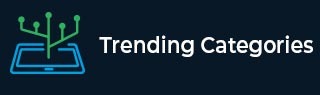
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How would you make a comma-separated string from a list in Python?
In Python, a list is an ordered sequence that can hold several object types such as integer, character, or float. In other programming languages, a list is equivalent to an array. Square brackets [] are used to denote it, and a comma (,) is used to divide two items in the list.
In this article, we will show you how to get a comma-separated string from a list using python. −
Using join() method
Using join() & map() Functions
Using join() & List comprehension
Assume we have taken a list containing some random elements. We will return the string separated by commas from the given list.
Method 1: Using join() method
Algorithm (Steps)
Following are the Algorithm/steps to be followed to perform the desired task −
Create a list and add some dummy strings to it.
Get the comma-separated string from the list by passing the list as an argument to the join() function (join() is a string function in Python that is used to join elements of a sequence that are separated by a string separator. This function connects sequence elements to form a string) and create a variable to store it.
Here we pass delimiter as ',' to separate the strings with comma(',')
Print the result of a comma-separated string.
Example
The following program makes a comma-separated string from a list using the join() function −
# input list lst = ['hello', 'hi', 'TutorialsPoint', 'python'] # getting the comma-separated string from the list resultString = ','.join(lst) # printing the result comma-separated string print('The result string after joining the list with comma separated: ') print(resultString)
Output
On executing, the above program will generate the following output −
The result string after joining the list with comma separated: hello,hi,TutorialsPoint,python
Method 2: Using join() & map() Functions
The map() function accepts iterable objects like lists, tuples, strings, and so on. Following that, the map() function maps the items of the iterable using the specified function.
map(function, iterable)
Consider the following list,
list = ['hello', 'hi', 'TutorialsPoint', 'python', 1, 45, 2.45]
which comprises strings, integers, and floating point numbers. When we use the join() function, we get the following type error.
TypeError: sequence item 4: expected str instance, int found
Let's take a look at how to solve this problem.
Algorithm (Steps)
Following are the Algorithm/steps to be followed to perform the desired task −
Create a variable to store the input list.
Get the comma-separated string from the list by passing the str function, list as arguments to the map() function. It maps each element of the input list to the str function given and returns the resultant list of items. The join() function (It is a string function in Python that is used to join elements of a sequence that are separated by a string separator. This function connects sequence elements to form a string) and then converts the result to a string separated by commas.
Print the result comma-separated string
Example
The following program makes a comma-separated string from a list using using the for loop (Naïve method) −
# input list lst = ['hello', 'hi', 'TutorialsPoint', 'python', 1, 45, 2.45] # getting the comma-separated string from the list resultString = ','.join(map(str, lst)) # printing the result comma-separated string print('The result string after joining the list with comma separated: ') print(resultString)
Output
On executing, the above program will generate the following output −
The result string after joining the list with comma separated: hello,hi,TutorialsPoint,python,1,45,2.45
We took an example list with some random string, integer, and floating point values. Then, using the map() method, we converted each element of the list to a string and used the join() function to get the result.
Method 3: Using join() & List comprehension
Algorithm (Steps)
Following are the Algorithm/steps to be followed to perform the desired task −
Create a variable to store the input list.
Get the comma-separated string from the list using for loop in a list comprehension by converting each element of the list to a string using str() function (converts the provided value into a string. The join() function (It is a string function in Python that is used to join elements of a sequence that are separated by a string separator. This function connects sequence elements to form a string) and then converts the result to a string.
Print the result comma-separated string
Example
The following program makes a comma-separated string from a list using join() & List comprehension -
# input list lst = ['hello', 'hi', 'TutorialsPoint', 'python', 1, 45, 2.45,True] # getting the comma-separated string from the list resultString = ",".join([str(item) for item in lst if item]) # printing the result comma-separated string print('The result string after joining the list with comma separated: ') print(resultString)
Output
On executing, the above program will generate the following output −
The result string after joining the list with comma separated: hello,hi,TutorialsPoint,python,1,45,2.45,True
We took an example list with some random string, integer, floating point, or boolean values. Then, using the list comprehension with for loop to convert each element of the list to a string and used the join() function to get the result.
Conclusion
We covered how to convert a list of strings to a comma-separated string using three different ways in this tutorial. We also learned how to utilize list comprehension to precisely apply any operation on list items. Later on we learned how to use the str() function to convert each item in the heterogeneous list to a string and how to use the map() method to apply a certain function to a list, such as str() in this article to convert each item of the list to a string.