- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 6685 Articles for Javascript
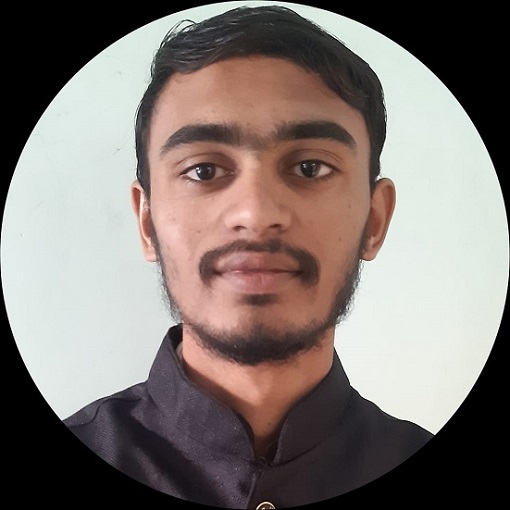
233 Views
Some old versions of the browsers or old browsers themselves don’t support the newly evolved features of JavaScript. For example, if you are using a very old browser version, it doesn’t support the features of the ES10 version of JavaScript. For example, some versions of the browser don’t support Array.falt() method, introduced in the ES10 version of JavaScript, to flatten the array. In such cases, we need to implement user-defined methods to support that features by the old browser version. Here, we will implement the polyfill for the trim() method of the String object. Syntax Users can follow the ... Read More
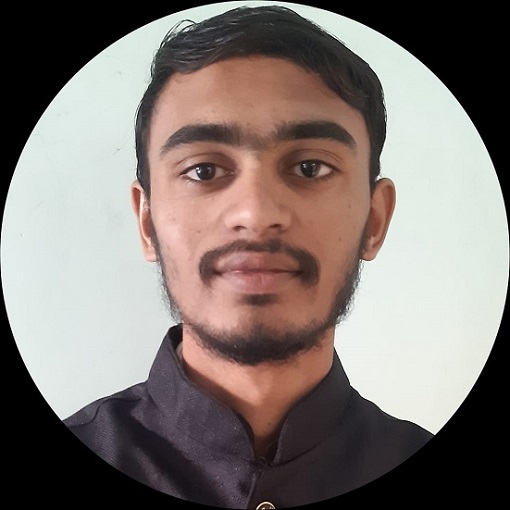
1K+ Views
The polyfill is a concept to extend the browser's features using user-defined methods. If the user’s browser is not updated, it can happen that browser doesn’t support the newer features of any programming language, such as JavaScript. As a developer, we require to check whether the feature is supported by the browser, and if not, we need to invoke a user-defined method. In this tutorial, we will discuss implementing the polyfill for the array.reduce() method. If any browser doesn’t support the array.reduce() method, we will invoke the userdefined reduce() method. Before we start with the tutorial, Let’s ... Read More
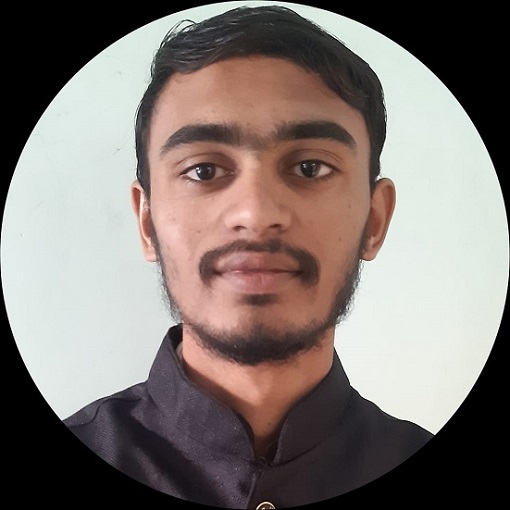
991 Views
When we click the input element in HTML, it sets the outline for the input element, focusing on it. Sometimes, we must execute some JavaScript code when the user loses focus from the input element. For example, We can autofocus the input element when the user refreshes the web page using the HTML’s ‘autoFocus’ attribute. After that, we can execute the JavaScript function when an element loses focus to ensure that the input value is not empty. If the input value is empty, and the element loses focus, we can show the error message like ‘This field is required’. ... Read More
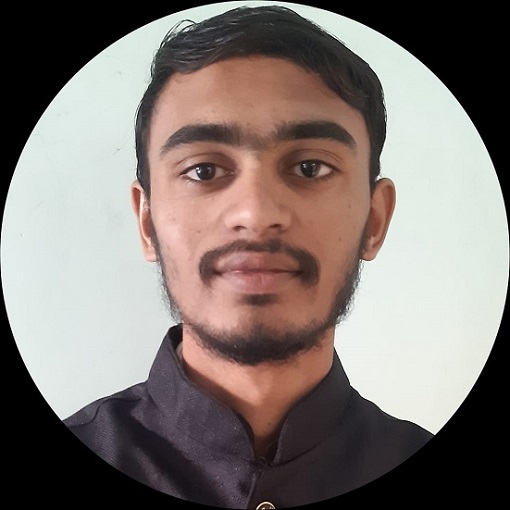
5K+ Views
