- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 2617 Articles for Java

406 Views
To list all files in a directory and nested sub-directory, the Java program is as follows −Exampleimport java.io.File; public class Demo{ static void print_recursively(File[] my_arr, int my_index, int sub_level){ if(my_index == my_arr.length) return; for (int i = 0; i < sub_level; i++) System.out.print("\t"); if(my_arr[my_index].isFile()) System.out.println(my_arr[my_index].getName()); else if(my_arr[my_index].isDirectory()){ System.out.println("[" + my_arr[my_index].getName() + "]"); print_recursively(my_arr[my_index].listFiles(), 0, sub_level + 1); } ... Read More

750 Views
To implement static method in Interface, the Java code is as follows −Example Live Demointerface my_interface{ static void static_fun(){ System.out.println("In the newly created static method"); } void method_override(String str); } public class Demo_interface implements my_interface{ public static void main(String[] args){ Demo_interface demo_inter = new Demo_interface(); my_interface.static_fun(); demo_inter.method_override("In the override method"); } @Override public void method_override(String str){ System.out.println(str); } }OutputIn the newly created static method In the override methodAn interface is defined, inside which a static function is defined. ... Read More

651 Views
The Static Control Flow identify static members, executes static blocks, and then executes the static main method. Let us see an example −Example Live Demopublic class Demo{ static int a = 97; public static void main(String[] args){ print(); System.out.println("The main method has completed executing"); } static{ System.out.println(a); print(); System.out.println("We are inside the first static block"); } public static void print(){ System.out.println(b); } static{ System.out.println("We are inside the second static block"); } ... Read More

3K+ Views
The static block executes when classloader loads the class. A static block is invoked before main() method. Let us see an example −Example Live Democlass Demo{ static int val_1; int val_2; static{ val_1 = 67; System.out.println("The static block has been called."); } } public class Main{ public static void main(String args[]){ System.out.println(Demo.val_1); } }OutputThe static block has been called. 67A class named Demo contains a static integer value and a normal integer value. In a static block, a value is defined, and in the main class, ... Read More

285 Views
Following is the Java program for Standard Normal Distribution −Example Live Demoimport java.io.*; import java.util.*; public class Demo{ public static void main(String[] args){ double std_dev, val_1, val_3, val_2; val_1 = 68; val_2 = 102; val_3 = 26; std_dev = (val_1 - val_2) / val_3; System.out.println("The standard normal deviation is: " + std_dev); } }OutputThe standard normal deviation is: -1.3076923076923077A class named Demo contains the main function, where certain double values are defined, and the formula for standard deviation is applied ((val_1 - val_2) / val_3) on these values and the resultant output is displayed on the console.

2K+ Views
To replace a word with asterisks in a sentence, the Java program is as follows −Example Live Demopublic class Demo{ static String replace_word(String sentence, String pattern){ String[] word_list = sentence.split("\s+"); String my_result = ""; String asterisk_val = ""; for (int i = 0; i < pattern.length(); i++) asterisk_val += '*'; int my_index = 0; for (String i : word_list){ if (i.compareTo(pattern) == 0) word_list[my_index] = asterisk_val; ... Read More

2K+ Views
To check if a string contains any special character, the Java program is as follows −Example Live Demoimport java.util.regex.Matcher; import java.util.regex.Pattern; public class Demo { public static void main(String[] args){ String my_str="This is a sample only !$@"; Pattern my_pattern = Pattern.compile("[^a-z0-9 ]", Pattern.CASE_INSENSITIVE); Matcher my_match = my_pattern.matcher(my_str); boolean check = my_match.find(); if (check) System.out.println("Special character found in the string"); else System.out.println("Special character not found in the string"); } }OutputSpecial ... Read More

112 Views
Following is the Java program for Stooge sort −Example Live Demoimport java.io.*; public class Demo { static void stooge_sort(int my_arr[], int l_val, int h_val){ if (l_val >= h_val) return; if (my_arr[l_val] > my_arr[h_val]){ int temp = my_arr[l_val]; my_arr[l_val] = my_arr[h_val]; my_arr[h_val] = temp; } if (h_val-l_val+1 > 2){ int temp = (h_val-l_val+1) / 3; stooge_sort(my_arr, l_val, h_val-temp); stooge_sort(my_arr, l_val+temp, h_val); ... Read More

175 Views
To find the sum of such series, the Java program is as follows −Example Live Demopublic class Demo { static long my_val = 1000000007; public static long compute_val(long my_int){ return ((my_int % my_val) * (my_int % my_val)) % my_val; } public static void main(String[] args){ long my_int = 45687234; System.out.println("The computed value is "); System.out.print(compute_val(my_int)); } }OutputThe computed value is 335959495A class named Demo defines a function named ‘compute_val’ that computes and returns the sum of a specific series. In the main function, the ... Read More
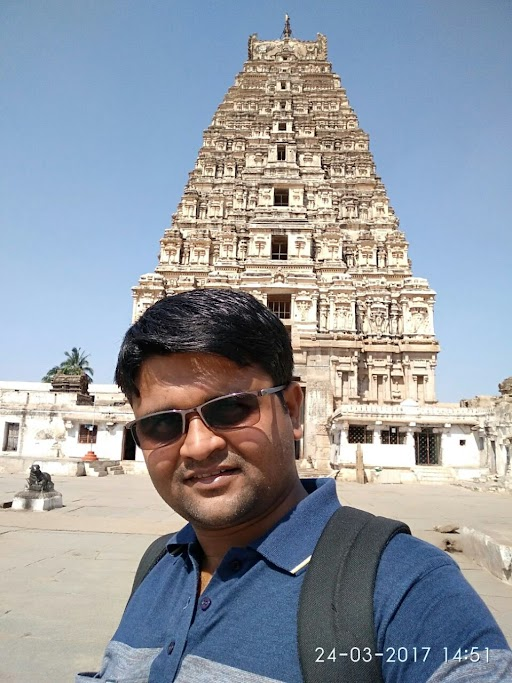
462 Views
Scala and Java are two of the most widely used high-level programming languages in the world of today's computer programming. Scala was developed specifically to address a number of Java's limitations. In particular, it is intended to be as condensed and terse as possible, with the goal of reducing the amount of code that the programmer is required to produce.Java and Scala have their own specialised fields and domains of application. Go through this article to find out the primary characteristics of these two high-level programming languages and how they are different from each other.What is Scala?Scala is a computer ... Read More