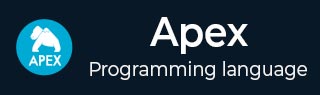
- Apex Programming Tutorial
- Apex - Home
- Apex - Overview
- Apex - Environment
- Apex - Example
- Apex - Data Types
- Apex - Variables
- Apex - Strings
- Apex - Arrays
- Apex - Constants
- Apex - Decision Making
- Apex - Loops
- Apex - Collections
- Apex - Classes
- Apex - Methods
- Apex - Objects
- Apex - Interfaces
- Apex - DML
- Apex - Database Methods
- Apex - SOSL
- Apex - SOQL
- Apex - Security
- Apex - Invoking
- Apex - Triggers
- Apex - Trigger Design Patterns
- Apex - Governer Limits
- Apex - Batch Processing
- Apex - Debugging
- Apex - Testing
- Apex - Deployment
- Apex Useful Resources
- Apex - Quick Guide
- Apex - Resources
- Apex - Discussion
Apex - For Loop
A for loop is a repetition control structure that allows you to efficiently write a loop that needs to execute a specific number of times. Consider a business case wherein, we are required to process or update the 100 records in one go. This is where the Loop syntax helps and makes work easier.
Syntax
for (variable : list_or_set) { code_block }
Flow Diagram
Example
Consider that we have an Invoice object which stores information of the daily invoices like CreatedDate, Status, etc. In this example, we will be fetching the invoices created today and have the status as Paid.
Note − Before executing this example, create at least one record in Invoice Object.
// Initializing the custom object records list to store the Invoice Records created today List<apex_invoice__c> PaidInvoiceNumberList = new List<apex_invoice__c>(); // SOQL query which will fetch the invoice records which has been created today PaidInvoiceNumberList = [SELECT Id,Name, APEX_Status__c FROM APEX_Invoice__c WHERE CreatedDate = today]; // List to store the Invoice Number of Paid invoices List<string> InvoiceNumberList = new List<string>(); // This loop will iterate on the List PaidInvoiceNumberList and will process each record for (APEX_Invoice__c objInvoice: PaidInvoiceNumberList) { // Condition to check the current record in context values if (objInvoice.APEX_Status__c == 'Paid') { // current record on which loop is iterating System.debug('Value of Current Record on which Loop is iterating is'+objInvoice); // if Status value is paid then it will the invoice number into List of String InvoiceNumberList.add(objInvoice.Name); } } System.debug('Value of InvoiceNumberList '+InvoiceNumberList);
apex_loops.htm
Advertisements