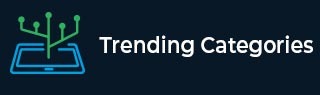
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Array index to balance sums in JavaScript
Problem
We are required to write a JavaScript function that takes in an array of integers, arr, as the first and the only argument.
Our function is required to pick and return one such index from the array such that the sum of elements on its left side is equal to the sum of elements on its right side. If there exists no such index in the array, we should return -1.
For example, if the input to the function is −
Input
const arr = [1, 2, 3, 4, 3, 2, 1];
Output
const output = 3;
Output Explanation
Because the sum of elements at either side of index 3 is equal (6).
Example
Following is the code −
const arr = [1, 2, 3, 4, 3, 2, 1]; const balancingIndex = (arr = []) => { const findSum = arr => arr.reduce((acc, x) => acc + x, 0); for(let i = 0; i < arr.length; i++){ const leftSum = findSum(arr.slice(0, i)); const rightSum = findSum(arr.slice(i + 1)); if(leftSum === rightSum){ return i; }; }; return -1; }; console.log(balancingIndex(arr));
Output
3
Advertisements