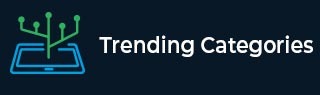
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What does the method clear() do in java?
The clear() method of the class java.util.ArrayList removes all of the elements from this list. The list will be empty after this call returns.
Example
import java.util.ArrayList; public class ArrayListDemo { public static void main(String[] args) { ArrayList<Integer> arrlist = new ArrayList<Integer>(5); arrlist.add(20); arrlist.add(30); arrlist.add(10); arrlist.add(50); for (Integer number : arrlist) { System.out.println("Number = " + number); } int retval = arrlist.size(); System.out.println("List consists of "+ retval +" elements"); System.out.println("Performing clear operation !!"); arrlist.clear(); retval = arrlist.size(); System.out.println("Now, list consists of "+ retval +" elements"); } }
Output
Number = 20 Number = 30 Number = 10 Number = 50 List consists of 4 elements Performing clear operation !! Now, list consists of 0 elements
Advertisements