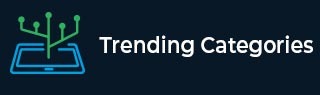
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What does the method sort(int[] a, int fromIndex, int toIndex) do in java?
The sort(int[] a, int fromIndex, int toIndex) method of the java.util.Arrays class sorts the specified range of the specified array of integer value into ascending numerical order. The range to be sorted extends from index fromIndex, inclusive, to index toIndex, exclusive.
Example
import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { int iArr[] = {3, 1, 2, 18, 10}; for (int number : iArr) { System.out.println("Number = " + number); } Arrays.sort(iArr, 0, 3); System.out.println("int array with some sorted values(0 to 3) is:"); for (int number : iArr) { System.out.println("Number = " + number); } } }
Output
Number = 3 Number = 1 Number = 2 Number = 18 Number = 10 int array with some sorted values(0 to 3) is: Number = 1 Number = 2 Number = 3 Number = 18 Number = 10
Advertisements