- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 7346 Articles for C++
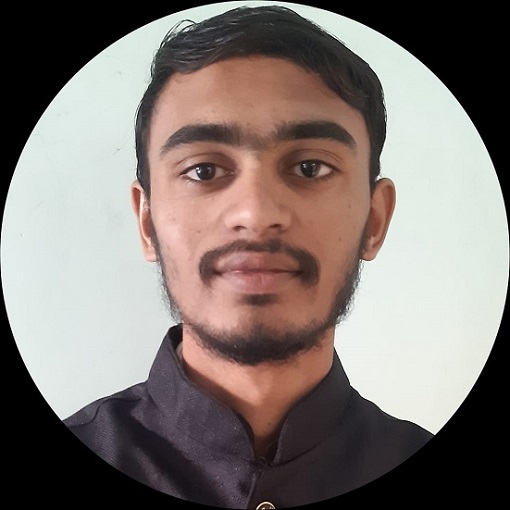
138 Views
In this problem, we will perform the given queries on the array elements. The queries contain the circular left rotation, right rotation, and updation of the array element. The logical part of solving the problem is array rotation. The naïve approach to rotating the array in the left direction is to replace each element with the next element and the last element with the first element. We can use the deque data structure to rotate the array efficiently. Problem statement − We have given an arr[] array containing the integer values. Also, we have given a queries[] array containing ... Read More

600 Views
Heap Sort − Heap sort is a comparison-based algorithm that used binary tree data structures to sort a list of numbers in increasing or decreasing order. It heap sorts to create a heap data structure where the root is the smallest element and then removes the root and again sorting gives the second smallest number in the list at the root position. Min Heap − Min heap is a data structure where the parent node is always smaller than the child node, thus root node is the smallest element among all. Problem Statement Given an array of integers. Sort them ... Read More
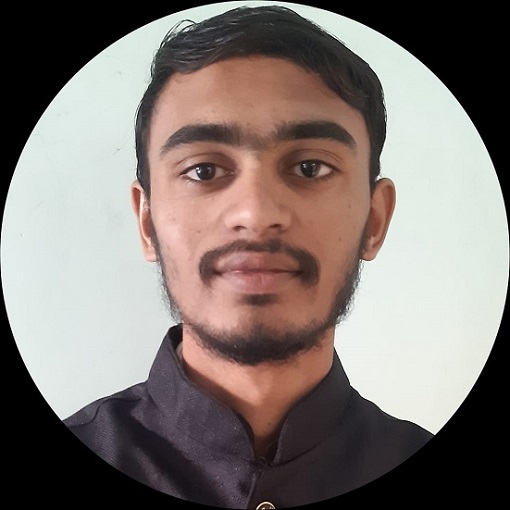
68 Views
In this problem, we will print all prime levels of the given binary tree. We will use the level order traversal technique to traverse each binary tree level and check whether all nodes contain the prime integer for the particular level. Problem statement − We have given a binary tree and need to print all prime levels of the binary tree. It is given that if all nodes of any binary tree contain a prime integer, we can say a particular level is a prime level. Sample examples Input ... Read More

448 Views
Golomb Sequence − The Golomb Sequence is a non-decreasing sequence of integers where the value of the nth term is the number of times the integer n appeared in the sequence. Some terms of Golomb sequence are, 1, 2, 2, 3, 3, 4, 4, 4, 5, 5, 5, 6, 6, 6, 6, 7, 7, 7, 7, 8, 8, 8, 8, 9, 9, 9, 9, 10, 10, 10, 10, … Here as we can see, the 5th term is 3 and 5 also appears 3 times in the sequence. The 6th term is 4 and 6 also appears 4 times in ... Read More
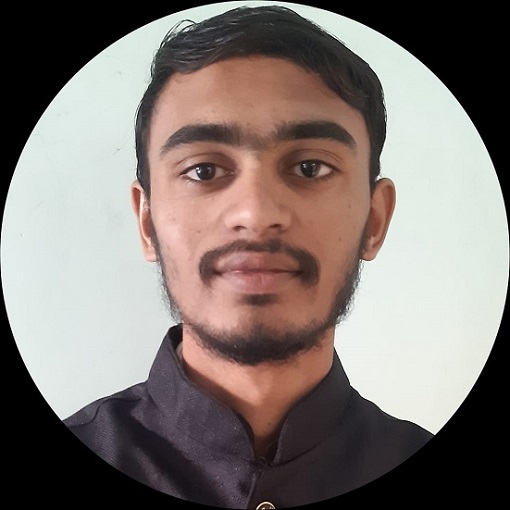
98 Views
In this problem, we will print each palindromic level of the given binary tree. We can use the breadth−first search algorithm to traverse each level of the given binary tree and check whether a particular level of the binary tree is palindromic. If yes, we print the level in the output. Problem statement − We have given a binary tree, and we need to print all palindromic levels of the binary tree. If any particular level of the binary tree contains the same numbers from left to right and right to left, we can say that the current level is ... Read More

