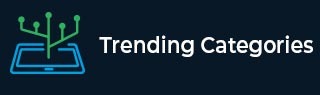
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Array Operations in Linux bash
Bash scripts are one of the most convenient approaches when we talk about making the command line processes automated in nature. They help us in doing a group of things in a simpler and more understandable manner, and we can do almost all the
operations that we could do in other languages as well.
It is well known that the syntax of the bash can be tricky at first, and hence I will try to explain everything that I have used in this tutorial.
We come across various situations where we would want to make use of bash scripts to perform different operations on arrays. In this tutorial, we will use different bash scripts to explain different operations that are possible on arrays.
How to Create and Execute a Bash File?
The first step when we want to work with a bash script is to create one. On Linux or macOS machines, we can create a bash file with the help of the following command.
touch mybashfile.sh
Notice that the name of the file can be anything you desire, but the extension of the file must be the same (i.e., bash).
Once you save the file, you can execute the same with the help of the following command −
bash mybashfile.sh
Now that we know how to create and run a bash script file, let's use it in an example.
Printing the First Element of an Array
In this example, we will learn how we can use a bash script to print the first element of an array. Consider the bash script shown below
#!/bin/bash myarray = (apple banana mango kiwi litchi watermelon) # printing the first element echo ${myarray[0]} echo ${myarray:0}
In the above bash script, we are creating an array named myarray which contains the names of different fruits inside it, and then we are making use of the echo command and different notations to print the first element inside the array.
Now to run and execute the above script, save it in a file. Let's assume that the name of the file is mybash.sh. Then we just need to execute the bash script, with the command shown below.
bash mybash.sh
Once we run the above command, it will produce the following output in the terminal −
apple apple
Printing all the Elements of an Array
In this example, we will learn how we can make use of a bash script to print all the elements of the array in it. Consider the bash script shown below.
#!/bin/bash myarray=( apple banana mango kiwi litchi watermelon ) # printing all array elements echo ${myarray[@]} echo ${myarray[@]:0}
In the above bash script, we are creating an array named myarray which contains the names of different fruits inside it, and then we are making use of the echo command and different notations to print all the elements that are present inside the array.
To run and execute the above script, save it in a file. Let's say that the name of the file is mybash.sh. Then we just need to execute the bash script, with the command shown below.
bash mybash.sh
Once we run the above command, it will produce the following output in the terminal −
apple banana mango kiwi litchi watermelon apple banana mango kiwi litchi watermelon
Printing Array Elements in a Certain Range
In this example, we will learn how we can use a bash script to print all the elements present in a certain range in an array. Consider the bash script shown below.
#!/bin/bash myarray = (apple banana mango kiwi litchi watermelon) # printing array elements in a certain range echo ${myarray[@]:1:3} echo ${myarray[@]:1:5}
In the above bash script, we are creating an array named myarray which contains the names of different fruits inside it, and then we are making use of the echo command and different notations to print all the elements that are present inside a range of the array.
Now to run and execute the above script, save it in a file. Let's assume that the name of the file is mybash.sh. Then we just need to execute the bash script, with the command shown below.
bash mybash.sh
Once we run the above command, it will produce the following output −
banana mango kiwi banana mango kiwi litchi watermelon
Printing the Number of Array Elements
In this example, we will learn how we can make use of a bash script to print the number of elements present in the array.
Consider the bash script shown below.
#!/bin/bash myarray = (apple banana mango kiwi litchi watermelon) # printing the number of elements in the array echo ${#myarray[*]} echo ${#myarray[@]}
In the above bash script, we are creating an array named myarray which contains the names of different fruits inside it, and then we are using the echo command and printing the length of the array using two different operators.
To run and execute the above script, save it in a file. Let's assume the name of the file is mybash.sh. Then we just need to execute the bash script, with the following command −
bash mybash.sh
Once we run the above command, the output we get in the terminal is shown below.
6 6
Conclusion
In this tutorial, you learned different basic operations that are possible on an array in Linux bash.