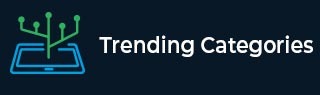
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Write a C# program to calculate a factorial using recursion
Factorial of a number is what we are finding using a recursive function checkFact () in the below example −
If the value is 1, it returns 1 since Factorial is 1 −
if (n == 1) return 1;
If not, then the recursive function will be called for the following iterations if you want the value of 5!
Interation1: 5 * checkFact (5 - 1); Interation2: 4 * checkFact (4 - 1); Interation3: 3 * checkFact (3 - 1); Interation4: 4 * checkFact (2 - 1);
To calculate a factorial using recursion, you can try to run the following code which shows what is done above −
Example
using System; namespace Demo { class Factorial { public int checkFact(int n) { if (n == 1) return 1; else return n * checkFact(n - 1); } static void Main(string[] args) { int value = 9; int ret; Factorial fact = new Factorial(); ret = fact.checkFact(value); Console.WriteLine("Value is : {0}", ret ); Console.ReadLine(); } } }
Output
Value is : 362880
Advertisements