- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 6702 Articles for Database

2K+ Views
The short is equivalent to MySQL’s small int. The Java short takes 2 bytes that has the range -32768 to 32767 while MySQL smallint also take 2 bytes with same range.Here is the demo code of short in Java −public class SmallIntAsShortDemo { public static void main(String[] args) { short value = 32767; System.out.println(value); value = -32768; System.out.println(value); // value = 32768; // System.out.println(value); } }The snapshot is as follows −This will produce the following output −32767 -32768Here is the snapshot of the output we ran in EclipseIDE −The MySQL smallint takes 2 bytes with same range.

129 Views
You can use trim() for this.Let us first create a table −mysql> create table DemoTable ( UserId varchar(100) ); Query OK, 0 rows affected (0.63 sec)Insert some records in the table using insert command. Here, we have added a question mark (?) to the end of some of the strings −mysql> insert into DemoTable values('User123?'); Query OK, 1 row affected (0.18 sec) mysql> insert into DemoTable values('User777'); Query OK, 1 row affected (0.14 sec) mysql> insert into DemoTable values('User456'); Query OK, 1 row affected (0.15 sec) mysql> insert into DemoTable values('User133?'); Query OK, 1 ... Read More

413 Views
You cannot use except in MySQL. You can work with NOT IN operator to get the same result. Let us first create a table −mysql> create table DemoTable1 ( Number1 int ); Query OK, 0 rows affected (0.71 sec)Insert some records in the table using insert command −mysql> insert into DemoTable1 values(100); Query OK, 1 row affected (0.14 sec) mysql> insert into DemoTable1 values(200); Query OK, 1 row affected (0.13 sec) mysql> insert into DemoTable1 values(300); Query OK, 1 row affected (0.13 sec)Display all records from the table using select statement:mysql> select *from DemoTable1This will produce the following output −+---------+ | Number1 | +---------+ | 100 | | 200 | | 300 | +---------+ 3 rows in set (0.00 sec)Following ... Read More

109 Views
Both pow() and power() are synonyms. Let us see the syntax −select pow(yourValue1, yourValue2); OR select power(yourValue1, yourValue2);Now we will see some examples.Using pow()mysql> select POW(4, 3);This will produce the following output −+----------+ | POW(4, 3) | +----------+ | 64 | +----------+ 1 row in set (0.00 sec)Using power()mysql> select POWER(4, 3);This will produce the following output −+------------+ | POWER(4, 3) | +------------+ | 64 | +------------+ 1 row in set (0.00 sec)Let us first create a table and look into the above concept ... Read More

59 Views
You cannot use from as a column name directly because from is a reserved word in MySQL. To avoid this, you need to use backtick symbol. Let us first create a table −mysql> create table DemoTable ( Id int NOT NULL AUTO_INCREMENT PRIMARY KEY, `from` varchar(100), Name varchar(10) ); Query OK, 0 rows affected (0.92 sec)Insert some records in the table using insert command −mysql> insert into DemoTable(`from`, Name) values('US', 'John'); Query OK, 1 row affected (0.20 sec) mysql> insert into DemoTable(`from`, Name) values('UK', 'Carol'); Query OK, 1 row affected (0.14 sec) mysql> ... Read More

2K+ Views
The SQL TRUNCATE statement is used to delete all the records from a table.SyntaxTRUNCATE TABLE table_name;To delete all the records from a table from using JDBC API you need to −Register the Driver: Register the driver class using the registerDriver() method of the DriverManager class. Pass the driver class name to it, as parameter.Establish a connection: Connect to the database using the getConnection() method of the DriverManager class. Passing URL (String), username (String), password (String) as parameters to it.Create Statement: Create a Statement object using the createStatement() method of the Connection interface.Execute the Query: Execute the query using the execute() method of the Statement interface.Following JDBC program ... Read More

562 Views
Stored procedures are sub routines, segment of SQL statements which are stored in SQL catalog. All the applications that can access Relational databases (Java, Python, PHP etc.), can access stored procedures.Stored procedures contain IN and OUT parameters or both. They may return result sets in case you use SELECT statements. Stored procedures can return multiple result sets.To create a stored procedure in (MySQL) a database using JDBC API you need to −Register the Driver: Register the driver class using the registerDriver() method of the DriverManager class. Pass the driver class name to it, as parameter.Establish a connection: Connect to the database using the getConnection() method ... Read More

443 Views
You can remove a particular record from a table in a database using the DELETE query.SyntaxDELETE FROM table_name WHERE [condition];To delete a record from a table using JDBC API you need to −Register the Driver: Register the driver class using the registerDriver() method of the DriverManager class. Pass the driver class name to it, as parameter.Establish a connection: Connect to the database using the getConnection() method of the DriverManager class. Passing URL (String), username (String), password (String) as parameters to it.Create Statement: Create a Statement object using the createStatement() method of the Connection interface.Execute the Query: Execute the query using the executeUpdate() method of the ... Read More

1K+ Views
You can update/modify the existing contents of a record in a table using the UPDATE query. Using this you can update all the records of the table or specific records.SyntaxUPDATE table_name SET column1 = value1, column2 = value2...., columnN = valueN WHERE [condition];To update the contents of a record in a table using JDBC API you need to −Register the Driver: Register the driver class using the registerDriver() method of the DriverManager class. Pass the driver class name to it, as parameter.Establish a connection: Connect to the database using the getConnection() method of the DriverManager class. Passing URL (String), username (String), password (String) ... Read More
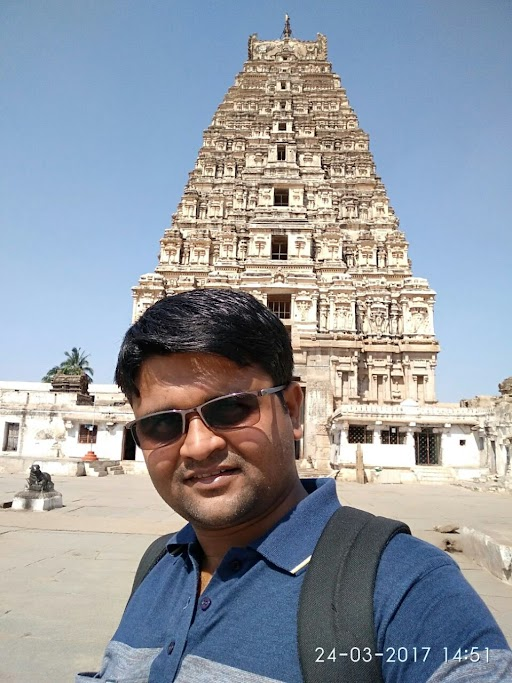
2K+ Views
In computer programming, a datatype represents the type and nature of data that is to be used by the user. It is the data type that tells the compiler or interpreter how to deal with the data and provide corresponding storing location in computer memory. According to the nature of data, the data types can be of two types namely Fundamental Datatype and Derived Datatype. Both these datatypes are extensively used in computer programming. They are equally important when we require to implement the business logic over the data. Read this tutorial to find out more about Fundamental and Derived ... Read More