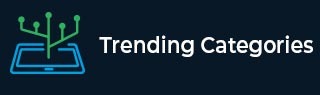
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
A modified game of Nim in C ?
Modified game of Nim is an optimisation games of arrays. This game predicts the winner based on the starting player and optimal moves.
Game Logic − In this game, we are given an array{}, that contains elements. There are generally two players that play the game namly player1 and player2. The aim of both is to make sure that all their numbers are removed from the array. Now, player1 has to remove all the numbers that are divisible by 3 and the player2 has to remove all the numbers that are divisible by 5. The aim is to make sure that they remove all elements optimally and find the winner in this case.
Sample
Array : {1,5, 75,2,65,7,25,6} Winner : playerB. A removes 75 -> B removes 5 -> A removes 6 -> B removes 65 -> No moves for A, B wins.
Code Preview
The code will find the number of elements that A can remove , number of elements that B can remove and the number of elements that they both can remove. Based on the number of the elements they both can remove the solution is found. As A removes first elements it can win even if he has to remove one element more than B. In normal case, the player with the maximum number of elements to remove wins.
PROGRAM TO FIND THE SOLUTION FOR GAME OF NIM
#include <bits/stdc++.h> using namespace std; int main() { int arr[] = {1,5, 75,2,65,7,25,6}; int n = sizeof(arr) / sizeof(arr[0]); int movesA = 0, movesB = 0, movesBoth = 0; for (int i = 0; i < n; i++) { if (arr[i] % 3 == 0 && arr[i] % 5 == 0) movesBoth++; else if (arr[i] % 3 == 0) movesA++; else if (arr[i] % 5 == 0) movesB++; } if (movesBoth == 0) { if (movesA > movesB) cout<<"Player 1 is the Winner"; cout<<"Player 2 is the Winner"; } if (movesA + 1 > movesB) cout<<"Player 1 is the Winner"; cout<<"Player 2 is the Winner"; ; return 0; }
Output
Player 2 is the Winner