184 Views
Unsorted Array − An array is a data structure consisting of a collection of elements of the same type. An unsorted array is such a structure where the order of elements is random, i.e. on insertion, the element is added to the last irrespective of the order of previous elements and searching in such an array is not helped by any search algorithm because of lack of a pattern of the positioning of elements. Searching − Searching in an array means finding a particular element in the array which can be either returning the position of a desired element or ... Read More
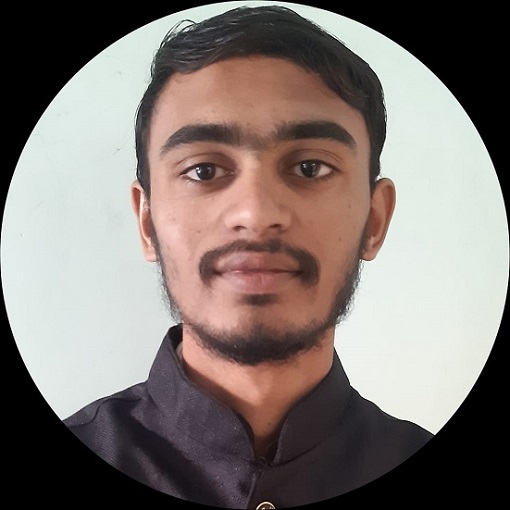
55 Views
In this problem, we will print all the nodes of the binary tree except the rightmost node of each level. We will use the level order traversal to traverse the binary tree, and we won’t print the last node of each level, which is the rightmost node. Problem statement − We have given a binary tree containing different nodes. We need to print all nodes of the binary tree except right most node. Sample examples Input 7 / \ ... Read More
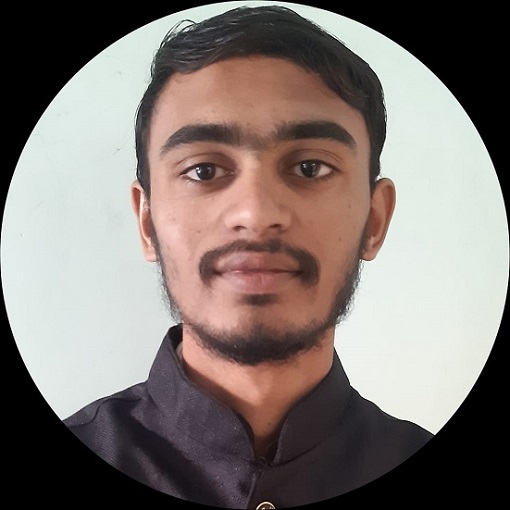
389 Views
In this problem, we will minimize the maximum difference between adjacent elements by removing any M elements from the array. The naïve approach to solving the problem is to pick total N − M array elements and check which set contains the minimum or maximum adjacent difference. The optimized approach uses the queue data structure to solve the problem. Problem statement : We have given an sorted array of numbers in sorted order. We have also given M. We need to remove M elements from the array such that we can minimize the maximum difference between the adjacent array ... Read More

109 Views
Fortunate Numbers − It is the smallest integer m > 1 such that, for a given positive integer n, pn# + m is a prime number, where pn# is the product of the first n prime numbers. For example, for calculating the third fortunate number, first calculate the product of the first 3 prime numbers (2, 3, 5) i.e. 30. Upon adding 2 we get 32 which is an even number, adding 3 gives 33 which is a multiple of 3. One would similarly rule out integers up to 6. Adding 7 gives, 37 which is a prime number. Thus, ... Read More
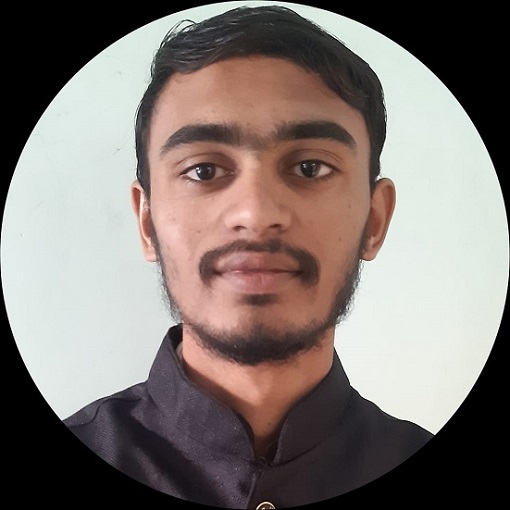
298 Views
The binary tree is a data structure. Each node of the binary tree contains either 0, 1, or 2 nodes. So, the binary tree can contain multiple levels. Here, we need to write the iterative code using the loops to find the height of the binary tree. The total number of levels in the binary tree represents the height of the binary tree. Alternatively, we can say that the maximum depth of the binary tree from the root node is the height of the binary tree. Problem statement − We have given a binary tree. We need to ... Read More