Image transition means changing the image and replacing one image with another. Users can see the image transition in the image slider. While developing the image slider, we should focus on the animation for image transition to make an attractive UX of the application. In this tutorial, we will learn to add fading effect using various approaches to image transition. Add class to the image to show an image with fading effect We can use CSS to add fading effect to the image transition. The transition property of CSS allows us to add any transition to the image. So, ... Read More
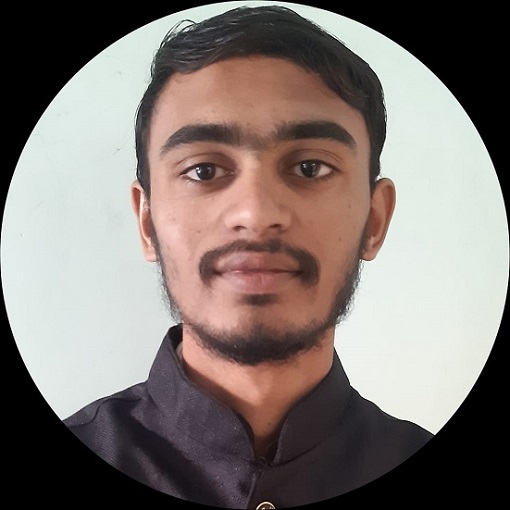
245 Views
Before creating the project, we will first discuss REST API. REST is a software architecture style and a collection of standards that helps make online services. Representational State Transfer is the full name of REST. At the same time, Application programming interfaces (API) allow communication between two or more computer programmes. It is a software interface that gives other software programmes a service. The user must follow the rules known as a REST API based on the HTTP (Hypertext Transfer Protocol) to access web services. Conventional HTTP methods like GET, POST, PUT, and DELETE access and modify resources like ... Read More
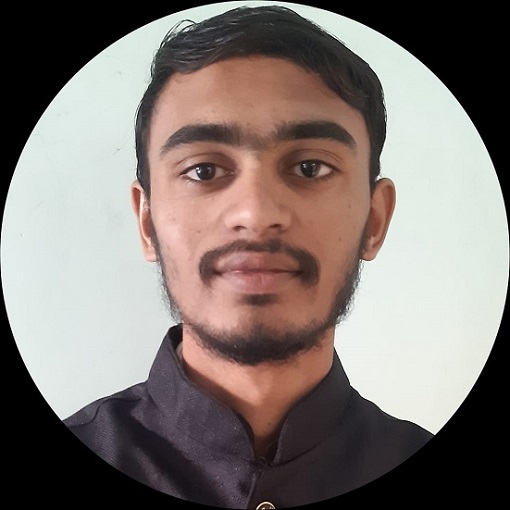
704 Views
The document.getElementById() method allows us to access any HTML element using its id in JavaScript. Every webpage can contain only a single HTML element with a single id. You can use the below example code to access any HTML element using its id. let element = document.getElementById('id'); In the above code, we used the getElementById() method of the document object and passed the id as a parameter. Now, if we require to access multiple elements using their id, it is not a good idea to use a document.getElementById(), but we can create a shorthand for it and ... Read More
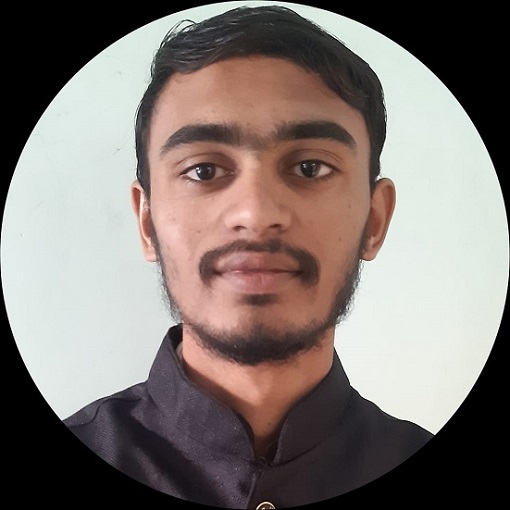
6K+ Views
Conditional statements are the most important and basic concept of any programming language. The if-else statement allows us to execute any code block conditionally. We can define the condition for the if statement in braces, and if the condition becomes true, it executes the code of the if block; Otherwise, it executes the code of the else block. Here, we have demonstrated how an if-else statement works in JavaScript. if (condition) { // code to execute when the condition becomes true } else { // ... Read More
