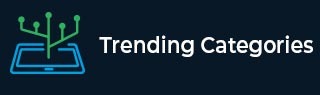
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Array.ConstrainedCopy() Method in C#
The Array.ConstrainedCopy() method in C# is used to copy a range of elements from an Array starting at the specified source index and pastes them to another Array starting at the specified destination index.
Syntax
public static void ConstrainedCopy (Array sourceArr, int sourceIndex, Array destinationArr, int destinationIndex, int length);
Here,
sourceArr − The Array that contains the data to copy.
sourceIndex − A 32-bit integer that represents the index in the sourceArr at which copying begins.
destinationArr − The Array that receives the data.
destinationIndex − A 32-bit integer that represents the index in the destinationArr at which storing begins.
len − A 32-bit integer that represents the number of elements to copy.
Let us now see an example to implement the Array.ConstrainedCopy() method −
Example
using System; public class Demo{ public static void Main(){ int[] arrDest = new int[10]; Console.WriteLine("Array elements..."); int[] arrSrc = { 20, 50, 100, 150, 200, 300, 400}; for (int i = 0; i < arrSrc.Length; i++){ Console.Write("{0} ", arrSrc[i]); } Console.WriteLine(); Array.ConstrainedCopy(arrSrc, 3, arrDest, 0, 4); Console.WriteLine("Destination Array: "); for (int i = 0; i < arrDest.Length; i++){ Console.Write("{0} ", arrDest[i]); } } }
Output
This will produce the following output −
Array elements... 20 50 100 150 200 300 400 Destination Array: 150 200 300 400 0 0 0 0 0 0
Advertisements