- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 7346 Articles for C++
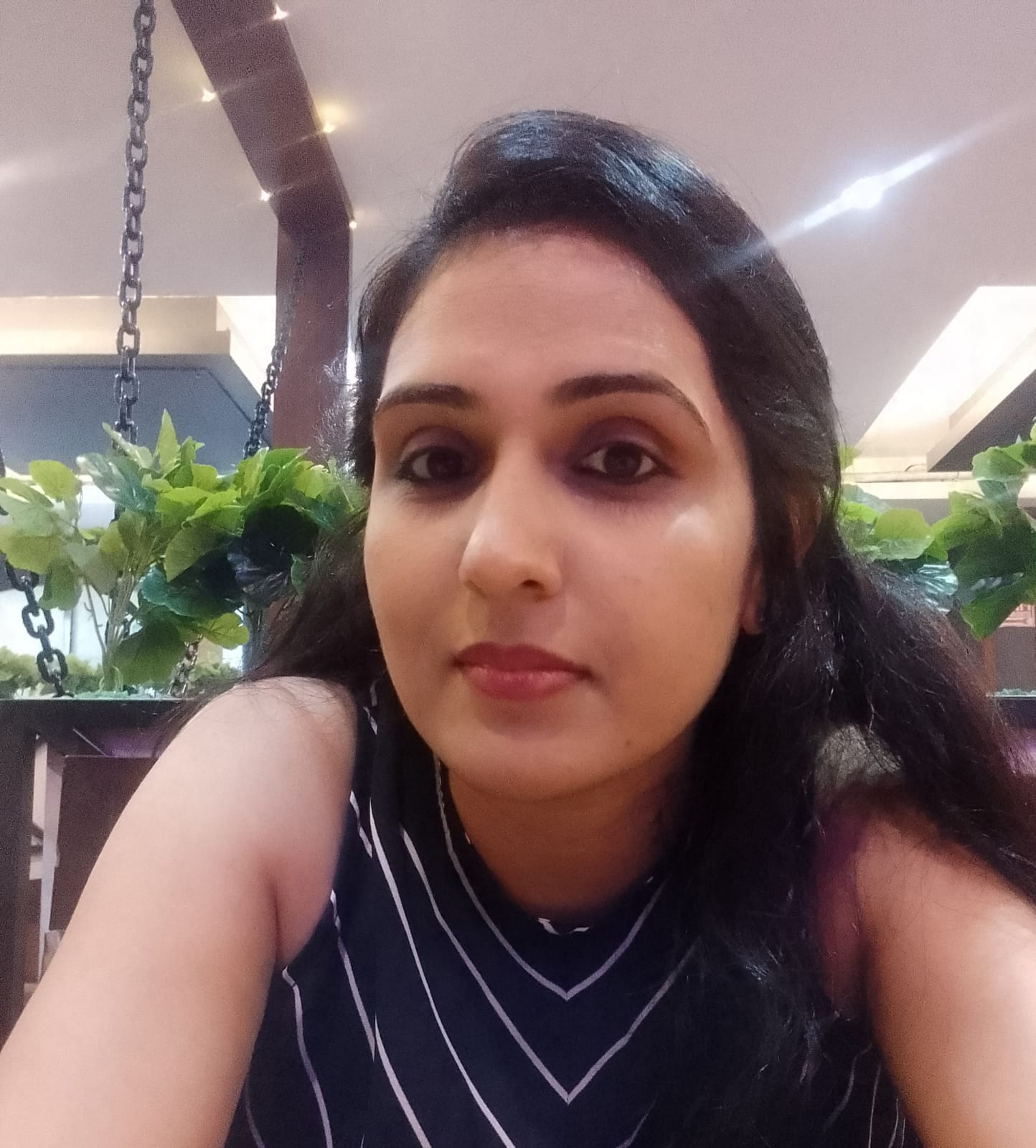
261 Views
Introduction In this tutorial, we implement two examples using C++ programming concepts to generate all permutations of an input string. Permutation of a string is the number of ways a string can be arranged by interchanging the position of characters. We also include some constraints or limitations. All permutations or arrangements of the input string ensure character B does not follow character A anywhere, meaning there is no AB combination in the string. To implement this task we use two approaches: Directly generate all combinations of the string while restricting AB. Using backtracking. Demonstration 1 String = ... Read More
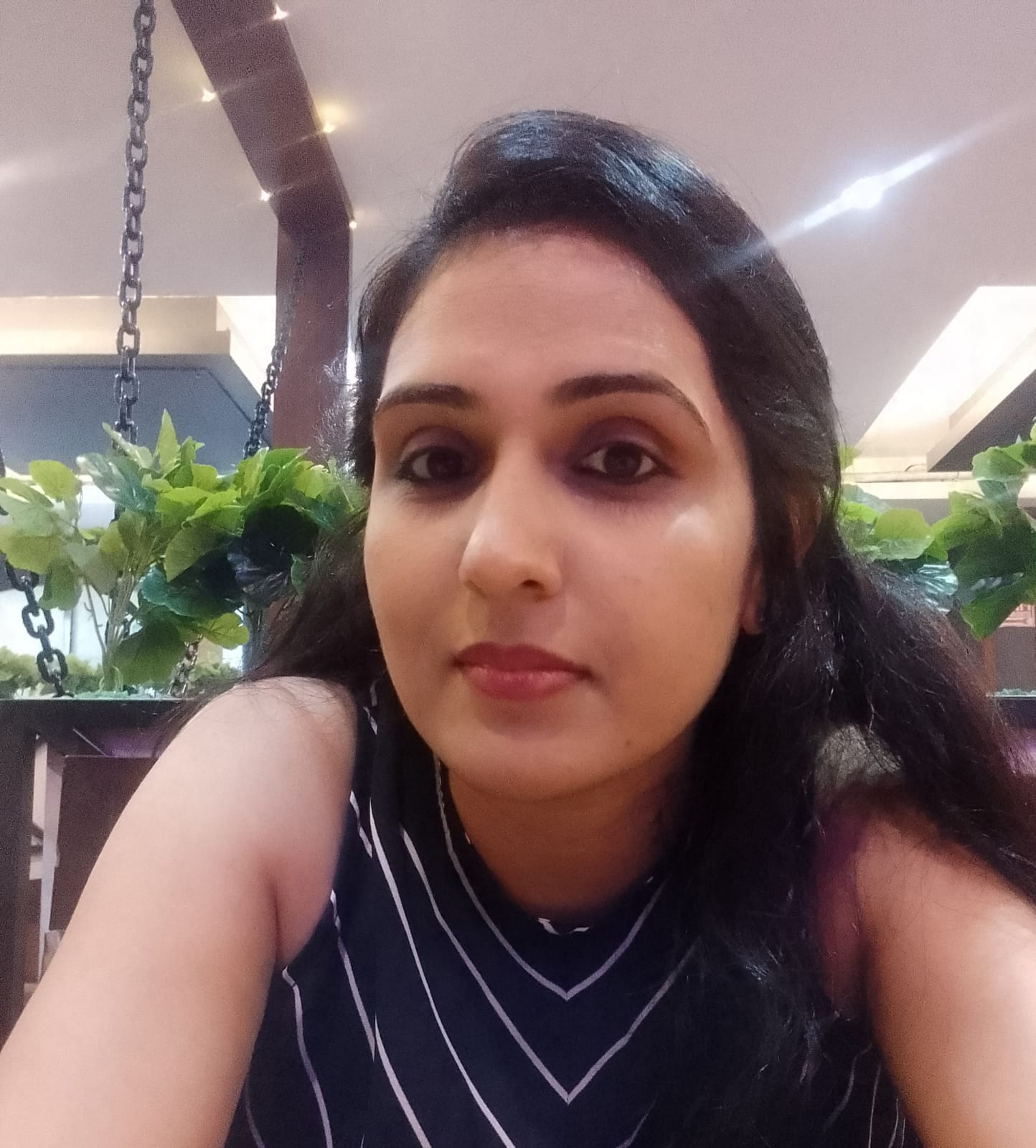
337 Views
Introduction In this tutorial, we implement an approach to capitalizing the first and last characters of each word in the input string. By iterating over the input string str, each word's starting and ending letters are capitalized. We implement this problem using C++ programming in two ways. Let's start this tutorial with some demonstrations. Demonstration 1 String = “coding world” Output CodinG WorlD In the above demonstration, consider the input string and the result after capitalizing the starting and ending character of each word of the string is CodinG WorlD. Demonstration 2 String = “`hello all” ... Read More