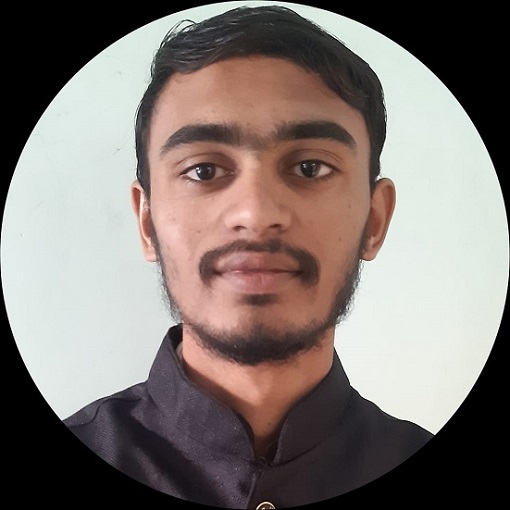
270 Views
Memoization is the optimization technique to improve the performance of the function. Before we start with the memoization technique, let’s understand why we require it using the example below. Example (Naïve approach to finding Fibonacci number) In the example below, we have implemented the naïve approach to find the nth Fibonacci number. We used the recursive approach to find the nth Fibonacci series. Finding the nth Fibonacci using recursive approach number in JavaScript Enter the number to find the nth Fibonacci number. ... Read More
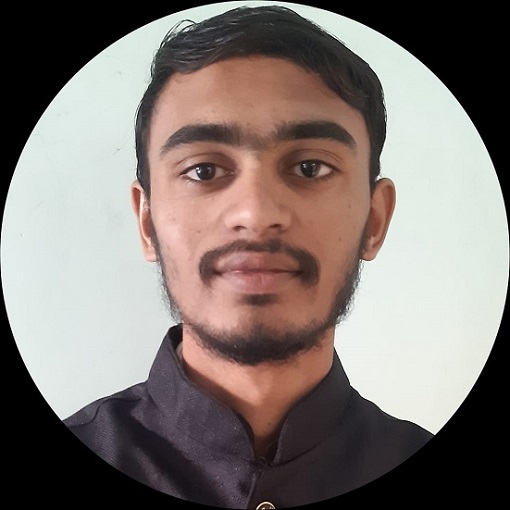
499 Views
When you have visitors from worldwide on your websites, it is a good idea to show things like a cell phone number according to international standards. So they can easily understand. We can use the International Public Telecommunication Numbering Format to represent the cell phone number in an international way. Also, it is represented by the ‘E-164’ format. We have given the syntax below to follow to write a cell phone number in an international way. [+] [country code] [local phone number] Users can see that the international phone number contains ‘+’ initially and ‘country ... Read More
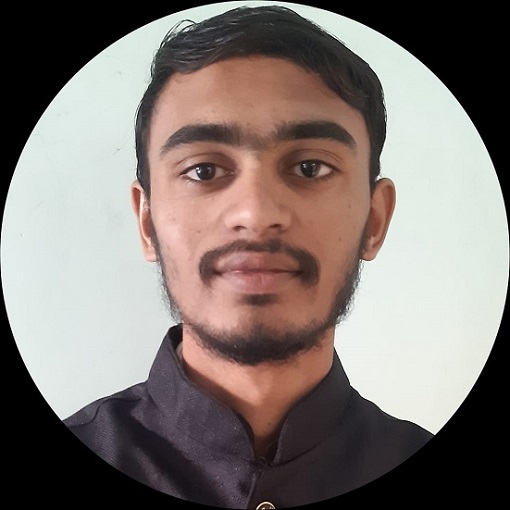
6K+ Views
The setTimeOut() method executes some block of code or functions after a particular number of milliseconds. Sometimes, we require to resolve or reject the promise after a particular delay, and we can use the setTimeout() method with the promises. In JavaScript, a promise is an object that returns the result of the asynchronous operation. Here, we will learn to resolve or reject promises after some delay using the setTimeOut() method. Example 1 (Promises without setTimeOut() method) In the example below, we have used the Promise() constructor to create a new promise. The promise constructor takes a callback ... Read More