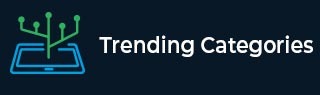
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
An Insertion Sort time complexity question in C++
What is the time complexity of insertion sort?
Time complexity is the amount of time taken by a set of codes or algorithms to process or run as a function of the amount of input.
For insertion sort, the time complexity is of the order O(n) i.e. big O of n in best case scenario. And in the average or worst case scenario the complexity is of the order O(n2).
What will be the time complexity of sorting when insertion sort algorithm is applied to n sized array of the following form: 6, 5, 8, 7, 10, 9 …… I, i-1
The time complexity of form sorting the above array is O(n). Let’s see this algorithm carefully, here all pairs are swapped from their original position i.e. positions of element 1 and element 2 are interchanged, 3 and 4 are interchanged and so on. So, while sorting the algorithm will take only one operation n times to sort this algorithm.
Define insertion sort and Write a code for insertion sort?
Insertion sort is a sorting algorithm that sorts the data structure by placing the element at its position in a sorted array.
The below code shows the function for insertion sort −
Example
void insertionSort(int arr[], int n) { for (int i = 1; i < n; i++){ int element = arr[i]; int j = i-1; while (j >= 0 && arr[j] > element){ arr[j+1] = arr[j]; j = j-1; } arr[j+1] = element; } }