433 Views
The following article provides an in depth explanation of the method used to modify a number by toggling its first and last bit using bitwise operators. A bitwise operator is an operator that can be used to manipulate individual bits in binary numbers or bit patterns. Problem Statement For a given number n, modify the number such that the first and the last bit of the binary expansion of the new number are flipped i.e. if the original bit is 1 then the flipped bit should be 0 and vice versa. All the bits between the first and the last ... Read More

374 Views
Natural numbers are numbers that start from 1 and include all the positive integers. The following article discusses two possible approaches to compute the sum of the fifth powers of the first n natural numbers. The article discusses the two approaches in detail and compares them with regards to efficiency and intuitiveness. Problem Statement The purpose of this problem is to compute the arithmetic sum of the first n natural numbers, all raised to their fifth power i.e. $\mathrm{1^5 + 2^5 + 3^5 + 4^5 + 5^5 + … + n^5}$ till the nth term. Examples Since n is a ... Read More

265 Views
This article seeks a method for setting a given number's leftmost unset bit. The first unset bit after the most significant set bit is considered the leftmost unset bit. Problem Statement Given a number n, the task is to set the left most bit of the binary expansion of the number that is unset. All the other bits should be left unchanged. If all the bits of the original number are already set, return the number. Examples Input: 46 Output: 62 Explanation Binary Expansion of 46 = 101110. Left most unset bit is 101110. Upon setting the underlined ... Read More

