- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 7346 Articles for C++
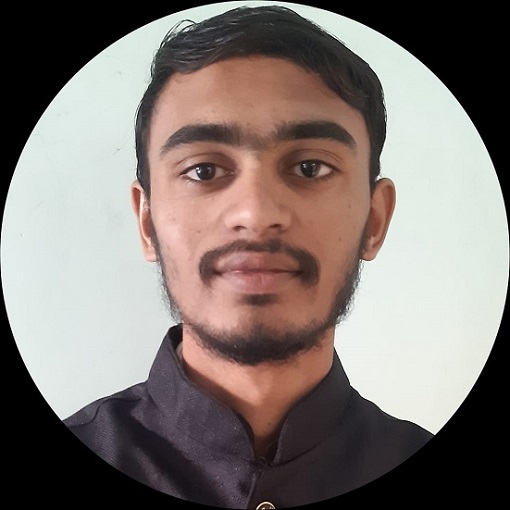
90 Views
In this problem, we will find the sum and product of the K maximum and minimum Fibonacci numbers in the array. The given problem is very basic and aims to focus on improving the problem-solving skills of beginners. The main goal of the problem is to introduce how to filter the Fibonacci numbers from the given array of elements and sum and product minimum and maximum Fibonacci numbers. Problem Statement We have given a nums[] array containing the N integer values. Also, we have given K positive integer. We need to find the sum and product of the K minimum ... Read More
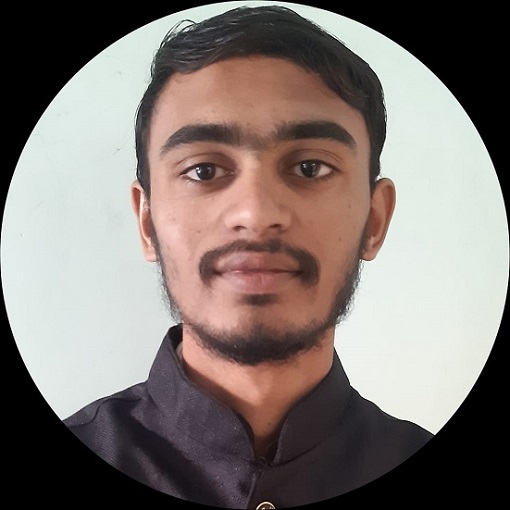
50 Views
In this problem, we will update the level of each child node based on the left and right subtree's weight difference. Here, we will recursively traverse the subtree of each node to get the weight of the left and right subtree. After that, we will again traverse each subtree node to update its level according to the difference in weight of the left and right sub-tree. Problem Statement We have given a complete binary tree containing N levels and 2N -1 nodes. The levels are numbered from 0 to N − 1 in decreasing order (0, -1, -2, -3, etc.). ... Read More
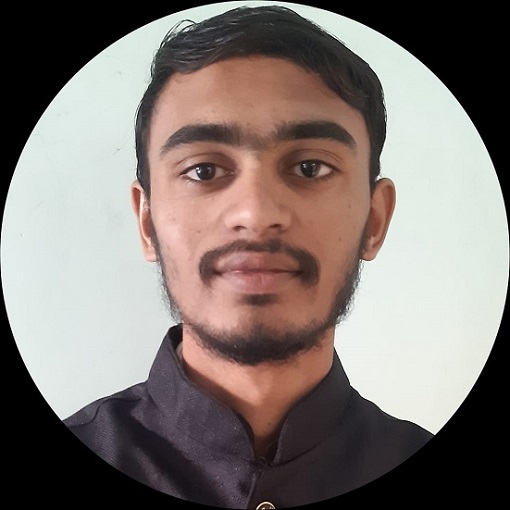
167 Views
In this problem, we will print the Nth Stepping number. The naïve approach for solving the problem is to traverse natural numbers, check whether each number is a Stepping number, and find the Nth Stepping number. Another approach can be using the queue data structure. Problem Statement We have given a positive integer N. We need to print the Nth Stepping number. The number is called a Stepping number if the difference between two adjacent digits of a number is 1. Sample Examples Input N = 15 Output 34 Explanation The Stepping numbers are 1, 2, ... Read More
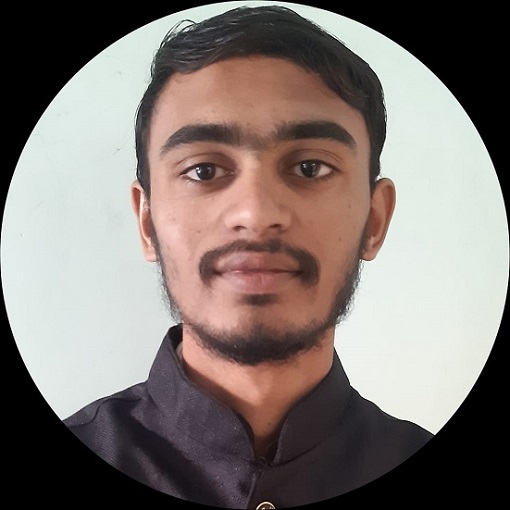
93 Views
