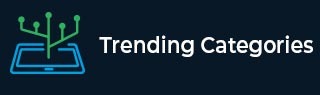
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Write down a python function to convert camel case to snake case?
Camel case and snake case are ways of writing words together when we are programming.
In camel case, we write words together without spaces and we start each new word with a capital letter except for the first word. For example, if we want to write a variable name for someone's date of birth, we can write it like this: dateOfBirth.
In snake case, we write words together with an underscore symbol between them, and all the letters are lowercase. For example, if we want to write a variable name for someone's home address, we can write it like this: home_address.
Both ways are useful because they make variable names easy to read and understand. It is important to choose one and use it consistently in our code to avoid confusion.
Example
Here is a simple function that converts a camel case string to a snake case string −
In this example, the function `camel_to_snake` takes a camel case string as input and returns a snake case string. The function loops through each character in the string and adds it to the new string, adding an underscore before each uppercase letter (except the first one) to create the snake case string.
def camel_to_snake(camel_case_string): snake_case_string = "" for i, c in enumerate(camel_case_string): if i == 0: snake_case_string += c.lower() elif c.isupper(): snake_case_string += "_" + c.lower() else: snake_case_string += c return snake_case_string camel_case_string = "camelCaseString" snake_case_string = camel_to_snake(camel_case_string) print("Camel case string:", camel_case_string) print("Snake case string:", snake_case_string)
Output
Camel case string: camelCaseString Snake case string: camel_case_string
Example
In this example, the function `camel_to_snake` uses the `re.sub()` method to replace any uppercase letter with an underscore followed by the same letter in lowercase. The `lower()` method is then called on the resulting string to convert it to lowercase.
Here is another function that converts a camel case string to a snake case string, using a regular expression −
import re def camel_to_snake(camel_case_string): snake_case_string = re.sub('([A-Z])', r'_\1', camel_case_string).lower() return snake_case_string camel_case_string = "camelCaseString" snake_case_string = camel_to_snake(camel_case_string) print("Camel case string:", camel_case_string) print("Snake case string:", snake_case_string)
Output
Camel case string: camelCaseString Snake case string: camel_case_string
Example
Here is one more function that converts a camel case string to a snake case string, using the `reduce()` function from the `functools` module −
In this example, the function `camel_to_snake` uses the `reduce()` function to iterate over each character in the input string and concatenate it with the previous character, adding an underscore before each uppercase letter (except the first one) to create the snake case string.
from functools import reduce def camel_to_snake(camel_case_string): return reduce(lambda x, y: x + ('_' if y.isupper() else '') + y.lower(), camel_case_string) camel_case_string = "camelCaseString" snake_case_string = camel_to_snake(camel_case_string) print("Camel case string:", camel_case_string) print("Snake case string:", snake_case_string)
Output
Camel case string: camelCaseString Snake case string: camel_case_string
Example
Here is another example that uses a for loop to iterate over each character in the input string, converting camel case to snake case −
def camel_to_snake_case(input_str): output_str = "" for char in input_str: if char.isupper(): output_str += "_" + char.lower() else: output_str += char return output_str # Example usage print(camel_to_snake_case("thisIsCamelCase")) # Output: this_is_camel_case
Output
this_is_camel_case
Example
Here is another example that uses a regular expression to match and replace camel case −
import re def camel_to_snake_case(input_str): output_str = re.sub('([a-z0-9])([A-Z])', r'\1_\2', input_str).lower() return output_str # Example usage print(camel_to_snake_case("thisIsCamelCase")) # Output: this_is_camel_case
Output
this_is_camel_case