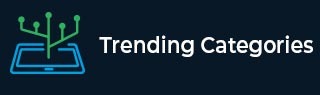
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Removing a node in a Javascript Tree
Tree is a nonlinear data structure which has nodes and edges; where edges act as link between two nodes and nodes hold values in it.
Traversal is a procedure where it will retrieve the data in every node of the tree and print them in a specified sequential order.
There are three types of tree traversals which are mentioned below −
In-order traversal − In-order technique will follow the path (Left → Root → Right).
Pre-order traversal − Pre-order technique will follow the path (Root → Left → Right).
Post-order traversal − Post-order technique will follow the path (Left → Right → Root).
Removal of Nodes from a tree
The term removal of node from the tree is basically a procedure where a node is deleted and forms a new shape. But whereas in some other cases it eliminates it’s link between them and replaces with some other node in the tree.
Usually there won’t be any tree kind of structure in memory (storage). They are randomly placed elements (Nodes) in the memory in which we are trying to connect them with the help of pointers (edges).
From that if we need to remove an element, need to detach the pointer attached to it and assign it to the appropriate element.
Consider this example below −
In the above case we’ve tried to remove a node (40) which has one child node attached to it. If a node to be deleted is having a single child, it will copy the child to the node and delete the child.
A node can be removed from a tree. In which there are three possible cases to delete a node. The following cases are mentioned below.
Scenario 1 − If the node to be deleted is leaf node it can be simply removed from the tree in this case.
Algorithm
If the node is a leaf node
- Remove the node from the tree.
Scenario 2 − If the node to be deleted has only one child it will copy its child to the node and delete the child.
Algorithm
If the node has one child
- Find it’s child node, Assume c_node.
- Make it node → data = c_node.
- Again delete the child node..
Scenario 3 − If the node to be deleted has Two child.
Firstly, find the in-order successor of the node and copy its value to the node to be removed and delete the in-order successor node.
Algorithm
If the node has both left and right child
- Find the smallest node in the right subtree, Assume min_node.
- Make it node → data = min_node.
- Again delete the min_node.
Example
In this below example we have implemented a binary search tree and inserted enough number of nodes to it. We’ve performed the deletion operations on the given tree. And we have also performed in-order traversal after removing the selected nodes from the tree.
<!DOCTYPE html> <html> <head> <script> class Node { constructor(item) { this.key = item; this.left = this.right = null; } } let root = null; function deleteKey(key) { root = deleteRecursive(root, key); } function deleteRecursive(root, key) { if (root == null) return root; if (key < root.key) root.left = deleteRecursive(root.left, key); else if (key > root.key) root.right = deleteRecursive(root.right, key); else { if (root.left == null) return root.right; else if (root.right == null) return root.left; root.key = minimumValue(root.right); root.right = deleteRecursive(root.right, root.key); } return root; } function minimumValue(root) { let minValue = root.key; while (root.left != null) { minValue = root.left.key; root = root.left; } return minValue; } function insert(key) { root = insertRec(root, key); } function insertRec(root, key) { if (root == null) { root = new Node(key); return root; } if (key < root.key) root.left = insertRec(root.left, key); else if (key > root.key) root.right = insertRec(root.right, key); return root; } function inorder() { InorderRecursion(root); } function InorderRecursion(root) { if (root != null) { InorderRecursion(root.left); document.write(root.key + " "); InorderRecursion(root.right); } } insert(90); insert(40); insert(10); insert(50); insert(100); insert(70); insert(60); insert(80); insert(120); document.write("After inserting the above nodes In-order will be: <br>"); inorder(); document.write("<br>Delete the node 10<br><br>"); deleteKey(10); document.write("In-order after node 10 is deleted from the tree <br>"); inorder(); document.write("<br>Delete the node 40<br><br>"); deleteKey(40); document.write("In-order after node 40 is deleted from the tree <br>"); inorder(); document.write("<br>Delete the node 90<br><br>"); deleteKey(90); document.write("After removing the above nodes, the In-order will be as follows: <br>"); inorder(); </script> </head> <body> </body> </html>
Output
The output of the above script will be −
After inserting the above nodes In-order will be: 10 40 50 60 70 80 90 100 120 Delete the node 10 In-order after node 10 is deleted from the tree 40 50 60 70 80 90 100 120 Delete the node 40 In-order after node 40 is deleted from the tree 50 60 70 80 90 100 120 Delete the node 90 After removing the above nodes, the In-order will be as follows: 50 60 70 80 100 120