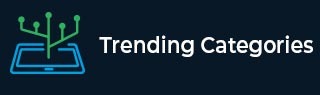
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What are required arguments of a function in python?
Functions accept arguments that can contain data. The function name is followed by parenthesis that list the arguments. Simply separate each argument with a comma to add as many as you like.
As the name implies, mandatory arguments are those that must be given to the function at the time of the function call. Failure to do so will lead to a mistake. Simply put, default function parameters are the exact opposite of required arguments. As we previously saw, while declaring the function, we give the function parameters a default value in the case of default arguments. The function automatically uses the default argument value for these arguments if no argument is provided when calling it. Consequently, it was not necessary to provide a value for default arguments.
It is necessary to give an argument when calling a function for parameters for which there is no default argument, though. Otherwise, the Python interpreter throws a warning that a positional argument is missing. The fact that required arguments lack a default value makes it simple to distinguish them from default arguments.
Example
Let’s look at an example of a function taking arguments. In the following code, a function takes different number of arguments.
def add_nums(num1, num2=12): print(num1 + num2) add_nums(num1=11, num2=13) # Output: 24 # no value for default argument add_nums(num1=11) # Output: 23 # no value for required argument add_nums(num2=13) # Will throw an error
Output
The output generated is as follows. As expected, the third function call throws an error.
24 23 Traceback (most recent call last): File "main.py", line 7, inadd_nums(num2=13) # Will throw an error TypeError: add_nums() missing 1 required positional argument: 'num1'