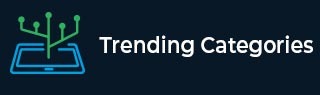
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Access property as a property using 'get' in JavaScript?
Property accessors offer dot notation or bracket notation-based access to an object's properties. Associative arrays are a good way to understand objects (a.k.a. map, dictionary, hash, lookup table). The names of the property names are the keys inside this array.
A difference between properties and methods is frequently made when discussing an object's properties. But the distinction between a property and a technique is simply an established practice. When a property's value is a reference to an example of a Function, for example, that property can be invoked to perform a function.
Syntax
ObjectName[propertyName]
You will study JavaScript getter and setter methods with the support of examples in this lesson.
There are two categories of object properties exist in JavaScript −
- Data properties
- Accessor properties
Accessor properties in JavaScript are ways to retrieve or modify an object's value. In order to do it, we use the following two keywords −
- Define a getter method called "get" to obtain the value of a property
- Define a setter method with the keyword “set” to set the property value
Example 1
To access an object's properties in JavaScript, use getter methods. For example −
The getter function getName() is defined in the code below to access an object's property. Additionally, we access the value as a property whenever accessing it. An error happens whenever you attempt to access the value as a method.
<!DOCTYPE html> <html> <title>Access property as a property using 'get' in JavaScript - TutorialsPoint</title> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> </head> <body style="text-align:center"> <script> const farm = { // this is data property fruitName: 'Pineapple', // this is accessor property(getter) get getName() { return this.fruitName; } }; // written to access data property document.write(farm.fruitName +'<br>'); // Pineapple // written to access getter methods document.write(farm.getName); // Pineapple // written trying to access as a method document.write(farm.getName()); // throws error </script> </body> </html>
Please press the f12 key on your keyboard to access the browser console to see the results.
TypeError: farm.getName is not a function
Example 2
JavaScript Setter. The values of an object can be changed in JavaScript by using setter methods. For example
The setter method is being used to modify an object's value in the example below. This set keyword is used to build setter methods. There should be one formal parameter in the setter only.
The value of fruitName in the programme below is Pineapple. Orange is then substituted as the value.
<!DOCTYPE html> <html> <title>Access property as a property using 'get' in JavaScript - TutorialsPoint</title> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> </head> <body style="text-align:center"> <script> const farm = { fruitName: 'Pineapple', //this is accessor property(setter) set changeName(newName) { this.fruitName = newName; } }; document.write(farm.fruitName +'<br>'); // Pineapple // this is change(set) object property using a setter farm.changeName = 'Orange '; document.write(farm.fruitName); // Orange </script> </body> </html>
Example 3
In this example let us understand how to create getters and setters in JavaScript, users can alternatively use the Object.defineProperty() method.
The method Object.defineProperty() is being used to access and modify an object's property in the example below.
There are three arguments given to the Object.defineProperty() function.
- The first argument is the objectName.
- The second argument is the name of the property.
- The third argument is an object that describes the property.
<!DOCTYPE html> <html> <title>Access property as a property using 'get' in JavaScript - TutorialsPoint</title> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> </head> <body style="text-align:center"> <script> const farm = { fruitName: 'Pineapple' } // written to get property Object.defineProperty(farm, "getName", { get : function () { return this.fruitName; } }); // written to setting property Object.defineProperty(farm, "changeName", { set : function (value) { this.fruitName = value; } }); document.write(farm.fruitName +'<br>'); // Pineapple // written to changing the property value farm.changeName = 'Orange '; document.write(farm.fruitName); // Orange </script> </body> </html>
Dot property accessor
The object's property is accessed in this using a dot property accessor; the property must have been a legal JavaScript identity.
Syntax
ObjectName.propertyName
Example 4
Using the dot property accessor is the earliest and most frequent way to access JavaScript properties of an object. Only accessing the stated object's valid IDs is possible with the help of this function.
<!DOCTYPE html> <html> <title>Access property as a property using 'get' in JavaScript - TutorialsPoint</title> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> </head> <body style="text-align:center"> <script> let object1={ studName : "Steve Jackson", studAge : 22, studAddress : "Delhi" }; let studName=object1.studName; document.write(studName); </script> </body> </html>
Using square brackets
Here the, a square bracket is used to retrieve the object's property. It works the same way as using the square bracket to access the items of an array.
Syntax
ObjectName[propertyName]
Example 5
Use the square property accessor to access any object properties that the dot property accessor does not allow you to access. It is primarily used to retrieve the properties and incorrect identifier of the array object.
<!DOCTYPE html> <html> <title>Access property as a property using 'get' in JavaScript - TutorialsPoint</title> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> </head> <body style="text-align:center"> <script> const school = { 'student-1': 'Bob Smith', '6': 'six' }; document.write(school['student-1'] +'<br>'); document.write(school[6]); </script> </body> </html>