109 Views
A number is considered to be pernicious if the number is a positive integer and the number of set bits in its binary expansion are prime. The first pernicious number is 3, as 3 = (11)2. It can be seen that the number of set bits in the binary representation of 3 are 2, which is a prime number. The first 10 pernicious numbers are 3, 5, 6, 7, 9, 10, 11, 12, 13, 14. Interestingly, powers of 2 can never be pernicious since they always have only 1 set bit. 1 is not a prime number. On the other ... Read More

189 Views
A number is considered to be an odious number if it has an odd number of 1s in its binary expansion. The first 10 odious numbers are 1, 2, 4, 7, 10, 11, 13, 14, 16, 19, 21. Interestingly, all powers of 2 are odious since they have only 1 set bit. The following article discusses 2 approaches in detail to find whether a number is an odious number or not. Problem Statement This problem aims to check whether the given number is an odious number i.e. it is a positive number with an odd number of set bits in ... Read More
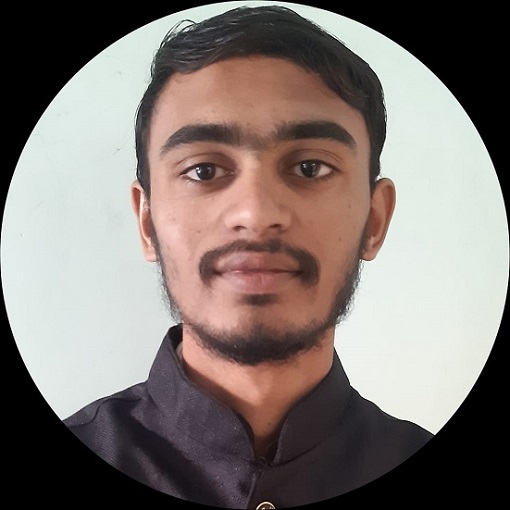
108 Views
In this problem, we need to find the total time required to complete all tasks according to the given condition. We can use the map data structure to solve the problem. We can keep tracking the last performed time for each task, and if the time interval is less than K, we can increment time units accordingly. Problem statement – We have given a string task containing alphabetical characters of length N. Each character represents a task, and we need one unit of time to perform the tasks. Also, the condition is that each task should be performed at ... Read More
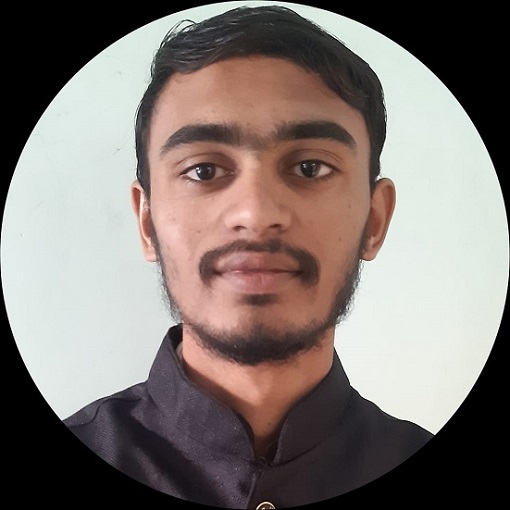
340 Views
We have given two strings and need to convert one string to another by click increments, and we can increment characters by either 1 or k in the single operation. To solve the problem, we need to make all characters of the first string the same as a second character by performing the cyclic increment operations. If the character at the same index in both strings is the same, we don’t need to perform any increment operation. Problem statement – We have given two strings named first and second containing the uppercase alphabetical characters. The length of both strings ... Read More
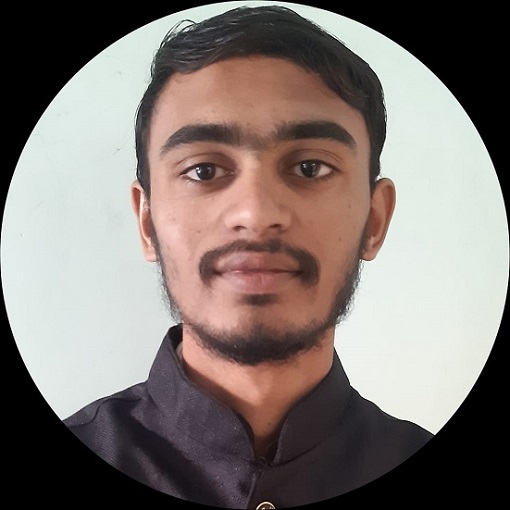
121 Views
In this problem, we need to find the maximum length of the subsequence containing the single character by increasing the multiple characters by, at most, k times. We can use the sliding window approach to solve the problem. We can find the maximum length of any window to get the result after sorting the string. Problem statement – We have given string str containing lowercase alphabetical characters. Also, we have given the positive integer k. After performing the at most k increment operations with multiple characters of the given string, we need to find the maximum length of the ... Read More