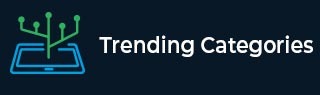
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Thread-based parallelism in C#
In C#, Task parallelism divide tasks. The tasks are then allocated to separate threads for processing. In .NET, you have the following mechanisms to run code in parallel: Thread, ThreadPool, and Task. For parallelism, use tasks in C# instead of Threads.
A task will not create its own OS thread, whereas they are executed by a TaskScheduler.
Let us see how to create tasks. Use a delegate to start a task −
Task tsk = new Task(delegate { PrintMessage(); }); tsk.Start();
Use Task Factory to start a task −
Task.Factory.StartNew(() => {Console.WriteLine("Welcome!"); });
You can also use Lambda −
Task tsk = new Task( () => PrintMessage() ); tsk.Start();
The most basic way to start a task is using the run() −
Example
using System; using System.Threading.Tasks; public class Example { public static void Main() { Task tsk = Task.Run(() => { int a = 0; for (a = 0; a <= 1000; a++) {} Console.WriteLine("{0} loop iterations ends", a); }); tsk.Wait(); } }
Output
1001 loop iterations ends
Advertisements