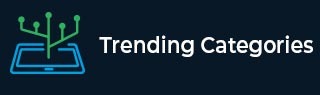
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Argument Parsing in Python
Every programming language has a feature to create scripts and run them from the terminal or being called by other programs. When running such scripts we often need to pass on arguments needed by the script for various functions to be executed inside the script. In this article we will see what are the various ways to pass arguments into a python script.
Using sys.argv
This is a inbuilt module sys.argv can process arguments that are passed on with the script. By default the first argument which is considered at sys.argv[0] is the file name. the rest of the arguments are indexed as 1,2 and so on. In the below example we see how a script uses the arguments passed onto it.
import sys print(sys.argv[0]) print("Hello ",sys.argv[1],", welcome!")
we take the below steps to run the above script and get the following result:
To run the above code we go to the terminal window and write the command shown below. Here the name of the script is args_demo.py. We pass an argument with value Samantha to this script.
D:\Pythons\py3projects>python3 args_demo.py Samantha args_demo.py Hello Samantha welcome!
Using getopt
This is another approach which has more flexibility as compared to the previous one. Here we can gather the value of the arguments and process them along with error handling and exceptions. In the below program we find the product of two numbers by taking in the arguments and skipping the first argument which is the file name. Of course the sys module is also used here.
Example
import getopt import sys # Remove the first argument( the filename) all_args = sys.argv[1:] prod = 1 try: # Gather the arguments opts, arg = getopt.getopt(all_args, 'x:y:') # Should have exactly two options if len(opts) != 2: print ('usage: args_demo.py -x <first_value> -b <second_value>') else: # Iterate over the options and values for opt, arg_val in opts: prod *= int(arg_val) print('Product of the two numbers is {}'.format(prod)) except getopt.GetoptError: print ('usage: args_demo.py -a <first_value> -b <second_value>') sys.exit(2)
Output
Running the above code gives us the following result −
D:\Pythons\py3projects >python3 args_demo.py -x 3 -y 12 Product of the two numbers is 36 # Next Run D:\Pythons\py3projects >python3 args_demo.py usage: args_demo.py -x <first_value> -b <second_value>
Using argparse
This is actually most preferred module to handle argument passing as the errors and exceptions are handled by the module itself without needing additional lines of code. We have to mention the name help text required for every argument we are going to use and then use the name of the argument in other parts of the code.
Example
import argparse # Construct an argument parser all_args = argparse.ArgumentParser() # Add arguments to the parser all_args.add_argument("-x", "--Value1", required=True, help="first Value") all_args.add_argument("-y", "--Value2", required=True, help="second Value") args = vars(all_args.parse_args()) # Find the product print("Product is {}".format(int(args['Value1']) * int(args['Value2'])))
Output
Running the above code gives us the following result −
D:\Pythons\py3projects>python3 args_demo.py -x 3 -y 21 Product is 63 # Next Run D:\Pythons\py3projects>python3 args_demo.py -x 3 -y usage: args_demo.py [-h] -x VALUE1 -y VALUE2 args_demo.py: error: argument -y/--Value2: expected one argument