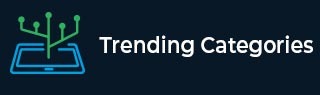
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
The Floyd-Warshall algorithm in Javascript
Djikstra's algorithm is used to find distance/path of the shortest path from one node to all other nodes. There are cases where we need to find shortest paths from all nodes to all other nodes. This is where the All pairs shortest path algorithms come in handy. The most used all pairs shortest path algorithm is Floyd Warshall algorithm.
Floyd Warshall algorithm works as follows −
- We initialize an N x N matrix of distances to be Infinity.
- Then for each edge u, v, we update this matrix to be showing the weight of this edge and for edges v, v we update the weight to be 0.
- We create 3 nested loops with iterators I, j and k. for every node I's distance to every node j, we consider using k as intermediate points and update the distance if we find a less than existing arr[i][j].
Instead of using a matrix, we'll use an object as we don't need to keep track of index in case we are using complex objects to represent each node.
Now let us look at the implementation of the same −
Example
floydWarshallAlgorithm() { let dist = {}; for (let i = 0; i < this.nodes.length; i++) { dist[this.nodes[i]] = {}; // For existing edges assign the dist to be same as weight this.edges[this.nodes[i]].forEach(e => (dist[this.nodes[i]][e.node] = e.weight)); this.nodes.forEach(n => { // For all other nodes assign it to infinity if (dist[this.nodes[i]][n] == undefined) dist[this.nodes[i]][n] = Infinity; // For self edge assign dist to be 0 if (this.nodes[i] === n) dist[this.nodes[i]][n] = 0; }); } this.nodes.forEach(i => { this.nodes.forEach(j => { this.nodes.forEach(k => { // Check if going from i to k then from k to j is better // than directly going from i to j. If yes then update // i to j value to the new value if (dist[i][k] + dist[k][j] < dist[i][j]) dist[i][j] = dist[i][k] + dist[k][j]; }); }); }); return dist; } }
You can test this using −
Example
let g = new Graph(); g.addNode("A"); g.addNode("B"); g.addNode("C"); g.addNode("D"); g.addEdge("A", "C", 100); g.addEdge("A", "B", 3); g.addEdge("A", "D", 4); g.addEdge("D", "C", 3); console.log(g.floydWarshallAlgorithm());
Output
This will give the output −
{ A: { C: 7, B: 3, D: 4, A: 0 }, B: { A: 3, B: 0, C: 10, D: 7 }, C: { A: 7, D: 3, B: 10, C: 0 }, D: { A: 4, C: 3, B: 7, D: 0 } }
Advertisements