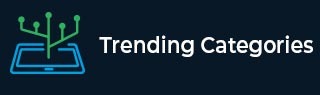
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Array.BinarySearch(Array, Int32, Int32, Object) Method with examples in C#
The Array.BinarySearch() method in C# is used to searches a range of elements in a one-dimensional sorted array for a value, using the IComparable interface implemented by each element of the array and by the specified value.
Note − It searches in a sorted array.
Syntax
The syntax is as follows −
public static int BinarySearch (Array arr, int index, int len, object val);
Above, the parameters arr is the 1-D array to search, index is the beginning index of the range to search, len is the length of the search. The val parameter is the object to search for.
Example
Let us now see an example −
using System; public class Demo { public static void Main() { int[] intArr = {10, 20, 30, 40, 50}; Array.Sort(intArr); Console.WriteLine("Array elements..."); foreach(int i in intArr) { Console.WriteLine(i); } Console.Write("Element 20 is at index = " + Array.BinarySearch(intArr, 1, 3, 20)); } }
Output
This will produce the following output −
Array elements... 10 20 30 40 50 Element 20 is at index = 1
Example
Let us now see another example −
using System; public class Demo { public static void Main() { int[] intArr = {5, 10, 15, 20}; Array.Sort(intArr); Console.WriteLine("Array elements..."); foreach(int i in intArr) { Console.WriteLine(i); } Console.Write("Element 25 is at index = " + Array.BinarySearch(intArr, 0, 2, 20)); } }
Output
This will produce the following output −
Array elements... 5 10 15 20 Element 25 is at index = -3
Advertisements