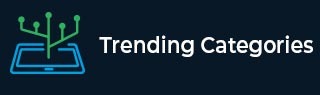
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Add Bold Tag in String in C++
Suppose we have a string s and a list of strings called dict, we have to add a closed pair of bold tag <b> and </b> to wrap the substrings in s that exist in that dict. When two such substrings overlap, then we have to wrap them together by only one pair of the closed bold tags. Also, if two substrings wrapped by bold tags are consecutive, we need to combine them.
So, if the input is like s = "abcxyz123" dict is ["abc","123"], then the output will be "<b>abc</b>xyz<b>123</b>"
To solve this, we will follow these steps −
n := size of s
Define an array bold of size n
ret := blank string
for initialize i := 0, end := 0, when i < size of s, update (increase i by 1), do −
for initialize j := 0, when j < size of dict, update (increase j by 1), do −
if substring of s from index (i to size of dict[j] - 1) is same as dict[j], then −
end := maximum of end and i + size of dict[j]
bold[i] := end > i
for initialize i := 0, when i < size of s, update i = j, do −
if bold[i] is zero, then −
ret := ret + s[i]
j := i + 1
Ignore following part, skip to the next iteration
j := i
while (j < size of s and bold[j] is non-zero), do −
(increase j by 1)
ret := substring of ret from index i to j - i - 1 concatenate "<b>" concatenate s
return ret
Example
Let us see the following implementation to get better understanding −
#include <bits/stdc++.h> using namespace std; class Solution { public: string addBoldTag(string s, vector<string>& dict) { int n = s.size(); vector<int> bold(n); string ret = ""; for (int i = 0, end = 0; i < s.size(); i++) { for (int j = 0; j < dict.size(); j++) { if (s.substr(i, dict[j].size()) == dict[j]) { end = max(end, i + (int)dict[j].size()); } } bold[i] = end > i; } int j; for (int i = 0; i < s.size(); i = j) { if (!bold[i]) { ret += s[i]; j = i + 1; continue; } j = i; while (j < s.size() && bold[j]) j++; ret += "<b>" + s.substr(i, j - i) + "</b>"; } return ret; } }; main(){ Solution ob; vector<string> v = {"abc","123"}; cout << (ob.addBoldTag("abcxyz123", v)); }
Input
"abcxyz123", ["abc","123"]
Output
<b>abc</b>xyz<b>123</b>