- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 7346 Articles for C++
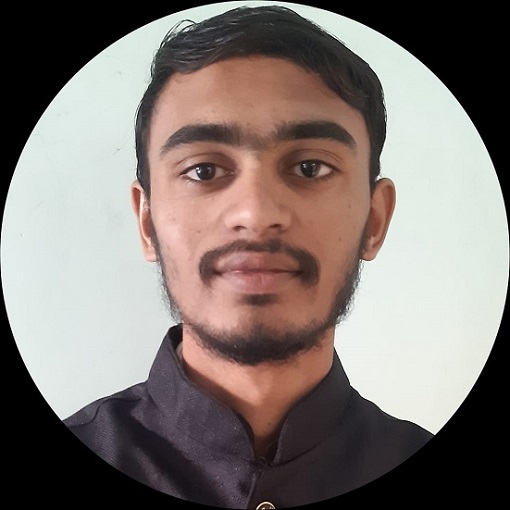
217 Views
In this problem, we will find the binary string of the given length having the same number of ‘01’ and ‘10’ subsequences. The naïve approach to solving the problem is to generate all binary strings of the given length and check whether it contains the same number of ‘10’ and ‘01’ subsequences using the dynamic programming technique. Another efficient approach is to prepare a binary string based on whether the given length is odd or even. Problem statement − We have given a positive integer ‘len’ which is greater than 2. The task is to find the binary string ... Read More
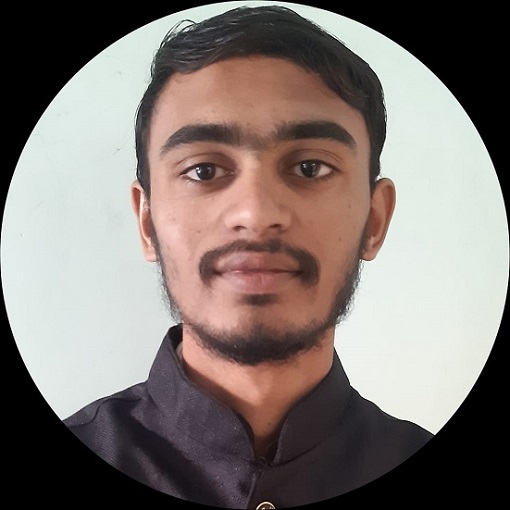
193 Views
In this problem, we will find the longest common substring using the binary search and rolling hash algorithm. The binary search is an efficient technique for searching values in the sorted array. Here, we will use it to find the maximum length of the common substring. The rolling hash algorithm is used to calculate the hash value for the string. Also, it calculates the hash value for the next substring from the previous substring’s hash value when we add or remove a character from the previous substring. Problem statement − We have given two strings named the first ... Read More
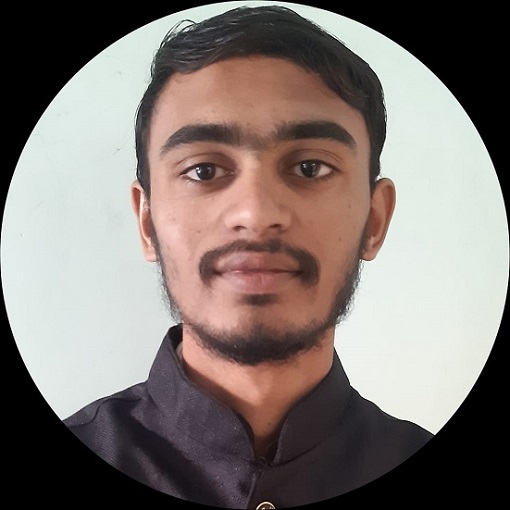
39 Views
In this problem, we will find all unique characters of the string with their first index, which is present in the string, after incrementing all characters of the given string by K. For a problem solution, we can take each unique character of the given string. Next, we can update each character individually and check whether the updated character is present in the string and not updated to another character to get the answer. Problem statement − We have given a string alpha and positive integer K. We need to increment each character’s ASCII value of the given string by ... Read More
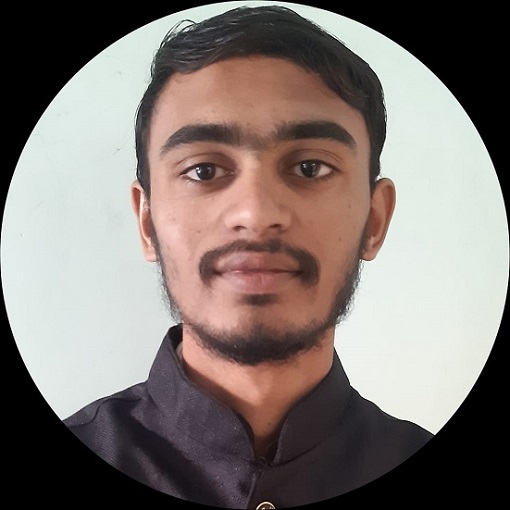
73 Views
