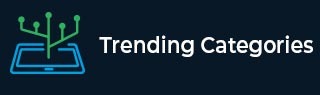
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
A Product Array Puzzle in C?
An array is a container of elements of the same data types. In product array Puzzle, the product of all elements is found.
In this Product Array Puzzle, we need to find the product of all elements of the array except element. The condition is that you cannot use the division operator and store this to another array.
For solving this we will be creating two products, one for all left elements and one for all right elements. And then adding this left and right products to get the desired products.
Example
#include<stdio.h> #include<stdlib.h> void productfind(int arr[], int n) { int *left = (int *)malloc(sizeof(int)*n); int *right = (int *)malloc(sizeof(int)*n); int *prod = (int *)malloc(sizeof(int)*n); int i, j; left[0] = 1; right[n-1] = 1; for(i = 1; i < n; i++) left[i] = arr[i-1]*left[i-1]; for(j = n-2; j >=0; j--) right[j] = arr[j+1]*right[j+1]; for (i = 0; i < n; i++) prod[i] = left[i] * right[i]; for (i = 0; i < n; i++) printf("%d ", prod[i]); return; } int main() { int arr[] = {10, 3, 5, 6, 2}; printf("The array is :
"); int n = sizeof(arr)/sizeof(arr[0]); for(int i = 0;i < n; i++){ printf("%d ",arr[i]); } printf("The product array is:
"); productfind(arr, n); }
Output
The array is : 10 3 5 6 2 The product array is: 180 600 360 300 900
Advertisements