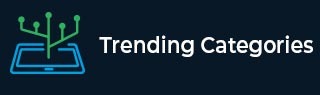
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
In this article, we will understand how to print alphabets from A to Z or a to z in Java. This is accomplished using a simple for loop.Below is a demonstration of the same −InputSuppose our input is −A to ZOutputThe desired output would be −A B C D E F G H I J K L M N O P Q R S T U V W X Y Z AlgorithmStep1- Start Step 2- Declare a character: my_temp Step 3- Run a for loop from A to Z and print the values using the increment operator Step 4- Display ... Read More
To count the number of consonants in a given sentence: Read a sentence from the user. Create a variable (count) initialize it with 0; Compare each character in the sentence with the characters {'a', 'e', 'i', 'o', 'u' } If match doesn't occurs increment the count. Finally print count.ExampleBelow is an example to count the number of consonants in a given sentence in Javaimport java.util.Scanner; public class CountingConsonants { public static void main(String args[]){ int count = 0; System.out.println("Enter a sentence :"); Scanner sc = new Scanner(System.in); String sentence = sc.nextLine(); for (int i=0 ; i
JavaScript Get href Value is a useful tool for web developers and designers who need to quickly access the value of an HTML element's href attribute. This article will provide step-by-step instructions on how to use JavaScript to get the value of an href attribute on a webpage, as well as some tips and tricks for getting the most out of this powerful feature. The ability to quickly obtain the values from an HTML element's attributes can help make development faster and more efficient, so let's get started! The value of the href attribute in JavaScript is a URL ... Read More
When we enter the coding world there are various mathematical operations that we learn to do through programming languages. We are expected to begin our coding journey with basic mathematical operations like adding, subtracting, multiplying and dividing. To evaluate the sum of two numbers, we use the '+' operator. However, Java also provides other approaches for adding two numbers. This article aims to explore the possible ways to add the two numbers in Java. To add the two numbers in Java, we are going to use the following approaches − By taking input of operands from user By ... Read More
To convert an integer to binary, use the Integer.toBinaryString() method in Java.Let’s say the following is the integer we want to convert.int val = 999;Converting the above integer to binary.Integer.toBinaryString(val)Example Live Demopublic class Demo { public static void main(String[] args) { int val = 999; // integer System.out.println("Integer: "+val); // binary System.out.println("Binary = " + Integer.toBinaryString(val)); } }OutputInteger: 999 Binary = 1111100111
Roots of a quadratic equation are determined by the following formula:$$x = \frac{-b\pm\sqrt[]{b^2-4ac}}{2a}$$Algorithm To calculate the rootsCalculate the determinant value (b*b)-(4*a*c).If determinant is greater than 0 roots are [-b +squareroot(determinant)]/2*a and [-b -squareroot(determinant)]/2*a.If determinant is equal to 0 root value is (-b+Math.sqrt(d))/(2*a)Example to find the roots of a quadratic equationBelow is an example to find the roots of a quadratic equation in Java programming.import java.util.Scanner; public class RootsOfQuadraticEquation { public static void main(String args[]){ double secondRoot = 0, firstRoot = 0; Scanner sc = new Scanner(System.in); System.out.println("Enter the value of ... Read More
To convert String to Boolean, use the parseBoolean() method in Java. The parseBoolean() parses the string argument as a boolean. The boolean returned represents the value true if the string argument is not null and is equal, ignoring case, to the string "true".Firstly, declare a string.String str = "false";Now, use the Boolean.parseBoolean() method to convert the above declared String to Boolean.boolean bool = Boolean.parseBoolean(str);Let us now see the complete example to learn how to convert String to Boolean.Example: Convert String to Boolean Live Demopublic class Demo { public static void main(String[] args) { // string ... Read More
In number theory, an Armstrong number is a pattern based number whose sum of each digit raised to the power of total number of digits is equal to the given number itself. Hence, to check whether a number is an Armstrong or not, first, determine the total number of digits and assume it 'n'. Then separate each digit and raise them to the power of 'n'. In the last step, calculate the power of each digit and add all of them. If the sum we get is equal to the original number, then it is an Armstrong number otherwise not. ... Read More
The * operator in Java is used to multiply two numbers. Read required numbers from the user using Scanner class and multiply these two integers using the * operator.Exampleimport java.util.Scanner; public class MultiplicationOfTwoNumbers { public static void main(String args[]){ Scanner sc = new Scanner(System.in); System.out.println("Enter the value of the first number ::"); int a = sc.nextInt(); System.out.println("Enter the value of the first number ::"); int b = sc.nextInt(); int result = a*b; System.out.println("Product of the given two numbers ... Read More
To count the occurrence of each character in a string using Hashmap, the Java code is as follows −Example Live Demoimport java.io.*; import java.util.*; public class Demo{ static void count_characters(String input_str){ HashMap my_map = new HashMap(); char[] str_array = input_str.toCharArray(); for (char c : str_array){ if (my_map.containsKey(c)){ my_map.put(c, my_map.get(c) + 1); }else{ my_map.put(c, 1); } } for (Map.Entry entry : my_map.entrySet()){ ... Read More