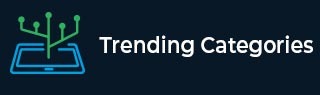
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What are different methods of passing parameters in C#?
When a method with parameters is called, you need to pass the parameters to the method using any of the following three methods -
Reference Parameters
This method copies the reference to the memory location of an argument into the formal parameter. This means that changes made to the parameter affect the argument.
Value Parameters
This method copies the actual value of an argument into the formal parameter of the function. In this case, changes made to the parameter inside the function have no effect on the argument.
In Value parameters, when a method is called, a new storage location is created for each value parameter. The values of the actual parameters are copied into them. Hence, the changes made to the parameter inside the method have no effect on the argument.
Let us see an example for Value Parameters in C# −
Example
using System; namespace CalculatorApplication { class NumberManipulator { public void swap(int x, int y) { int temp; temp = x; /* save the value of x */ x = y; /* put y into x */ y = temp; /* put temp into y */ } static void Main(string[] args) { NumberManipulator n = new NumberManipulator(); /* local variable definition */ int a = 7; int b = 10; Console.WriteLine("Before swap, value of a : {0}", a); Console.WriteLine("Before swap, value of b : {0}", b); /* calling a function to swap the values */ n.swap(a, b); Console.WriteLine("After swap, value of a : {0}", a); Console.WriteLine("After swap, value of b : {0}", b); Console.ReadLine(); } } }
Output
Before swap, value of a : 7 Before swap, value of b : 10 After swap, value of a : 7 After swap, value of b : 10