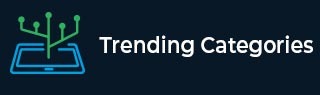
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What is the difference between Python's re.search and re.match?
In the vast realm of Python programming, regular expressions stand as essential tools for text processing and pattern matching, unlocking a plethora of possibilities for developers. Within Python's re module, two prominent functions, re.search() and re.match(), emerge as the veritable champions of pattern matching within strings. While both functions share the noble mission of pattern searching, they diverge in their behavior and method of execution. In this comprehensive and enlightening article, we embark on an illuminating journey, delving step-by-step into the intricacies of Python's re.search() and re.match() functions. With a few practical code examples and clear explanations, we aim to provide you with a profound understanding of the nuanced differences between these two powerful tools.
Understanding the Power of Regular Expressions in Python
Before we venture into the depths of re.search() and re.match(), it is imperative to grasp the true essence of regular expressions, often referred to as "regex," in the Python realm. These potent tools enable developers to engage in an intricate dance with strings, wielding the power to match and manipulate them based on specific patterns. With a repertoire of special characters and symbols, regular expressions define sets of strings, ushering in a new era of sophisticated text searches, replacements, and validations.
The power of re.search() Function
The re.search() function stands as a formidable ally, answering the call to seek a given pattern within a specified string. It embarks on its quest, scanning the entire input string, in search of the first occurrence of the specified pattern. When the required pattern unravels itself, re.search() presents the developer with a match object bearing the proof of the first successful encounter. Yet, in the event of an elusive pattern, re.search gives the output as None.
Using re.search()
Example
Consider a special function called "search_pattern_in_string." It works on a pattern made of special characters and a piece of text. This function "re.search()"helps it in this matter. It carefully looks through the text to find a specified pattern. In our case, the code looks for groups of one or more digits. When we try this function with a specific text, it reveals the pattern "100" in the text, and prints out that the pattern has been found.
import re def search_pattern_in_string(pattern, text): match = re.search(pattern, text) if match: print(f"Pattern '{pattern}' found in the text.") else: print(f"Pattern '{pattern}' not found in the text.") # Example usage pattern = r'\d+' text = "The price of the product is $100." search_pattern_in_string(pattern, text)
Output
Pattern '\d+' found in the text.
Using the re.match() Function
On the flip side, there's another powerful tool called "re.match()." It comes as a bit of a mystery, taking a unique approach to its working. This function only looks for the required pattern at the very beginning of the text. If the pattern is found there, it returns a match object. But if the pattern is not found at the beginning, re.match returns "None," indicating that the pattern is absent.
Example
Let us consider the match_pattern_at_start function that accepts a pattern and a piece of text as arguments. Empowered by re.match(), this function seeks the hidden pattern at the very beginning of the text. The special code 'r'\d+'' reveals the presence of one or more digits. As we invoke the function with a specific text, the pattern "100" is not found appearing at the start of the text, so it is printed that the pattern has not been found by the function.
import re def match_pattern_at_start(pattern, text): match = re.match(pattern, text) if match: print(f"Pattern '{pattern}' found at the start of the text.") else: print(f"Pattern '{pattern}' not found at the start of the text.") # Example usage pattern = r'\d+' text = "100 is the price of the product." match_pattern_at_start(pattern, text)
Output
Pattern '\d+' found at the start of the text.
Distinctions between re.search() and re.match()
Now that we have witnessed the working of re.search() and re.match() functions, let us endeavor to illumine the key distinctions between these two functions −
Search Scope
re.search() − Scours the entire input string in search of the pattern.
re.match() − Restricts its search to the beginning of the input string.
Position of Match
re.search() − finds the first occurrence of the pattern, regardless of its position.
re.match() − Permits a match only if the pattern is discovered at the sacred start of the input string.
Return Value
re.search() − returns a match object if the pattern is found; else, returns None.
re.match() − returns a match object if the pattern is found at the start; else, returns None.
Performance
re.search() − Undertakes a thorough search of the entire input string, potentially affecting performance for extensive strings.
re.match() − Exhibits efficiency by focusing on matching the pattern only at the sacred beginning of the string.
Harnessing re.search() for Multiple Matches
An additional facet that sets re.search() apart is its response to multiple matches within the input string. As the function finds the first occurrence, it diverges from re.match(), which solely seeks the pattern at the very start.
Let us now learn how re.search() handles multiple matches −
Example
Let's welcome the search_multiple_matches function, which takes a special code called a regular expression pattern and a text as input variables. By using re.search(), this function begins its important task, searching for the hidden pattern within the given text. The pattern 'r'\d+'' represents one or more digits. When we use this function with a specific text, the magic happens - it finds the pattern "100" at index 19 in the text.
import re def search_multiple_matches(pattern, text): matches = re.search(pattern, text) if matches: print(f"Pattern '{pattern}' found at index {matches.start()}.") # Example usage pattern = r'\d+' text = "The product costs $100. The discounted price is $50." search_multiple_matches(pattern, text)
Output
Pattern '\d+' found at index 19.
Using re.match() for Multiple Matches
Let us now venture into the realm of re.match(), as it unveils its response to multiple matches while searching for the pattern at the very start.
Example
Observe the match_multiple_matches_at_start function, which takes a regex pattern and a text string as arguments. Empowered by re.match(), this function embarks on a mission, seeking the required pattern at the start of the text. The pattern 'r'\d+'' represents one or more digits. As we invoke the function with the example text, - the pattern "100" remains elusive and it is printed that the pattern is not found.
import re def match_multiple_matches_at_start(pattern, text): matches = re.match(pattern, text) if matches: print(f"Pattern '{pattern}' found at index {matches.start()}.") # Example usage pattern = r'\d+' text = "100 is the product code. 200 is the order code." match_multiple_matches_at_start(pattern, text)
Output
Pattern '\d+' found at index 0.
Empowering re.search() with Flags
Additional functionality of re.search() lies in its ability to invoke flags, granting the power of case-insensitive matching, multi-line searching, and other options. Let us illuminate this aspect with an example featuring the case-insensitive flag −
Example
Let us consider the case_insensitive_search function, taking regular expression patterns and the text string as arguments. Empowered by re.search(), this function embarks on a mission of searching for a specified pattern while invoking the case-insensitive flag. The pattern 'r'text'' matches the word "text." As we call the function with the given example text, it will find the pattern "TEXT" by using the case-insensitive flag, and print that the pattern has been found.
import re def case_insensitive_search(pattern, text): matches = re.search(pattern, text, re.IGNORECASE) if matches: print(f"Pattern '{pattern}' found in the text.") # Example usage pattern = r'text' text = "This is a TEXT example." case_insensitive_search(pattern, text)
Output
Pattern 'text' found in the text.
In the comparison of re.search() and re.match(), we have witnessed the utility of two distinct yet interconnected functions, each blessed with its unique functionalities. Understanding their nuances is essential for navigating the realm of text processing with finesse and proficiency. As we incorporate the knowledge of re.search() and re.match() into our Python repertoire, we are equipped to tackle various text processing challenges, be it finding the first occurrence, matching at the start, or embracing case-insensitive searches. Python's regular expressions offer a mighty toolkit for handling complex text matching and manipulation tasks. With these potent tools at our disposal, we can stride forward with confidence, unraveling the boundless wonders of text processing in the realm of Python.