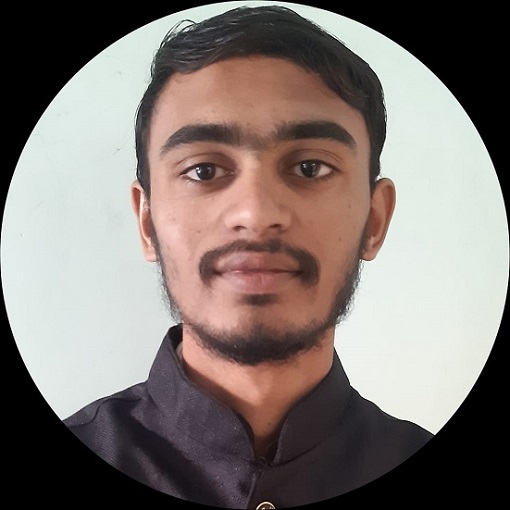
619 Views
Roman numerals are a type of number system used to represent a fixed decimal number. Several letters from the Latin alphabet are used for the representation of roman numerals. In this tutorial, the user will learn how to convert a decimal number to roman using JavaScript. Seven symbols represent Roman numerals: I, V, X, L, C, D, and M. The values for symbols are given below. Syntax I is 1 V is 5 X is 10 L is 50 C is 100 D is 500 M is 1000 Using these seven symbols user can convert decimal numbers to roman. ... Read More

2K+ Views
You can try to run the following code to change the alert message text color. To change the text color of the alert message, use the following custom alert box. We’re using JavaScript library, jQuery to achieve this and will change the alert message text color to “#FCD116” −ExampleLive Demo function functionAlert(msg, myYes) { var confirmBox = $("#confirm"); confirmBox.find(".message").text(msg); confirmBox.find(".yes").unbind().click(function() { ... Read More

2K+ Views
You will not be able to change the style of a standard alert box in JavaScript. To change the style, use the following custom alert box. We’re using JavaScript library, jQuery to achive this.ExampleLive Demo function functionAlert(msg, myYes) { var confirmBox = $("#confirm"); confirmBox.find(".message").text(msg); confirmBox.find(".yes").unbind().click(function() { confirmBox.hide(); }); ... Read More

582 Views
To count the number of occurrences of a character in a string, try to run the following code −ExampleLive Demo function displayCount() { setTimeout(function() { var str = document.getElementById('str1').value; var letter = document.getElementById('letter1').value; letter = letter[letter.length-1]; var lCount; for (var i = lCount = 0; i < str.length; lCount += (str[i++] == letter)); document.querySelector('p').innerText = lCount; return lCount; }, 50); } Enter String: Enter Letter: Click for Result result=