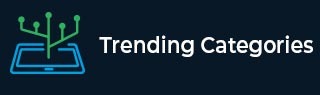
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Linked List Data Structure in Javascript
In this article, we are going to discuss the Linked List data structure in JavaScript.
A linked-list is a sequence of data structures which are connected together via links.
Linked List is a sequence of links which contains items. Each link contains a connection to another link. It is one of the most used Data structures. There are some terms we'll be using when creating linked lists.
Node − This represents each element in the linked list. It consists of 2 parts, data and next. Data contains the data we intend to store, while next contains the reference to the next element in the list.
Link − Each next reference is a link.
Head − The reference to the first element is called ahead.
Example
The following example demonstrates the linked list data structure in JavaScript. We will first create a linked list, then we perform various operations like insertion, deletion, display and check the status of the linked list.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Linked List Data Structure</title> </head> <body> <script type="text/javascript"> class Node { constructor(data) { this.element = data; this.next = null; } } class LinkedList { constructor() { this.head = null; this.size = 0; } add(element) { let node = new Node(element); let temp; if (this.head == null) this.head = node; else { temp = this.head; while (temp.next) { temp = temp.next; } temp.next = node; } this.size++; } insertAt(element, index) { if (index < 0 || index > this.size) return document.write("Enter a valid index."); else { let node = new Node(element); let curr, prev; curr = this.head; if (index == 0) { node.next = this.head; this.head = node; } else { curr = this.head; let it = 0; while (it < index) { it++; prev = curr; curr = curr.next; } node.next = curr; prev.next = node; } this.size++; } } removeFrom(index) { if (index < 0 || index >= this.size) return document.write("Enter a valid index"); else { let curr, prev, it = 0; curr = this.head; prev = curr; if (index === 0) { this.head = curr.next; } else { while (it < index) { it++; prev = curr; curr = curr.next; } prev.next = curr.next; } this.size--; return curr.element; } } removeElement(element) { let curr = this.head; let prev = null; while (curr != null) { if (curr.element === element) { if (prev == null) { this.head = curr.next; } else { prev.next = curr.next; } this.size--; return curr.element; } prev = curr; curr = curr.next; } return -1; } indexOf(element) { let count = 0; let temp = this.head; while (temp != null) { if (temp.element === element) { document.write("</br>The Element is found at the index : "); return count; } count++; temp = temp.next; } return -1; } isEmpty() { return this.size == 0; } size_of_list() { document.write("</br>The size of the Linked List is : " + this.size); } displayList() { let curr = this.head; let str = ""; while (curr) { str += curr.element + " "; curr = curr.next; } document.write("The Elements in the Linked List are: " + str); } } let ll = new LinkedList(); ll.add(10); ll.add(20); ll.add(30); ll.add(40); ll.add(50); ll.displayList(); ll.size_of_list(); document.write(ll.indexOf(40)); </script> </body> </html>