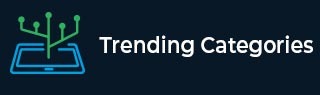
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
agent.createConnection() Method in Node.js
The agent.createConnection() method is an interface provided by the 'http' module. This method produces a socket/stream that can be used for the HTTP requests. One can use custom agents to override this method for greater flexibility. A socket/stream can be returned in two ways – either by returning the socket/stream directly from this function, or by passing this socket/stream to the callback.
Syntax
agent.createConnection(options, [callback])
Parameters
The above function can accept the following parameters −
options – These options will contain the connection details for which stream has to be created.
callback – This will receive the created socket connection from the agent.
Example
Create a file with the name – connection.js and copy the below code snippet. After creating the file, use the following command to run this code as shown in the example below −
node connection.js
connection.js
// Node.js program to demonstrate the creation of socket // using agent.createConnection() method // Importing the http module const http = require('http'); // Creating a new agent var agent = new http.Agent({}); // Creating connection with the above agent var conn = agent.createConnection; console.log('Connection is succesfully created !'); // Printing connection details console.log('Connection: ', conn);
Output
C:\home
ode>> node connection.js Connection is succesfully created ! Connection: function connect(...args) { var normalized = normalizeArgs(args); var options = normalized[0]; debug('createConnection', normalized); var socket = new Socket(options); if (options.timeout) { socket.setTimeout(options.timeout); } return socket.connect(normalized); }
Example
Let's take a look at one more example.
// Node.js program to demonstrate the creation of socket // using agent.createConnection() method // Importing the http module const http = require('http'); // Creating a new agent var agent = new http.Agent({}); // Defining options for agent const aliveAgent = new http.Agent({ keepAlive: true, maxSockets: 0, maxSockets: 5, }); // Creating connection with alive agent var aliveConnection = aliveAgent.createConnection; // Creating new connection var connection = agent.createConnection; // Printing the connection console.log('Succesfully created connection with agent: ', connection.toString); console.log('Succesfully created connection with alive agent: ', aliveConnection.toString);
Output
C:\home
ode>> node connection.js Succesfully created connection with agent: function toString() { [native code] } Succesfully created connection with alive agent: function toString() { [native code] }