In this problem, we will find each word of the string which comes after all words of the ‘words’ array. The first approach to solve the problem is to split the string into words and match elements of the words[] array with the string words. If we find words[] array’s element in the same order inside the string, we print the next word of the string. Another approach is to create a string of all elements of the words[] array. After that, we can find that string as a substring in the alpha string. If we find it as a ... Read More
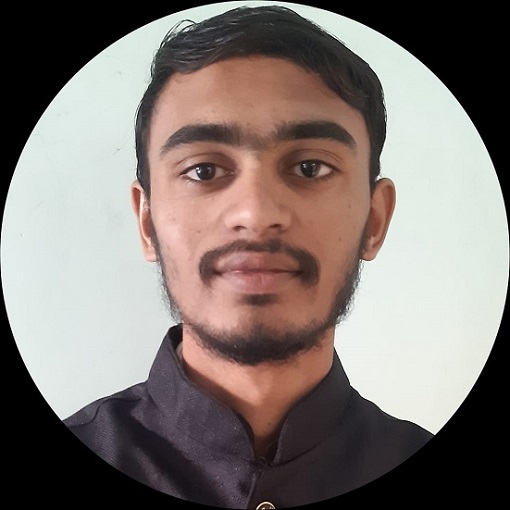
121 Views
In this problem, we will find the string of length equal to the given string so that when we sum both strings, we get the palindromic strings. Here, we can find another string such that the sum of both becomes 99999…, the largest palindromic string of the same length. If the given string starts with the ‘9’, we can find another string such that the sum of both becomes ‘11111…’. Problem statement – We have given a num string containing the numeric digits. We need to find the numeric string of the same length without leading zeros so that ... Read More
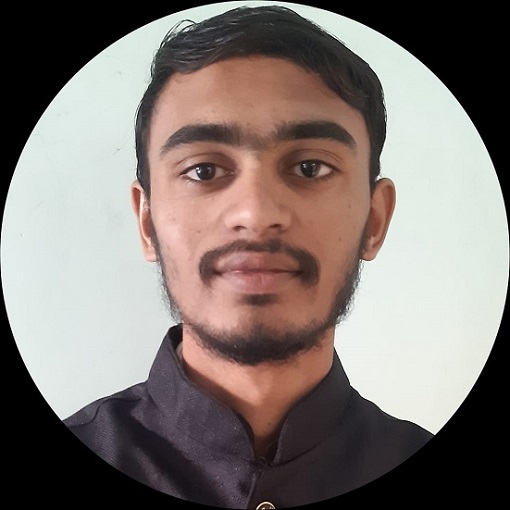
170 Views
In this problem, we will count ways to partition the given string into the K substrings such that it follows the condition given in the problem statement. We will use the recursion to solve the problem. Also, we will use the tabular dynamic programming approach to solve the problem efficiently. Problem statement − We have given a string named bin_Str of a particular length. The string contains only numeric digits from ‘0’ to ‘9’. We need to count the number of ways to partition the string in K substrings such that it follows the below condition. The substring should ... Read More
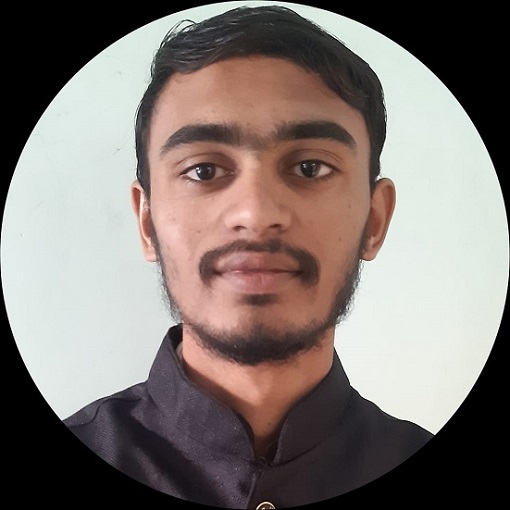
299 Views
In this problem, we will count substrings of the given binary string containing the number of ‘0’ and ‘1’ characters in the X : Y ratio. The naïve approach finds all substrings of the given binary string, counts ‘0’ and ‘1’, and checks whether the counts are in the X : Y ratio. The efficient approach uses the prefix sum technique to solve the problem. Problem statement − We have given a binary string of length bin_len. We need to count substrings having a number of 0s and 1s in the ratio of X : Y. Sample examples Input ... Read More
