- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 7346 Articles for C++
Find Binary String of size at most 3N containing at least 2 given strings of size 2N as subsequences

56 Views
We are given three strings of equal size equal to 2*N, where N is an integer. We have to create a string of the size 3*N and at least two strings from the given strings be the subsequence of it. Also, the given strings are binary strings which means they only contain two different characters '0' and '1'. We will implement a code by traversing over the string and getting the frequency of the zeros and ones. Sample Examples Input string str1 = “11”; string str2 = “10”; string str3 = “10”; Output 110 ... Read More

81 Views
Set bits are the bits in the binary representation of the number which are '1'. The binary representation of the number contains only two digits '1' and '0', also it may be present in the form of a string. We are given a string, the binary representation of a given number, and an integer k. We have to get all the substrings of the length k from the given string and take the bitwise OR of all of them and at last, we have to return the number of the set bits present in the final string. Sample ... Read More

120 Views
In a string, a subsequence is a string that can be formed by deleting some character from it which means it contains some character from the string may be all or none and all will be present in the same order of the string. Among two strings we have to find the longest common subsequence that will not contain any repeating characters. Sample Examples Input string str1 = "aabcadjmuorrrcc" string str2 = "adbcwcadjomrorlc" Output The length of the longest common subsequence is: 8 Explanation: In the above-given strings, we have the largest ... Read More

48 Views
In the English language while writing a sentence we need to start with the capital character and for any name of the city/person, etc we begin with the capital letter. Here in this problem, we are given a string and a number and we have to update the first character of all the words of the given string if their size is not less than k. Also, if the size of the words is more than k and their first character is already capitalized then we will leave it as it is. Sample Examples Input string str ... Read More

