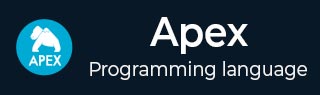
- Apex Programming Tutorial
- Apex - Home
- Apex - Overview
- Apex - Environment
- Apex - Example
- Apex - Data Types
- Apex - Variables
- Apex - Strings
- Apex - Arrays
- Apex - Constants
- Apex - Decision Making
- Apex - Loops
- Apex - Collections
- Apex - Classes
- Apex - Methods
- Apex - Objects
- Apex - Interfaces
- Apex - DML
- Apex - Database Methods
- Apex - SOSL
- Apex - SOQL
- Apex - Security
- Apex - Invoking
- Apex - Triggers
- Apex - Trigger Design Patterns
- Apex - Governer Limits
- Apex - Batch Processing
- Apex - Debugging
- Apex - Testing
- Apex - Deployment
- Apex Useful Resources
- Apex - Quick Guide
- Apex - Resources
- Apex - Discussion
Apex - if elseif else statement
An if statement can be followed by an optional else if...else statement, which is very useful to test various conditions using single if...else if statement.
Syntax
The syntax of an if...else if...else statement is as follows −
if boolean_expression_1 { /* Executes when the boolean expression 1 is true */ } else if boolean_expression_2 { /* Executes when the boolean expression 2 is true */ } else if boolean_expression_3 { /* Executes when the boolean expression 3 is true */ } else { /* Executes when the none of the above condition is true */ }
Example
Suppose, our Chemical company has customers of two categories – Premium and Normal. Based on the customer type we should provide them discount and other benefits like after sales service and support. Following program shows an implementation of the same.
//Execute this code in Developer Console and see the Output String customerName = 'Glenmarkone'; //premium customer Decimal discountRate = 0; Boolean premiumSupport = false; if (customerName == 'Glenmarkone') { discountRate = 0.1; //when condition is met this block will be executed premiumSupport = true; System.debug('Special Discount given as Customer is Premium'); }else if (customerName == 'Joe') { discountRate = 0.5; //when condition is met this block will be executed premiumSupport = false; System.debug('Special Discount not given as Customer is not Premium'); }else { discountRate = 0.05; //when condition is not met and customer is normal premiumSupport = false; System.debug('Special Discount not given as Customer is not Premium'); }
apex_decision_making.htm
Advertisements