In this problem, we will print all exponential levels of the binary tree. We will use the level order traversal to traverse through each level of the binary tree. After that, we will find minimum P and q values using the first element of the level. In the next step, we can check whether other level values are exponential. Problem Statement We have given a binary tree. We need to print values of all exponential levels of the binary tree. It is given if the values of each node of the level of the binary tree are equal ... Read More
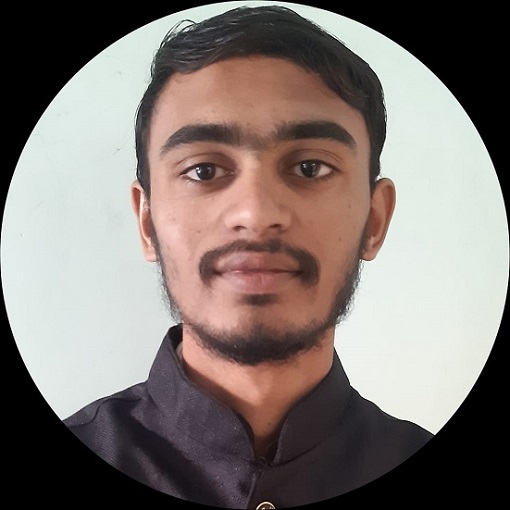
141 Views
In this problem, we will find the minimum difference between minimum and maximum array elements after multiplying or dividing any array elements with K. The simple approach to solving the problem is dividing each element of the array with K if divisible, multiplying each element with K, and tracking the array's minimum and maximum elements. Problem Statement We have given array nums[] containing the integer values and positive integer K. We can multiply any number of the nums[] array with K or divide it by K if it is divisible. The given task is to find the minimum difference between ... Read More
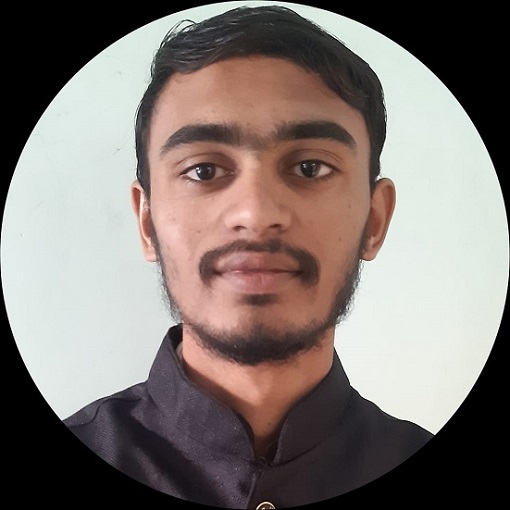
67 Views
In this problem, we will find the number of rotations required to achieve the target string from the string containing N 0s. Also, we will skip the strings given in the array while making rotations. We can use the BFS algorithm to find the minimum rotations required to get the target string. Problem Statement We have given a target string containing the N numeric characters. Also, we have given the strs[] array containing the M strings of size N containing the numeric characters. We need to initially achieve the target string from a string containing N 0's by performing the ... Read More
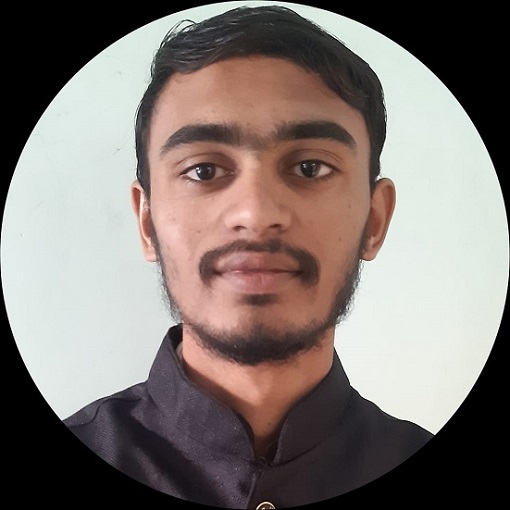
66 Views