118 Views
Splitting the array means we have to divide the array and make subsets. Here in this problem, we have given an array of integers with size n and integer k and our goal is to calculate the lowest cost by splitting the whole given array into the subsets of size k and adding the highest k/2 element of each subset into the cost. NOTE: here we consider a ceiling of k/2. Let’s see examples with explanations below to understand the problem in a better way. Sample Example Input n: 4 array: [ 3, 4, 2, 1 ... Read More
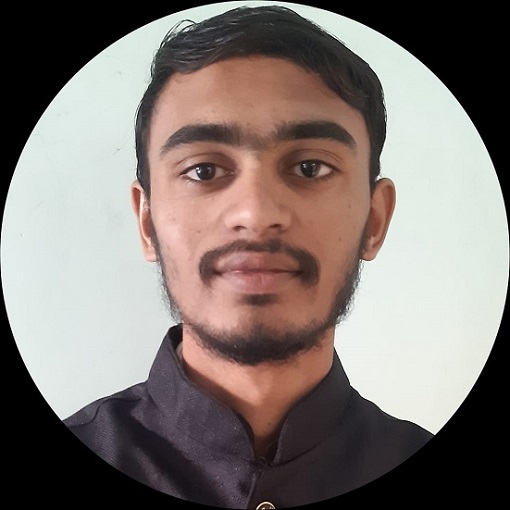
85 Views
In this problem, we will find the substring of the given string whose character's ASCII value's sum is maximum when we redefine the ASCII values. The naïve approach to solve the problem is to find the sum of all substring's character's ASCII value and get the substring having maximum sum. Another approach to solving the problem is using Kadane's algorithm to find the maximum sub-array sum. Problem statement - We have given a string alpha of size N containing the alphabetical characters. We have also given the chars[], and ASCII[] array of size M, where chars[] contains ... Read More
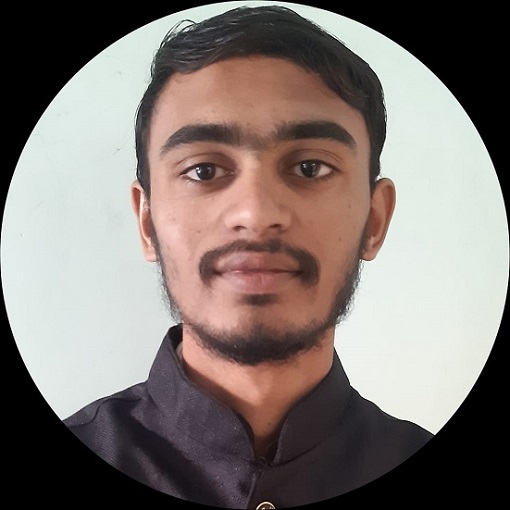
81 Views
In this problem, we need to split the parenthesis string into valid groups. When all opening brackets have related closing brackets, we can say that the group of parenthesis is valid. Problem Statement We have given a string containing open and closed parentheses. We need to split the string to get the maximum valid parenthesis string. Sample Examples Input: par = "(())()(()())" Output: (()), (), (()()), Explanation Each substring contains the valid parentheses sequence. Input: par = "()()" Output: (), () Explanation We have splited the string into two groups. Input: ... Read More
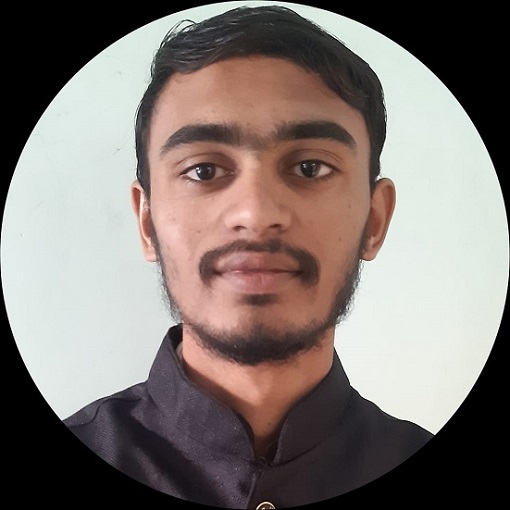
85 Views
In this problem, we will count the number of minimum operations required to convert string non-decreasing order by flipping the characters of the binary string. We can flip all characters of the substring starting from the \mathrm{p^{th}} index if the character at the pth index is 0 and not matching with character at the previous index, and we can count the minimum flips. Problem statement - We have given a binary string alpha. We need to count the minimum flips required to convert the binary string in increasing order. In one flip, we can select any index p ... Read More
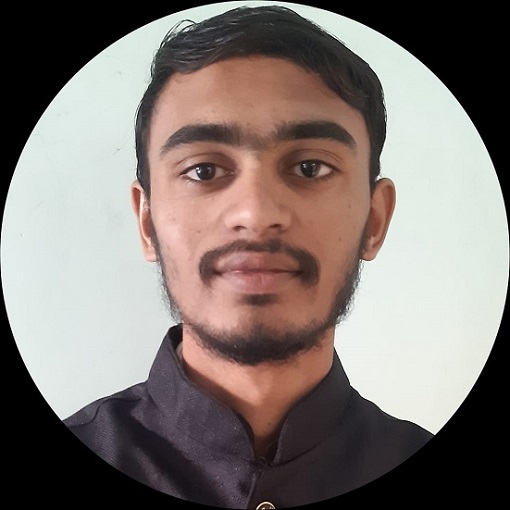
61 Views
In this problem, we will find the maximum points while converting the string S to T according to the conditions in the problem statement. We can traverse the string S and make each character of the string S same as string T's character at the same index by maximum swaps to get maximum points. In the other approach, we will prepare a mathematical formula based on the string's observation to get the answer. Problem statement - We have given a string S and T containing the alphabetical and numeric characters. We need to count the maximum ... Read More
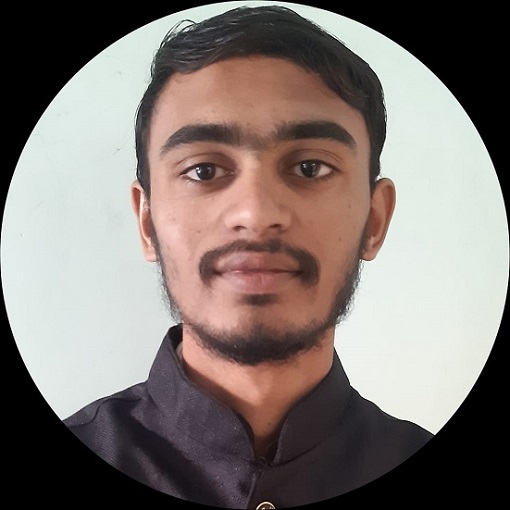
68 Views
In this problem, we need to find the maximum OR value of any two substrings of the given strings. The first approach is to find all substrings of the given binary string, take OR value of each string, and print the maximum OR value. The other approach is to take the original string as one substring and take another substring starting from left most zero to maximize the OR value. Problem statement - We have given a binary string alpha. We need to find the maximum OR value of any two substrings of the given binary ... Read More