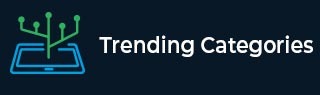
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Append all elements of other Collection to ArrayList in Java
The elements of a Collection can be appended at the end of the ArrayList using the method java.util.ArrayList.addAll(). This method takes a single parameter i.e. the Collection whose elements are added to the ArrayList.
A program that demonstrates this is given as follows −
Example
import java.util.ArrayList; import java.util.Vector; public class Demo { public static void main(String[] args) { ArrayList<String> aList = new ArrayList<String>(); aList.add("John"); aList.add("Sally"); aList.add("Harry"); aList.add("Martha"); aList.add("Susan"); Vector<String> vec = new Vector<String>(); vec.add("Peter"); vec.add("Michael"); vec.add("Jenna"); aList.addAll(vec); System.out.println("The ArrayList elements are: " + aList); } }
Output
The ArrayList elements are: [John, Sally, Harry, Martha, Susan, Peter, Michael, Jenna]
Now let us understand the above program.
First the ArrayList is defined and elements are added using the ArrayList.add() method. A code snippet which demonstrates this is as follows −
ArrayList<String> aList = new ArrayList<String>(); aList.add("John"); aList.add("Sally"); aList.add("Harry"); aList.add("Martha"); aList.add("Susan");
Then the vector is defined and elements are added. After that the ArrayList.addAll() method is used to append all the vector elements at the end of the arrayList. Then the ArrayList elements are displayed. A code snippet which demonstrates this is as follows −
Vector<String> vec = new Vector<String>(); vec.add("Peter"); vec.add("Michael"); vec.add("Jenna"); aList.addAll(vec); System.out.println("The ArrayList elements are: " + aList);
Advertisements