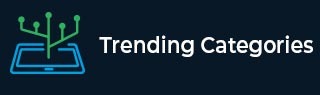
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What are static members of a Java class?
In Java, static members are those which belongs to the class and you can access these members without instantiating the class.
The static keyword can be used with methods, fields, classes (inner/nested), blocks.
Static Methods − You can create a static method by using the keyword static. Static methods can access only static fields, methods. To access static methods there is no need to instantiate the class, you can do it just using the class name as −
Example
public class MyClass { public static void sample(){ System.out.println("Hello"); } public static void main(String args[]){ MyClass.sample(); } }
Output
Hello
Static Fields − You can create a static field by using the keyword static. The static fields have the same value in all the instances of the class. These are created and initialized when the class is loaded for the first time. Just like static methods you can access static fields using the class name (without instantiation).
Example
public class MyClass { public static int data = 20; public static void main(String args[]){ System.out.println(MyClass.data); } Java Arrays with Answers 27 }
Output
20
Static Blocks − These are a block of codes with a static keyword. In general, these are used to initialize the static members. JVM executes static blocks before the main method at the time of class loading.
Example
public class MyClass { static{ System.out.println("Hello this is a static block"); } public static void main(String args[]){ System.out.println("This is main method"); } }
Output
Hello this is a static block This is main method