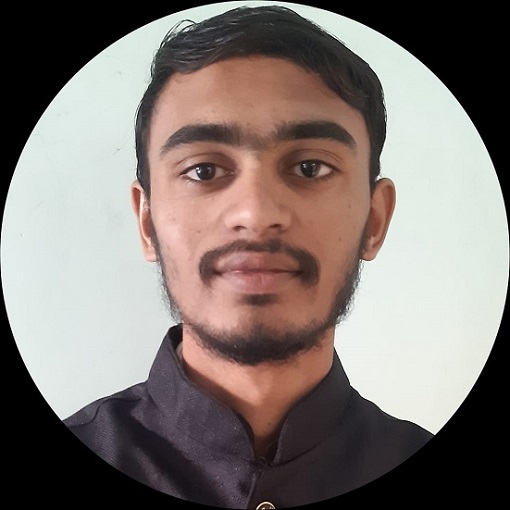
55 Views
In this problem, we will count substrings that we can make of length 1 by replacing the ‘10’ and ‘01’ substrings with ‘1’ or ‘0’ characters. When any binary string contains an equal number of ‘0’ and ‘1’, we can always make it of length 1 by performing the replacement operations. So, the problem is solved by finding the substrings with equal numbers of ‘0’ and ‘1’. Problem statement − We have given a binary string named bin_str and of length bin_len. We need to count the total number of substrings that we can make of length 1 by ... Read More
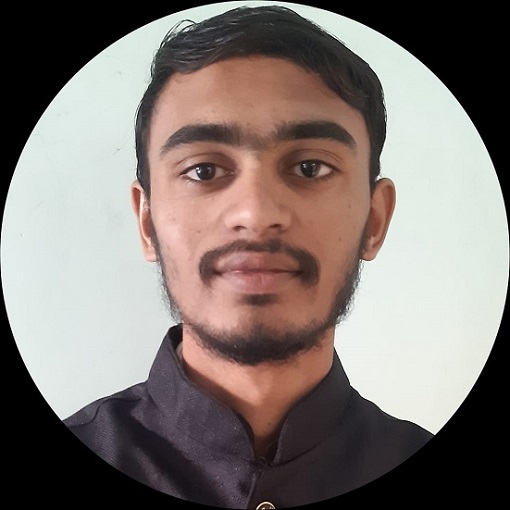
118 Views
In this problem, we will check whether we can convert string alpha2 to alpha1 by performing the XOR operation of any two adjacent characters and replacing both characters with an XOR value. We will use the logic based on the XOR value of two digits to solve the problem. If the string contains at least one ‘1’ and more than two adjacent consecutive characters, we can convert the alpha2 string to alpha1. Problem statement − We have given two binary strings of the same length. We need to check whether we can convert the alpha2 string to alpha1 by performing ... Read More
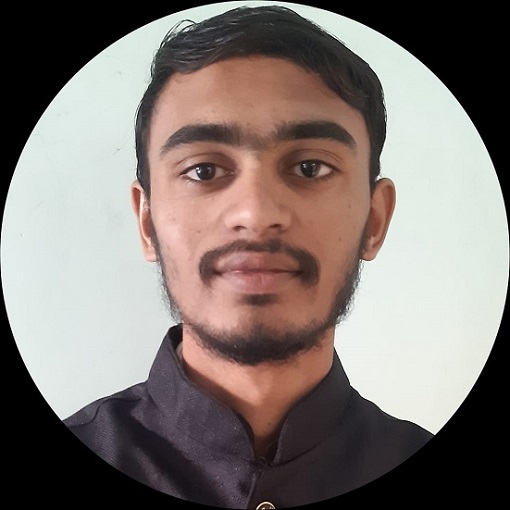
162 Views
In this problem, we will validate the Morse code. The Morse code method was used to transform the text in the encoded format, and it was very helpful to provide communication between two entities before the telephone was invented. There are standard codes for each alphabetical character containing the ‘.’ And ‘−‘. The code is prepared based on the transmission time, where ‘.’ Represents the short signals, and ‘−‘ represents the long signals. However, in this tutorial, we will use the standardized Morse code to decode and validate the string. Here is the table of standardized Morse code. ... Read More