In this problem, we will find the maximum number of cities to which we can supply water. We can consider this problem as a graph traversing the blocked node. So, we can use the breadth-first search algorithm to find the maximum number of connected cities. Problem Statement We have given the total N cities. Also, we have given an edge between two cities; all cities are connected with any other single or multiple cities. We need to set up the water supply connection in each city. We have also given the blocked[] array containing the 0 and 1 values. ... Read More
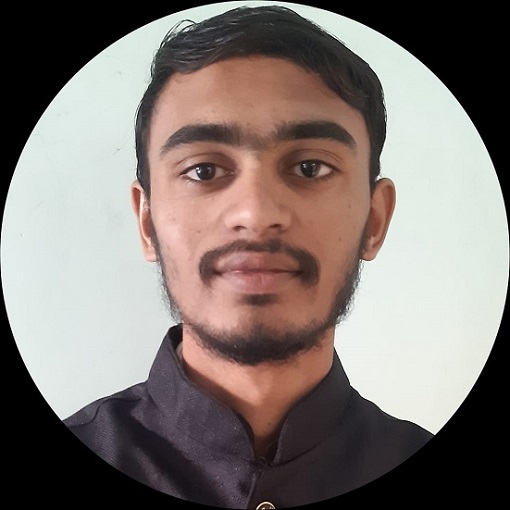
43 Views
In this problem, we will find the K points which are nearest to any of the given points from the points given in the array. To find the points which are nearest to the given points, we can take the nums[p] + 1 or nums[p] -1 for each element of the array if it is not present in the array. If we require more points, we can take the nums[p] + 2 or nums[p] – 2 points, and so on. Problem Statement We have given a nums[] array containing the N positive and negative integers. Each point of ... Read More
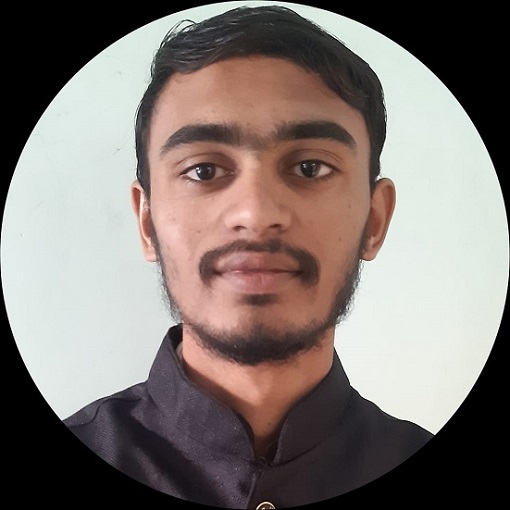
118 Views
In this problem, we will create a complete binary tree and traverse it in a circular clockwise direction. For the clockwise traversal, we can think of traversing the boundaries of the tree. For example, we can traverse the outer boundary of the tree first. After that, we can remove visited nodes and traverse the inner boundary of the tree. In this way, we need to make min(height/2, width/2) traversal of the given binary tree. Problem Statement We have given a complete binary tree containing N nodes and need to traverse it in a clockwise direction. Sample Examples Input n ... Read More
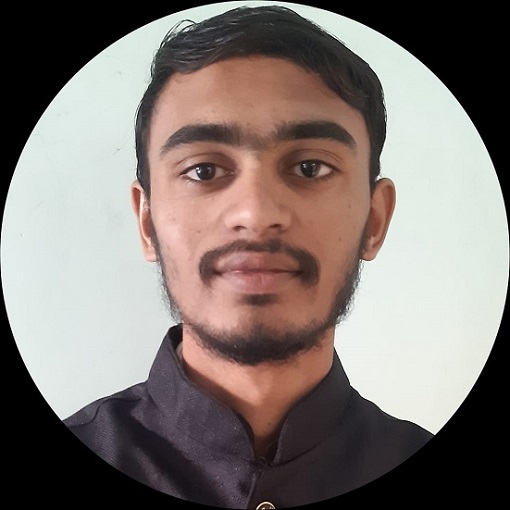
68 Views
In this problem, we will check whether the left view of the binary tree is sorted. The left view of the binary tree means nodes we can see when we look at the binary tree from the left side. In simple terms, we can see only the first node of each level. So, we need to extract the value of the first node and check whether they are sorted to get the output. Problem Statement We have given a binary tree. We need to print whether the binary tree's left view is sorted. If it is sorted, print 'Yes'. ... Read More