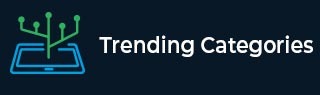
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java program to find the LCM of two numbers
L.C.M. or Least Common Multiple of two values is the smallest positive value which the multiple of both values.
For example multiples of 3 and 4 are:
3 → 3, 6, 9, 12, 15 ... 4 → 4, 8, 12, 16, 20 ...
The smallest multiple of both is 12, hence the LCM of 3 and 4 is 12.
Algorithm
- Initialize A and B with positive integers.
- Store maximum of A & B to the max.
- Check if max is divisible by A and B.
- If divisible, Display max as LCM.
- If not divisible then step increase max, go to step 3.
Example
public class LCMOfTwoNumbers { public static void main(String args[]){ int a, b, max, step, lcm = 0; Scanner sc = new Scanner(System.in); System.out.println("Enter first number ::"); a = sc.nextInt(); System.out.println("Enter second number ::"); b = sc.nextInt(); if(a > b){ max = step = a; } else{ max = step = b; } while(a!= 0) { if(max % a == 0 && max % b == 0) { lcm = max; break; } max += step; } System.out.println("LCM of given numbers is :: "+lcm); } }
Output
Enter first number :: 6 Enter second number :: 10 LCM of given numbers is :: 30
Advertisements