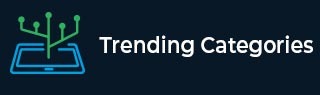
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Nuts and Bolt Problem
A list of different nuts and another list of bolts are given. Our task is to find the correct match of nuts and bolts from the given list, and assign that nut with the Bolt, when it is matched.
This problem is solved by the quick-sort technique. By taking the last element of the bolt as a pivot, rearrange the nuts list and get the final position of the nut whose bolt is the pivot element. After partitioning the nuts list, we can partition the bolts list using the selected nut. The same tasks are performed for left and right sub-lists to get all matches.
Input and Output
Input: The lists of locks and keys. nuts = { ),@,*,^,(,%, !,$,&,#} bolts = { !, (, #, %, ), ^, &, *, $, @ } Output: After matching nuts and bolts: Nuts: ! # $ % & ( ) * @ ^ Bolts: ! # $ % & ( ) * @ ^
Algorithm
partition(array, low, high, pivot)
Input: One array, the low and high index, the pivot element.
Output: Final location of pivot element.
Begin i := low for j in range low to high, do if array[j] < pivot, then swap array[i] and array[j] increase i by 1 else if array[j] = pivot, then swap array[j] and array[high] decrease j by 1 done swap array[i] and array[high] return i End
nutAndBoltMatch(nuts, bolts, low, high)
Input: The list of nuts, the list of bolts, lower and higher index of the array.
Output: Display which nut is for which bolt.
Begin pivotLoc := partition(nuts, low, high, bolts[high]) partition(bolts, low, high, nuts[pivotLoc]) nutAndBoltMatch(nuts, bolts, low, pivotLoc-1) nutAndBoltMatch(nuts, bolts, pivotLoc + 1, high) End
Example
#include<iostream> using namespace std; void show(char array[], int n) { for(int i = 0; i<n; i++) cout << array[i] << " "; } int partition(char array[], int low, int high, char pivot) { //find location of pivot for quick sort int i = low; for(int j = low; j<high; j++) { if(array[j] <pivot) { //when jth element less than pivot, swap ith and jth element swap(array[i], array[j]); i++; }else if(array[j] == pivot) { //when jth element is same as pivot, swap jth and last element swap(array[j], array[high]); j--; } } swap(array[i], array[high]); return i; //the location of pivot element } void nutAndBoltMatch(char nuts[], char bolts[], int low, int high) { if(low < high) { int pivotLoc = partition(nuts, low, high, bolts[high]); //choose item from bolt to nut partitioning partition(bolts, low, high, nuts[pivotLoc]); //place previous pivot location in bolt also nutAndBoltMatch(nuts, bolts, low, pivotLoc - 1); nutAndBoltMatch(nuts, bolts, pivotLoc+1, high); } } int main() { char nuts[] = {')','@','*','^','(','%','!','$','&','#'}; char bolts[] = {'!','(','#','%',')','^','&','*','$','@'}; int n = 10; nutAndBoltMatch(nuts, bolts, 0, n-1); cout << "After matching nuts and bolts:"<< endl; cout << "Nuts: "; show(nuts, n); cout << endl; cout << "Bolts: "; show(bolts, n); cout << endl; }
Output
After matching nuts and bolts: Nuts: ! # $ % & ( ) * @ ^ Bolts: ! # $ % & ( ) * @ ^